mirror of
https://github.com/RetroShare/RetroShare.git
synced 2024-10-01 02:35:48 -04:00
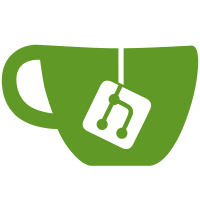
added orig msgid and removed sign from nxsmsg, renamed nxsitems more appropriately added more code to nxs net service removed msg versioning from data service and added extra msgField modified nxsitem test and datastore service appropriately, all pass added serialisation of transactions and added transaction number to nxsitems git-svn-id: http://svn.code.sf.net/p/retroshare/code/branches/v0.5-new_cache_system@5215 b45a01b8-16f6-495d-af2f-9b41ad6348cc
129 lines
3.8 KiB
C++
129 lines
3.8 KiB
C++
#ifndef RSDATASERVICE_H
|
|
#define RSDATASERVICE_H
|
|
|
|
#include "rsgds.h"
|
|
|
|
#include "util/retrodb.h"
|
|
|
|
class RsDataService : public RsGeneralDataService
|
|
{
|
|
public:
|
|
|
|
RsDataService(const std::string& serviceDir, const std::string& dbName, uint16_t serviceType, RsGxsSearchModule* mod = NULL);
|
|
virtual ~RsDataService();
|
|
|
|
/*!
|
|
* Retrieves latest version of msgs for a service
|
|
* @param msgIds ids of messagesto retrieve
|
|
* @param msg result of msg retrieval
|
|
* @param cache whether to store retrieval in memory for faster later retrieval
|
|
* @return error code
|
|
*/
|
|
int retrieveMsgs(const std::string& grpId, std::map<std::string, RsNxsMsg*>& msg, bool cache);
|
|
|
|
/*!
|
|
* Retrieves latest version of groups for a service
|
|
* @param grpId the Id of the groups to retrieve
|
|
* @param grp results of retrieval
|
|
* @param cache whether to store retrieval in mem for faster later retrieval
|
|
* @return error code
|
|
*/
|
|
int retrieveGrps(std::map<std::string, RsNxsGrp*>& grp, bool cache);
|
|
|
|
/*!
|
|
* Retrieves all the versions of a group
|
|
* @param grpId the id of the group to get versions for
|
|
* @param cache whether to store the result in memory
|
|
* @return errCode
|
|
*/
|
|
int retrieveGrpVersions(const std::string &grpId, std::set<RsNxsGrp *> &grp, bool cache);
|
|
|
|
/*!
|
|
* @param grpId the id of the group to retrieve
|
|
* @return NULL if group does not exist or pointer to grp if found
|
|
*/
|
|
RsNxsGrp* retrieveGrpVersion(const RsGxsGrpId& grpId);
|
|
|
|
/*!
|
|
* remove msgs in data store listed in msgIds param
|
|
* @param msgIds ids of messages to be removed
|
|
* @return error code
|
|
*/
|
|
int removeMsgs(const std::list<RsGxsMsgId>& msgIds);
|
|
|
|
/*!
|
|
* remove groups in data store listed in grpIds param
|
|
* @param grpIds ids of groups to be removed
|
|
* @return error code
|
|
*/
|
|
int removeGroups(const std::list<RsGxsGrpId>& grpIds);
|
|
|
|
|
|
/*!
|
|
* allows for more complex queries specific to the service
|
|
* @param search generally stores parameters needed for query
|
|
* @param msgId is set with msg ids which satisfy the gxs search
|
|
* @return error code
|
|
*/
|
|
int searchMsgs(RsGxsSearch* search, std::list<RsGxsSrchResMsgCtx*>& result);
|
|
|
|
/*!
|
|
* allows for more complex queries specific to the associated service
|
|
* @param search generally stores parameters needed for query
|
|
* @param msgId is set with msg ids which satisfy the gxs search
|
|
* @return error code
|
|
*/
|
|
int searchGrps(RsGxsSearch* search, std::list<RsGxsSrchResGrpCtx*>& result);
|
|
|
|
/*!
|
|
* @return the cache size set for this RsGeneralDataService in bytes
|
|
*/
|
|
uint32_t cacheSize() const;
|
|
|
|
/*!
|
|
* @param size size of cache to set in bytes
|
|
*/
|
|
virtual int setCacheSize(uint32_t size);
|
|
|
|
/*!
|
|
* Stores a list signed messages into data store
|
|
* @param msg list of signed messages to store
|
|
* @return error code
|
|
*/
|
|
int storeMessage(std::set<RsNxsMsg*>& msg);
|
|
|
|
/*!
|
|
* Stores a list of groups in data store
|
|
* @param msg list of messages
|
|
* @return error code
|
|
*/
|
|
int storeGroup(std::set<RsNxsGrp*>& grp);
|
|
|
|
/*!
|
|
* Completely clear out data stored in
|
|
* this data store and returns this to a state
|
|
* as it was when first constructed
|
|
* This also clears any data store items created in service directory
|
|
*/
|
|
int resetDataStore();
|
|
|
|
private:
|
|
|
|
|
|
RsNxsMsg* getMessage(RetroCursor& c);
|
|
RsNxsGrp* getGroup(RetroCursor& c);
|
|
void initialise();
|
|
|
|
private:
|
|
|
|
|
|
RetroDb* mDb;
|
|
std::list<std::string> msgColumns;
|
|
std::list<std::string> grpColumns;
|
|
|
|
std::string mServiceDir, mDbName;
|
|
uint16_t mServType;
|
|
};
|
|
|
|
#endif // RSDATASERVICE_H
|