mirror of
https://github.com/RetroShare/RetroShare.git
synced 2024-10-01 02:35:48 -04:00
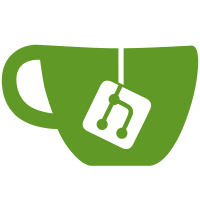
service control. Crypto is not done yet => distant chat is unencrypted. - changed GUI for distant chat. Removed invitation system. - added menu item to distant chat GXS ids from IdentityItem (only entry point for now). - fixed bug in chat lobbies causing re-connexion of lobbies not to happen everytime (bug reported bu Lain) git-svn-id: http://svn.code.sf.net/p/retroshare/code/trunk@7378 b45a01b8-16f6-495d-af2f-9b41ad6348cc
83 lines
2.0 KiB
C++
83 lines
2.0 KiB
C++
#pragma once
|
|
|
|
#include <map>
|
|
#include <QGraphicsView>
|
|
#include <stdint.h>
|
|
#include <retroshare/rstypes.h>
|
|
|
|
#include "util/TokenQueue.h"
|
|
|
|
#include "gui/gxs/RsGxsUpdateBroadcastPage.h"
|
|
|
|
class UIStateHelper;
|
|
class IdentityItem ;
|
|
class CircleItem ;
|
|
|
|
class GroupListView : public QGraphicsView, public TokenResponse
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
static const uint32_t GLVIEW_IDLIST ;
|
|
static const uint32_t GLVIEW_IDDETAILS ;
|
|
static const uint32_t GLVIEW_CIRCLES ;
|
|
static const uint32_t GLVIEW_REFRESH ;
|
|
|
|
GroupListView(QWidget * = NULL);
|
|
|
|
void clearGraph() ;
|
|
|
|
// Derives from RsGxsUpdateBroadcastPage
|
|
virtual void updateDisplay(bool) ;
|
|
|
|
virtual void itemMoved();
|
|
|
|
void setEdgeLength(uint32_t l) ;
|
|
uint32_t edgeLength() const { return _edge_length ; }
|
|
|
|
void loadRequest(const TokenQueue * /*queue*/, const TokenRequest &req) ;
|
|
|
|
void requestIdList() ;
|
|
void requestCirclesList() ;
|
|
|
|
void insertIdList(uint32_t token) ;
|
|
void insertCircles(uint32_t token) ;
|
|
|
|
protected slots:
|
|
void forceRedraw() ;
|
|
protected:
|
|
void timerEvent(QTimerEvent *event);
|
|
void wheelEvent(QWheelEvent *event);
|
|
void drawBackground(QPainter *painter, const QRectF &rect);
|
|
|
|
void scaleView(qreal scaleFactor);
|
|
|
|
virtual void keyPressEvent(QKeyEvent *event);
|
|
virtual void resizeEvent(QResizeEvent *e) ;
|
|
|
|
static QImage makeDefaultIcon(const RsGxsId& id) ;
|
|
|
|
private:
|
|
int timerId;
|
|
//Node *centerNode;
|
|
bool mDeterminedBB ;
|
|
bool mIsFrozen;
|
|
|
|
//std::vector<Node *> _groups ;
|
|
//std::map<std::pair<NodeId,NodeId>,Edge *> _edges ;
|
|
//std::map<std::string,QPointF> _node_cached_positions ;
|
|
|
|
uint32_t _edge_length ;
|
|
float _friction_factor ;
|
|
//NodeId _current_node ;
|
|
|
|
TokenQueue *mIdentityQueue;
|
|
TokenQueue *mCirclesQueue;
|
|
|
|
UIStateHelper *mStateHelper;
|
|
|
|
std::map<RsGxsGroupId,IdentityItem *> _identity_items ;
|
|
std::map<RsGxsGroupId,CircleItem *> _circles_items ;
|
|
};
|
|
|