mirror of
https://github.com/RetroShare/RetroShare.git
synced 2025-01-21 12:51:09 -05:00
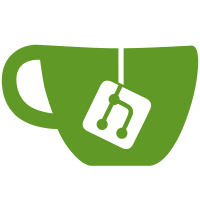
Bugfixes and a couple of small improvements to the DHT code. * fixed up buggy overloading for the udplayer (for testing). * added processing multiple (5) remote processes per tick. - (1 wasn't enough in testing, and led to dropped peers) * removed unused variables from query data structure. * #defined out debugging in bdSpace, removed old functions. * More agressive attempts to find proxies for connections. * made "final query attempt" use exact peer address rather than midid. - mid id meant that the target wasn't always returned. * tweaked bdconnection debugging. * increase CONNECTION_MAX_TIMEOUT from 30 => 45. wasn't enough! * Limited bdQuery::QueryIdlePeriod to 15min (was unlimited). * added bdQuery::PotentialPeer cleanup functions - for more robust reporting. * fixed bdQuery debugging printouts. * Implemented BITDHT_QFLAGS_UPDATES flag. git-svn-id: http://svn.code.sf.net/p/retroshare/code/trunk@4398 b45a01b8-16f6-495d-af2f-9b41ad6348cc
197 lines
5.7 KiB
C++
197 lines
5.7 KiB
C++
#ifndef BITDHT_MANAGER_H
|
|
#define BITDHT_MANAGER_H
|
|
|
|
/*
|
|
* bitdht/bdmanager.h
|
|
*
|
|
* BitDHT: An Flexible DHT library.
|
|
*
|
|
* Copyright 2010 by Robert Fernie
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Library General Public
|
|
* License Version 3 as published by the Free Software Foundation.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Library General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Library General Public
|
|
* License along with this library; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
|
|
* USA.
|
|
*
|
|
* Please report all bugs and problems to "bitdht@lunamutt.com".
|
|
*
|
|
*/
|
|
|
|
|
|
|
|
/*******
|
|
* Node Manager.
|
|
******/
|
|
|
|
/******************************************
|
|
* 1) Maintains a list of ids to search for.
|
|
* 2) Sets up initial search for own node.
|
|
* 3) Checks on status of queries.
|
|
* 4) Callback on successful searches.
|
|
*
|
|
* This is pretty specific to RS requirements.
|
|
****/
|
|
|
|
#define BITDHT_PS_MASK_ACTIONS (0x000000ff)
|
|
#define BITDHT_PS_MASK_STATE (0x0000ff00)
|
|
|
|
#define BITDHT_PS_ACTION_SEARCHING (0x00000001)
|
|
#define BITDHT_PS_ACTION_WAITING (0x00000002)
|
|
#define BITDHT_PS_ACTION_PINGING (0x00000004)
|
|
|
|
#define BITDHT_PS_STATE_UNKNOWN (0x00000100)
|
|
#define BITDHT_PS_STATE_OFFLINE (0x00000200)
|
|
#define BITDHT_PS_STATE_ONLINE (0x00000400)
|
|
#define BITDHT_PS_STATE_CONNECTED (0x00000800)
|
|
|
|
#include "bitdht/bdiface.h"
|
|
#include "bitdht/bdnode.h"
|
|
#include "util/bdbloom.h"
|
|
|
|
|
|
|
|
class bdQueryPeer
|
|
{
|
|
public:
|
|
bdId mId;
|
|
uint32_t mStatus;
|
|
uint32_t mQFlags;
|
|
//time_t mLastQuery;
|
|
//time_t mLastFound;
|
|
struct sockaddr_in mDhtAddr;
|
|
time_t mCallbackTS; // for UPDATES flag.
|
|
};
|
|
|
|
|
|
#define BITDHT_MGR_STATE_OFF 0
|
|
#define BITDHT_MGR_STATE_STARTUP 1
|
|
#define BITDHT_MGR_STATE_FINDSELF 2
|
|
#define BITDHT_MGR_STATE_ACTIVE 3
|
|
#define BITDHT_MGR_STATE_REFRESH 4
|
|
#define BITDHT_MGR_STATE_QUIET 5
|
|
#define BITDHT_MGR_STATE_FAILED 6
|
|
|
|
#define MAX_STARTUP_TIME 10
|
|
#define MAX_REFRESH_TIME 10
|
|
|
|
#define BITDHT_MGR_QUERY_FAILURE 1
|
|
#define BITDHT_MGR_QUERY_PEER_OFFLINE 2
|
|
#define BITDHT_MGR_QUERY_PEER_UNREACHABLE 3
|
|
#define BITDHT_MGR_QUERY_PEER_ONLINE 4
|
|
|
|
|
|
/*** NB: Nothing in here is protected by mutexes
|
|
* must be done at a higher level!
