mirror of
https://github.com/RetroShare/RetroShare.git
synced 2024-10-01 02:35:48 -04:00
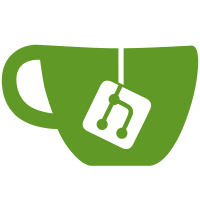
- Added RsTvlUnit: public RsTlvItem, which handles some of the infrstructure. - made TlvSize() SetTlv() and print() const. - split rstlvtypes into its bits and cleaned up header files. - added templated RsTlvList, imilar to cyrils id one. - removed GXS_GEN_TESTNET - cleanedup rsserviceids. git-svn-id: http://svn.code.sf.net/p/retroshare/code/trunk@7215 b45a01b8-16f6-495d-af2f-9b41ad6348cc
377 lines
8.5 KiB
C++
377 lines
8.5 KiB
C++
|
|
/*
|
|
* libretroshare/src/serialiser: rstlvstring.cc
|
|
*
|
|
* RetroShare Serialiser.
|
|
*
|
|
* Copyright 2007-2008 by Robert Fernie, Chris Parker
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Library General Public
|
|
* License Version 2 as published by the Free Software Foundation.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Library General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Library General Public
|
|
* License along with this library; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
|
|
* USA.
|
|
*
|
|
* Please report all bugs and problems to "retroshare@lunamutt.com".
|
|
*
|
|
*/
|
|
|
|
#include "serialiser/rstlvstring.h"
|
|
|
|
#include "serialiser/rstlvbase.h"
|
|
#include "util/rsprint.h"
|
|
#include <iostream>
|
|
|
|
#define TLV_DEBUG 1
|
|
|
|
/************************************* Peer Id Set ************************************/
|
|
|
|
|
|
RsTlvStringSet::RsTlvStringSet(uint16_t type) :mType(type)
|
|
{
|
|
}
|
|
|
|
|
|
bool RsTlvStringSet::SetTlv(void *data, uint32_t size, uint32_t *offset) const
|
|
{
|
|
/* must check sizes */
|
|
uint32_t tlvsize = TlvSize();
|
|
uint32_t tlvend = *offset + tlvsize;
|
|
|
|
if (size < tlvend)
|
|
return false; /* not enough space */
|
|
|
|
bool ok = true;
|
|
|
|
|
|
/* start at data[offset] */
|
|
ok &= SetTlvBase(data, tlvend, offset, mType , tlvsize);
|
|
|
|
/* determine the total size of ids strings in list */
|
|
|
|
std::list<std::string>::const_iterator it;
|
|
|
|
for(it = ids.begin(); it != ids.end() ; ++it)
|
|
{
|
|
if (it->length() > 0)
|
|
ok &= SetTlvString(data, tlvend, offset, TLV_TYPE_STR_GENID, *it);
|
|
}
|
|
|
|
return ok;
|
|
|
|
}
|
|
|
|
|
|
void RsTlvStringSet::TlvClear()
|
|
{
|
|
ids.clear();
|
|
|
|
}
|
|
|
|
uint32_t RsTlvStringSet::TlvSize() const
|
|
{
|
|
|
|
uint32_t s = TLV_HEADER_SIZE; /* header */
|
|
|
|
/* determine the total size of ids strings in list */
|
|
|
|
std::list<std::string>::const_iterator it;
|
|
|
|
for(it = ids.begin(); it != ids.end() ; ++it)
|
|
{
|
|
if (it->length() > 0)
|
|
s += GetTlvStringSize(*it);
|
|
}
|
|
|
|
return s;
|
|
}
|
|
|
|
|
|
bool RsTlvStringSet::GetTlv(void *data, uint32_t size, uint32_t *offset)
|
|
{
|
|
if (size < *offset + TLV_HEADER_SIZE)
|
|
return false;
|
|
|
|
uint16_t tlvtype = GetTlvType( &(((uint8_t *) data)[*offset]) );
|
|
uint32_t tlvsize = GetTlvSize( &(((uint8_t *) data)[*offset]) );
|
|
uint32_t tlvend = *offset + tlvsize;
|
|
|
|
|
|
|
|
if (size < tlvend) /* check size */
|
|
return false; /* not enough space */
|
|
|
|
if (tlvtype != mType) /* check type */
|
|
return false;
|
|
|
|
bool ok = true;
|
|
|
|
/* ready to load */
|
|
TlvClear();
|
|
|
|
/* skip the header */
|
|
(*offset) += TLV_HEADER_SIZE;
|
|
|
|
|
|
|
|
/* while there is TLV */
|
|
while((*offset) + 2 < tlvend)
|
|
{
|
|
/* get the next type */
|
|
uint16_t tlvsubtype = GetTlvType( &(((uint8_t *) data)[*offset]) );
|
|
|
|
if (tlvsubtype == TLV_TYPE_STR_GENID)
|
|
{
|
|
std::string newIds;
|
|
ok &= GetTlvString(data, tlvend, offset, TLV_TYPE_STR_GENID, newIds);
|
|
if(ok)
|
|
{
|
|
ids.push_back(newIds);
|
|
|
|
}
|
|
}
|
|
else
|
|
{
|
|
/* Step past unknown TLV TYPE */
|
|
ok &= SkipUnknownTlv(data, tlvend, offset);
|
|
}
|
|
|
|
if (!ok)
|
|
{
|
|
break;
|
|
}
|
|
}
|
|
|
|
/***************************************************************************
|
|
* NB: extra components could be added (for future expansion of the type).
