mirror of
https://github.com/RetroShare/RetroShare.git
synced 2025-07-08 15:29:35 -04:00
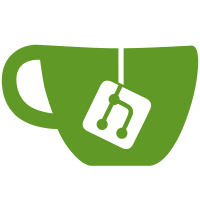
- can add album (with thumbnail!) - add photo (with photo!) - can subscribe, but not added to ui yet need to try some dummy msgs and bring up gxs_net git-svn-id: http://svn.code.sf.net/p/retroshare/code/branches/v0.5-gxs-b1@5549 b45a01b8-16f6-495d-af2f-9b41ad6348cc
155 lines
4 KiB
C++
155 lines
4 KiB
C++
#include <QMouseEvent>
|
|
#include <QBuffer>
|
|
#include <iostream>
|
|
#include <QLabel>
|
|
|
|
#include "PhotoItem.h"
|
|
#include "ui_PhotoItem.h"
|
|
|
|
PhotoItem::PhotoItem(PhotoShareItemHolder *holder, const RsPhotoPhoto &photo, QWidget *parent):
|
|
QWidget(parent),
|
|
ui(new Ui::PhotoItem), mHolder(holder), mPhotoDetails(photo)
|
|
{
|
|
|
|
ui->setupUi(this);
|
|
setSelected(false);
|
|
|
|
ui->lineEdit_PhotoGrapher->setEnabled(false);
|
|
ui->lineEdit_Title->setEnabled(false);
|
|
|
|
ui->editLayOut->removeWidget(ui->lineEdit_Title);
|
|
ui->editLayOut->removeWidget(ui->lineEdit_PhotoGrapher);
|
|
|
|
ui->lineEdit_Title->setVisible(false);
|
|
ui->lineEdit_PhotoGrapher->setVisible(false);
|
|
|
|
setUp();
|
|
}
|
|
|
|
PhotoItem::PhotoItem(PhotoShareItemHolder *holder, const QString& path, QWidget *parent) :
|
|
QWidget(parent),
|
|
ui(new Ui::PhotoItem), mHolder(holder)
|
|
{
|
|
ui->setupUi(this);
|
|
|
|
int width = 120;
|
|
int height = 120;
|
|
|
|
QPixmap qtn = QPixmap(path).scaled(width, height, Qt::KeepAspectRatio, Qt::SmoothTransformation);
|
|
ui->label_Thumbnail->setPixmap(qtn);
|
|
mThumbNail = qtn;
|
|
setSelected(false);
|
|
|
|
getPhotoThumbnail(mPhotoDetails.mThumbnail);
|
|
|
|
connect(ui->lineEdit_Title, SIGNAL(editingFinished()), this, SLOT(setTitle()));
|
|
connect(ui->lineEdit_PhotoGrapher, SIGNAL(editingFinished()), this, SLOT(setPhotoGrapher()));
|
|
|
|
}
|
|
|
|
void PhotoItem::setSelected(bool selected)
|
|
{
|
|
mSelected = selected;
|
|
if (mSelected)
|
|
{
|
|
ui->photoFrame->setStyleSheet("QFrame#photoFrame{border: 2px solid #9562B8;\nbackground: qlineargradient(x1: 0, y1: 0, x2: 0, y2: 1, stop: 0 #55EE55, stop: 1 #CCCCCC);\nborder-radius: 10px}");
|
|
}
|
|
else
|
|
{
|
|
ui->photoFrame->setStyleSheet("QFrame#photoFrame{border: 2px solid #CCCCCC;\nbackground: qlineargradient(x1: 0, y1: 0, x2: 0, y2: 1, stop: 0 #EEEEEE, stop: 1 #CCCCCC);\nborder-radius: 10px}");
|
|
}
|
|
update();
|
|
}
|
|
|
|
bool PhotoItem::getPhotoThumbnail(RsPhotoThumbnail &nail)
|
|
{
|
|
const QPixmap *tmppix = &mThumbNail;
|
|
|
|
QByteArray ba;
|
|
QBuffer buffer(&ba);
|
|
|
|
if(!tmppix->isNull())
|
|
{
|
|
// send chan image
|
|
|
|
buffer.open(QIODevice::WriteOnly);
|
|
tmppix->save(&buffer, "PNG"); // writes image into ba in PNG format
|
|
|
|
RsPhotoThumbnail tmpnail;
|
|
tmpnail.data = (uint8_t *) ba.data();
|
|
tmpnail.size = ba.size();
|
|
|
|
nail.copyFrom(tmpnail);
|
|
|
|
return true;
|
|
}
|
|
|
|
nail.data = NULL;
|
|
nail.size = 0;
|
|
return false;
|
|
}
|
|
|
|
|
|
|
|
void PhotoItem::setTitle(){
|
|
|
|
mPhotoDetails.mMeta.mMsgName = ui->lineEdit_Title->text().toStdString();
|
|
}
|
|
|
|
void PhotoItem::setPhotoGrapher()
|
|
{
|
|
mPhotoDetails.mPhotographer = ui->lineEdit_PhotoGrapher->text().toStdString();
|
|
}
|
|
|
|
const RsPhotoPhoto& PhotoItem::getPhotoDetails()
|
|
{
|
|
return mPhotoDetails;
|
|
}
|
|
|
|
PhotoItem::~PhotoItem()
|
|
{
|
|
delete ui;
|
|
}
|
|
|
|
void PhotoItem::setUp()
|
|
{
|
|
|
|
mTitleLabel = new QLabel();
|
|
mPhotoGrapherLabel = new QLabel();
|
|
|
|
mTitleLabel->setText(QString::fromStdString(mPhotoDetails.mMeta.mMsgName));
|
|
mPhotoGrapherLabel->setText(QString::fromStdString(mPhotoDetails.mPhotographer));
|
|
|
|
|
|
ui->editLayOut->addWidget(mPhotoGrapherLabel);
|
|
ui->editLayOut->addWidget(mTitleLabel);
|
|
|
|
updateImage(mPhotoDetails.mThumbnail);
|
|
}
|
|
|
|
void PhotoItem::updateImage(const RsPhotoThumbnail &thumbnail)
|
|
{
|
|
if (thumbnail.data != NULL)
|
|
{
|
|
QPixmap qtn;
|
|
qtn.loadFromData(thumbnail.data, thumbnail.size, thumbnail.type.c_str());
|
|
ui->label_Thumbnail->setPixmap(qtn);
|
|
mThumbNail = qtn;
|
|
}
|
|
}
|
|
|
|
void PhotoItem::mousePressEvent(QMouseEvent *event)
|
|
{
|
|
|
|
QPoint pos = event->pos();
|
|
|
|
std::cerr << "PhotoItem::mousePressEvent(" << pos.x() << ", " << pos.y() << ")";
|
|
std::cerr << std::endl;
|
|
|
|
if(mHolder)
|
|
mHolder->notifySelection(this);
|
|
else
|
|
setSelected(true);
|
|
|
|
QWidget::mousePressEvent(event);
|
|
}
|