|
|
***/
|
|
|
|
class bdNodeManager: public bdNode, public BitDhtInterface
|
|
{
|
|
public:
|
|
bdNodeManager(bdNodeId *id, std::string dhtVersion, std::string bootfile, bdDhtFunctions *fns);
|
|
|
|
|
|
void iteration();
|
|
|
|
/***** Functions to Call down to bdNodeManager ****/
|
|
/* Request DHT Peer Lookup */
|
|
/* Request Keyword Lookup */
|
|
virtual void addFindNode(bdNodeId *id, uint32_t mode);
|
|
virtual void removeFindNode(bdNodeId *id);
|
|
virtual void findDhtValue(bdNodeId *id, std::string key, uint32_t mode);
|
|
|
|
/***** Add / Remove Callback Clients *****/
|
|
virtual void addCallback(BitDhtCallback *cb);
|
|
virtual void removeCallback(BitDhtCallback *cb);
|
|
|
|
/***** Get Results Details *****/
|
|
virtual int getDhtPeerAddress(const bdNodeId *id, struct sockaddr_in &from);
|
|
virtual int getDhtValue(const bdNodeId *id, std::string key, std::string &value);
|
|
virtual int getDhtBucket(const int idx, bdBucket &bucket);
|
|
|
|
virtual int getDhtQueries(std::map<bdNodeId, bdQueryStatus> &queries);
|
|
virtual int getDhtQueryStatus(const bdNodeId *id, bdQuerySummary &query);
|
|
|
|
/***** Connection Interface ****/
|
|
virtual void ConnectionRequest(struct sockaddr_in *laddr, bdNodeId *target, uint32_t mode, uint32_t start);
|
|
virtual void ConnectionAuth(bdId *srcId, bdId *proxyId, bdId *destId,
|
|
uint32_t mode, uint32_t loc, uint32_t answer);
|
|
virtual void ConnectionOptions(uint32_t allowedModes, uint32_t flags);
|
|
|
|
|
|
/* stats and Dht state */
|
|
virtual int startDht();
|
|
virtual int stopDht();
|
|
virtual int stateDht(); /* STOPPED, STARTING, ACTIVE, FAILED */
|
|
virtual uint32_t statsNetworkSize();
|
|
virtual uint32_t statsBDVersionSize(); /* same version as us! */
|
|
/******************* Internals *************************/
|
|
|
|
// Overloaded from bdnode for external node callback.
|
|
virtual void addPeer(const bdId *id, uint32_t peerflags);
|
|
// Overloaded from bdnode for external node callback.
|
|
virtual void callbackConnect(bdId *srcId, bdId *proxyId, bdId *destId,
|
|
int mode, int point, int cbtype, int errcode);
|
|
|
|
int isBitDhtPacket(char *data, int size, struct sockaddr_in &from);
|
|
private:
|
|
|
|
|
|
void doNodeCallback(const bdId *id, uint32_t peerflags);
|
|
void doPeerCallback(const bdId *id, uint32_t status);
|
|
void doValueCallback(const bdNodeId *id, std::string key, uint32_t status);
|
|
|
|
int status();
|
|
int checkStatus();
|
|
int checkPingStatus();
|
|
int SearchOutOfDate();
|
|
void startQueries();
|
|
|
|
int QueryRandomLocalNet();
|
|
void SearchForLocalNet();
|
|
|
|
std::map<bdNodeId, bdQueryPeer> mActivePeers;
|
|
std::list<BitDhtCallback *> mCallbacks;
|
|
|
|
uint32_t mMode;
|
|
time_t mModeTS;
|
|
|
|
time_t mStartTS;
|
|
time_t mSearchTS;
|
|
bool mSearchingDone;
|
|
|
|
bdDhtFunctions *mFns;
|
|
|
|
uint32_t mNetworkSize;
|
|
uint32_t mBdNetworkSize;
|
|
|
|
bdBloom mBloomFilter;
|
|
|
|
/* future node functions */
|
|
//addPeerPing(foundId);
|
|
//clearPing(it->first);
|
|
//PingStatus(it->first);
|
|
};
|
|
|
|
class bdDebugCallback: public BitDhtCallback
|
|
{
|
|
public:
|
|
~bdDebugCallback();
|
|
virtual int dhtPeerCallback(const bdId *id, uint32_t status);
|
|
virtual int dhtValueCallback(const bdNodeId *id, std::string key, uint32_t status);
|
|
virtual int dhtConnectCallback(const bdId *srcId, const bdId *proxyId, const bdId *destId,
|
|
uint32_t mode, uint32_t point, uint32_t cbtype, uint32_t errcode);
|
|
|
|
};
|
|
|
|
|
|
#endif
|