|
|
* or be present (if this code is reading an extended version).
|
|
*
|
|
* We must chew up the extra characters to conform with TLV specifications
|
|
***************************************************************************/
|
|
if (*offset != tlvend)
|
|
{
|
|
#ifdef TLV_DEBUG
|
|
std::cerr << "RsTlvPeerIdSet::GetTlv() Warning extra bytes at end of item";
|
|
std::cerr << std::endl;
|
|
#endif
|
|
*offset = tlvend;
|
|
}
|
|
|
|
return ok;
|
|
}
|
|
|
|
/// print to screen RsTlvStringSet contents
|
|
std::ostream &RsTlvStringSet::print(std::ostream &out, uint16_t indent) const
|
|
{
|
|
printBase(out, "RsTlvStringSet", indent);
|
|
uint16_t int_Indent = indent + 2;
|
|
|
|
printIndent(out, int_Indent);
|
|
out << "type:" << mType;
|
|
out << std::endl;
|
|
|
|
std::list<std::string>::const_iterator it;
|
|
for(it = ids.begin(); it != ids.end() ; ++it)
|
|
{
|
|
printIndent(out, int_Indent);
|
|
out << "id:" << *it;
|
|
out << std::endl;
|
|
}
|
|
|
|
printEnd(out, "RsTlvStringSet", indent);
|
|
return out;
|
|
|
|
}
|
|
|
|
/// print to screen RsTlvStringSet contents
|
|
std::ostream &RsTlvStringSet::printHex(std::ostream &out, uint16_t indent) const
|
|
{
|
|
printBase(out, "RsTlvStringSet", indent);
|
|
uint16_t int_Indent = indent + 2;
|
|
|
|
printIndent(out, int_Indent);
|
|
out << "type:" << mType;
|
|
out << std::endl;
|
|
|
|
std::list<std::string>::const_iterator it;
|
|
for(it = ids.begin(); it != ids.end() ; ++it)
|
|
{
|
|
printIndent(out, int_Indent);
|
|
out << "id: 0x" << RsUtil::BinToHex(*it);
|
|
out << std::endl;
|
|
}
|
|
|
|
printEnd(out, "RsTlvStringSet", indent);
|
|
return out;
|
|
|
|
}
|
|
|
|
|
|
/************************************* String Set Ref ************************************/
|
|
/* This is exactly the same as StringSet, but it uses an alternative list.
