mirror of
https://github.com/RetroShare/RetroShare.git
synced 2024-10-01 02:35:48 -04:00
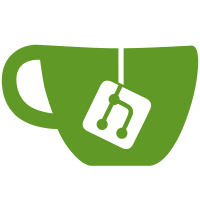
The crash was introduced at 533dbef0c7
This has been particurarly tricky as lot of different parts contributed
in causing unexpected behaviours
When the activity is created onNewIntent is not called and we have to
get the intent data from C++ bu other means, but C++ code is running
in a different thread so there is no guarantee that the intent data is
reacheable yet on starting, so the C++ code has to wait for the intent
data being ready, but paying attention to not cause a deadlock beetween
the two thread (the android ui thread may be waiting for some
operation performed by Qt)
Because of notification intent flags not properly set the activity was
recreated also if it was already on top, this caused a nasty
interaction between android ui thread and qt thread that derived in a
deadlock, to avoid this lot of try/error has been made until the
proper soup of manifest and intent flags has been found
At this point link handling, notification handling, and Activity closing
should work as expected without any deadlock or crash
50 lines
1.5 KiB
C
50 lines
1.5 KiB
C
#pragma once
|
|
/*
|
|
* RetroShare Android Service
|
|
* Copyright (C) 2017 Gioacchino Mazzurco <gio@eigenlab.org>
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU Affero General Public License as
|
|
* published by the Free Software Foundation, either version 3 of the
|
|
* License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU Affero General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Affero General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include <QObject>
|
|
#include <QString>
|
|
#include <QDebug>
|
|
|
|
#ifdef __ANDROID__
|
|
# include <QtAndroid>
|
|
# include <QtAndroidExtras/QAndroidJniObject>
|
|
#endif // __ANDROID__
|
|
|
|
struct NotificationsBridge : QObject
|
|
{
|
|
Q_OBJECT
|
|
|
|
public slots:
|
|
static void notify(const QString& title, const QString& text = "",
|
|
const QString& uri = "")
|
|
{
|
|
qDebug() << __PRETTY_FUNCTION__ << title << text << uri;
|
|
|
|
#ifdef __ANDROID__
|
|
QtAndroid::androidService().callMethod<void>(
|
|
"notify",
|
|
"(Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;)V",
|
|
QAndroidJniObject::fromString(title).object(),
|
|
QAndroidJniObject::fromString(text).object(),
|
|
QAndroidJniObject::fromString(uri).object()
|
|
);
|
|
#endif // __ANDROID__
|
|
}
|
|
};
|