mirror of
https://github.com/RetroShare/RetroShare.git
synced 2024-10-01 02:35:48 -04:00
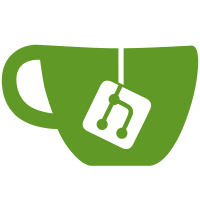
libretroshare/src/retroshare/ All the relevant headers have been modified to reflect that change. This allows installation of libretroshare on a system, headers will be put in $WHEREVER/retroshare/ and we keep the ability to compile against them, be it on the system or in the SVN tree. git-svn-id: http://svn.code.sf.net/p/retroshare/code/trunk@3342 b45a01b8-16f6-495d-af2f-9b41ad6348cc
133 lines
3.4 KiB
C++
Executable File
133 lines
3.4 KiB
C++
Executable File
/****************************************************************
|
|
* RetroShare is distributed under the following license:
|
|
*
|
|
* Copyright (C) 2006 - 2009 RetroShare Team
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor,
|
|
* Boston, MA 02110-1301, USA.
|
|
****************************************************************/
|
|
|
|
#include <QMessageBox>
|
|
#include <QClipboard>
|
|
#include <QFileDialog>
|
|
#include <QTextStream>
|
|
#include <QTextCodec>
|
|
|
|
#include "CryptoPage.h"
|
|
|
|
#include <retroshare/rspeers.h> //for rsPeers variable
|
|
|
|
/** Constructor */
|
|
CryptoPage::CryptoPage(QWidget * parent, Qt::WFlags flags)
|
|
: ConfigPage(parent, flags)
|
|
{
|
|
/* Invoke the Qt Designer generated object setup routine */
|
|
ui.setupUi(this);
|
|
|
|
connect(ui.copykeyButton, SIGNAL(clicked()), this, SLOT(copyPublicKey()));
|
|
connect(ui.saveButton, SIGNAL(clicked()), this, SLOT(fileSaveAs()));
|
|
|
|
|
|
loadPublicKey();
|
|
|
|
|
|
/* Hide platform specific features */
|
|
#ifdef Q_WS_WIN
|
|
|
|
#endif
|
|
}
|
|
|
|
CryptoPage::~CryptoPage()
|
|
{
|
|
}
|
|
|
|
void
|
|
CryptoPage::closeEvent (QCloseEvent * event)
|
|
{
|
|
|
|
QWidget::closeEvent(event);
|
|
}
|
|
|
|
/** Saves the changes on this page */
|
|
bool
|
|
CryptoPage::save(QString &errmsg)
|
|
{
|
|
return true;
|
|
}
|
|
|
|
/** Loads the settings for this page */
|
|
void
|
|
CryptoPage::load()
|
|
{
|
|
|
|
}
|
|
|
|
/** Loads ouer default Puplickey */
|
|
void
|
|
CryptoPage::loadPublicKey()
|
|
{
|
|
QFont font("Courier New",9,50,false) ;
|
|
ui.certtextEdit->setFont(font) ;
|
|
|
|
ui.certtextEdit->setPlainText(QString::fromStdString(rsPeers->GetRetroshareInvite()));
|
|
ui.certtextEdit->setReadOnly(true);
|
|
ui.certtextEdit->setMinimumHeight(200);
|
|
}
|
|
|
|
void
|
|
CryptoPage::copyPublicKey()
|
|
{
|
|
QMessageBox::information(this,
|
|
tr("RetroShare"),
|
|
tr("Your Public Key is copied to Clipboard, paste and send it to your"
|
|
" friend via email or some other way"));
|
|
QClipboard *clipboard = QApplication::clipboard();
|
|
clipboard->setText(ui.certtextEdit->toPlainText());
|
|
|
|
}
|
|
|
|
bool CryptoPage::fileSave()
|
|
{
|
|
if (fileName.isEmpty())
|
|
return fileSaveAs();
|
|
|
|
QFile file(fileName);
|
|
if (!file.open(QFile::WriteOnly))
|
|
return false;
|
|
QTextStream ts(&file);
|
|
ts.setCodec(QTextCodec::codecForName("UTF-8"));
|
|
ts << ui.certtextEdit->document()->toPlainText();
|
|
ui.certtextEdit->document()->setModified(false);
|
|
return true;
|
|
}
|
|
|
|
bool CryptoPage::fileSaveAs()
|
|
{
|
|
QString fn = QFileDialog::getSaveFileName(this, tr("Save as..."),
|
|
QString(), tr("RetroShare Certificate (*.rsc );;All Files (*)"));
|
|
if (fn.isEmpty())
|
|
return false;
|
|
setCurrentFileName(fn);
|
|
return fileSave();
|
|
}
|
|
|
|
void CryptoPage::setCurrentFileName(const QString &fileName)
|
|
{
|
|
this->fileName = fileName;
|
|
ui.certtextEdit->document()->setModified(false);
|
|
|
|
setWindowModified(false);
|
|
}
|