mirror of
https://github.com/RetroShare/RetroShare.git
synced 2025-07-01 09:36:46 -04:00
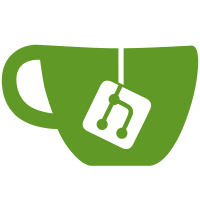
git-svn-id: http://svn.code.sf.net/p/retroshare/code/trunk@6928 b45a01b8-16f6-495d-af2f-9b41ad6348cc
137 lines
3.5 KiB
C++
137 lines
3.5 KiB
C++
#include <fstream>
|
|
#include <string.h>
|
|
|
|
#include <util/utest.h>
|
|
#include <util/argstream.h>
|
|
//#include <pqi/cleanupxpgp.h>
|
|
#include <retroshare/rspeers.h>
|
|
#include <pgp/rscertificate.h>
|
|
#include <pgp/pgphandler.h>
|
|
|
|
INITTEST() ;
|
|
|
|
static std::string pgp_pwd_cb(void * /*hook*/, const char *uid_hint, const char * /*passphrase_info*/, int prev_was_bad)
|
|
{
|
|
#define GPG_DEBUG2
|
|
#ifdef GPG_DEBUG2
|
|
fprintf(stderr, "pgp_pwd_callback() called.\n");
|
|
#endif
|
|
std::string password;
|
|
|
|
std::cerr << "SHould not be called!" << std::endl;
|
|
return password ;
|
|
}
|
|
|
|
int main(int argc,char *argv[])
|
|
{
|
|
try
|
|
{
|
|
argstream as(argc,argv) ;
|
|
std::string keyfile ;
|
|
bool clean_cert = false ;
|
|
|
|
as >> parameter('i',"input",keyfile,"input certificate file (old or new formats)",true)
|
|
>> option('c',"clean",clean_cert,"clean cert before parsing")
|
|
>> help() ;
|
|
|
|
as.defaultErrorHandling() ;
|
|
|
|
FILE *f = fopen(keyfile.c_str(),"rb") ;
|
|
|
|
CHECK(f != NULL) ;
|
|
|
|
std::string res ;
|
|
char c ;
|
|
|
|
while( (c = fgetc(f)) != EOF)
|
|
res += c ;
|
|
|
|
fclose(f) ;
|
|
|
|
std::cerr << "Read this string from the file:" << std::endl;
|
|
std::cerr << "==========================================" << std::endl;
|
|
std::cerr << res << std::endl;
|
|
std::cerr << "==========================================" << std::endl;
|
|
|
|
if(clean_cert)
|
|
{
|
|
std::string res2 ;
|
|
int err ;
|
|
|
|
//res2 = cleanUpCertificate(res,err) ;
|
|
|
|
//if(res2 == "")
|
|
// std::cerr << "Error while cleaning: " << err << std::endl;
|
|
//else
|
|
// res = res2 ;
|
|
|
|
std::cerr << "Certificate after cleaning:" << std::endl;
|
|
std::cerr << "==========================================" << std::endl;
|
|
std::cerr << res << std::endl;
|
|
std::cerr << "==========================================" << std::endl;
|
|
}
|
|
std::cerr << "Parsing..." << std::endl;
|
|
|
|
RsCertificate cert(res) ;
|
|
|
|
std::cerr << "Output from certificate:" << std::endl;
|
|
|
|
std::cerr << cert.toStdString_oldFormat() << std::endl ;
|
|
|
|
std::cerr << "Output from certificate (new format):" << std::endl;
|
|
std::cerr << cert.toStdString() << std::endl ;
|
|
|
|
std::string key_id ;
|
|
std::string name ;
|
|
std::list<std::string> signers ;
|
|
|
|
PGPHandler::setPassphraseCallback(pgp_pwd_cb) ;
|
|
PGPHandler handler("toto1","toto2","toto3","toto4") ;
|
|
handler.getGPGDetailsFromBinaryBlock(cert.pgp_key(),cert.pgp_key_size(),key_id,name,signers) ;
|
|
|
|
CHECK(key_id == "660FB771727CE286") ;
|
|
CHECK(name == "Cyril-test (generated by Retroshare) <cyril.soler@imag.fr>") ;
|
|
|
|
std::cerr << "Details loaded from certificate:" << std::endl;
|
|
std::cerr << "PGP id\t: " << key_id << std::endl;
|
|
std::cerr << "Key name\t: " << name << std::endl;
|
|
std::cerr << "Signers\t:" << std::endl;
|
|
|
|
bool found = false ;
|
|
|
|
for(std::list<std::string>::const_iterator it(signers.begin());it!=signers.end();++it)
|
|
{
|
|
std::cerr << " " << *it << std::endl;
|
|
|
|
if(*it == key_id)
|
|
found = true ;
|
|
}
|
|
CHECK(found) ;
|
|
|
|
// try to load the certificate.
|
|
|
|
std::cerr << "Checking that the certificate is imported correctly" << std::endl;
|
|
|
|
std::string error_string ;
|
|
PGPIdType found_id ;
|
|
|
|
bool result = handler.LoadCertificateFromString(res,found_id,error_string) ;
|
|
|
|
if(!result)
|
|
std::cerr << "Certificate error: " << error_string << std::endl;
|
|
else
|
|
std::cerr << "Certificate loaded correctly. Id = " << found_id.toStdString() << std::endl;
|
|
|
|
CHECK(result) ;
|
|
|
|
FINALREPORT("Test certificate parsing") ;
|
|
|
|
return TESTRESULT();
|
|
}
|
|
catch(std::exception& e)
|
|
{
|
|
std::cerr << "Exception never handled: " << e.what() << std::endl;
|
|
return 1 ;
|
|
}
|
|
}
|
|
|