|
|
*/
|
|
RsTlvStringSetRef::RsTlvStringSetRef(uint16_t type, std::list<std::string> &refids)
|
|
:mType(type), ids(refids)
|
|
{
|
|
}
|
|
|
|
void RsTlvStringSetRef::TlvClear()
|
|
{
|
|
ids.clear();
|
|
|
|
}
|
|
|
|
uint32_t RsTlvStringSetRef::TlvSize() const
|
|
{
|
|
|
|
uint32_t s = TLV_HEADER_SIZE; /* header */
|
|
|
|
/* determine the total size of ids strings in list */
|
|
|
|
std::list<std::string>::const_iterator it;
|
|
|
|
for(it = ids.begin(); it != ids.end() ; ++it)
|
|
{
|
|
if (it->length() > 0)
|
|
s += GetTlvStringSize(*it);
|
|
}
|
|
|
|
return s;
|
|
}
|
|
|
|
|
|
bool RsTlvStringSetRef::SetTlv(void *data, uint32_t size, uint32_t *offset) const
|
|
{
|
|
/* must check sizes */
|
|
uint32_t tlvsize = TlvSize();
|
|
uint32_t tlvend = *offset + tlvsize;
|
|
|
|
if (size < tlvend)
|
|
return false; /* not enough space */
|
|
|
|
bool ok = true;
|
|
|
|
|
|
/* start at data[offset] */
|
|
ok &= SetTlvBase(data, tlvend, offset, mType , tlvsize);
|
|
|
|
/* determine the total size of ids strings in list */
|
|
|
|
std::list<std::string>::const_iterator it;
|
|
|
|
for(it = ids.begin(); it != ids.end() ; ++it)
|
|
{
|
|
if (it->length() > 0)
|
|
ok &= SetTlvString(data, tlvend, offset, TLV_TYPE_STR_GENID, *it);
|
|
}
|
|
|
|
return ok;
|
|
|
|
}
|
|
|
|
|
|
bool RsTlvStringSetRef::GetTlv(void *data, uint32_t size, uint32_t *offset)
|
|
{
|
|
if (size < *offset + TLV_HEADER_SIZE)
|
|
return false;
|
|
|
|
uint16_t tlvtype = GetTlvType( &(((uint8_t *) data)[*offset]) );
|
|
uint32_t tlvsize = GetTlvSize( &(((uint8_t *) data)[*offset]) );
|
|
uint32_t tlvend = *offset + tlvsize;
|
|
|
|
|
|
|
|
if (size < tlvend) /* check size */
|
|
return false; /* not enough space */
|
|
|
|
if (tlvtype != mType) /* check type */
|
|
return false;
|
|
|
|
bool ok = true;
|
|
|
|
/* ready to load */
|
|
TlvClear();
|
|
|
|
/* skip the header */
|
|
(*offset) += TLV_HEADER_SIZE;
|
|
|
|
|
|
|
|
/* while there is TLV */
|
|
while((*offset) + 2 < tlvend)
|
|
{
|
|
/* get the next type */
|
|
uint16_t tlvsubtype = GetTlvType( &(((uint8_t *) data)[*offset]) );
|
|
|
|
if (tlvsubtype == TLV_TYPE_STR_GENID)
|
|
{
|
|
std::string newIds;
|
|
ok &= GetTlvString(data, tlvend, offset, TLV_TYPE_STR_GENID, newIds);
|
|
if(ok)
|
|
{
|
|
ids.push_back(newIds);
|
|
|
|
}
|
|
}
|
|
else
|
|
{
|
|
/* Step past unknown TLV TYPE */
|
|
ok &= SkipUnknownTlv(data, tlvend, offset);
|
|
}
|
|
|
|
if (!ok)
|
|
{
|
|
break;
|
|
}
|
|
}
|
|
|
|
/***************************************************************************
|
|
* NB: extra components could be added (for future expansion of the type).
|
|
* or be present (if this code is reading an extended version).
|
|
*
|
|
* We must chew up the extra characters to conform with TLV specifications
|
|
***************************************************************************/
|
|
if (*offset != tlvend)
|
|
{
|
|
#ifdef TLV_DEBUG
|
|
std::cerr << "RsTlvPeerIdSetRef::GetTlv() Warning extra bytes at end of item";
|
|
std::cerr << std::endl;
|
|
#endif
|
|
*offset = tlvend;
|
|
}
|
|
|
|
return ok;
|
|
}
|
|
|
|
/// print to screen RsTlvStringSet contents
|
|
std::ostream &RsTlvStringSetRef::print(std::ostream &out, uint16_t indent) const
|
|
{
|
|
printBase(out, "RsTlvStringSetRef", indent);
|
|
uint16_t int_Indent = indent + 2;
|
|
|
|
printIndent(out, int_Indent);
|
|
out << "type:" << mType;
|
|
out << std::endl;
|
|
|
|
std::list<std::string>::const_iterator it;
|
|
for(it = ids.begin(); it != ids.end() ; ++it)
|
|
{
|
|
printIndent(out, int_Indent);
|
|
out << "id:" << *it;
|
|
out << std::endl;
|
|
}
|
|
|
|
printEnd(out, "RsTlvStringSetRef", indent);
|
|
return out;
|
|
|
|
}
|
|
|