mirror of
https://github.com/RetroShare/RetroShare.git
synced 2024-10-01 02:35:48 -04:00
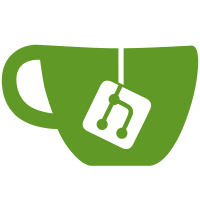
- click in MessageToaster shows the MainWindow and activates the MessagesDialog git-svn-id: http://svn.code.sf.net/p/retroshare/code/trunk@2980 b45a01b8-16f6-495d-af2f-9b41ad6348cc
234 lines
5.9 KiB
C++
234 lines
5.9 KiB
C++
/****************************************************************
|
|
* RetroShare is distributed under the following license:
|
|
*
|
|
* Copyright (C) 2006,2007 The RetroShare Team
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor,
|
|
* Boston, MA 02110-1301, USA.
|
|
****************************************************************/
|
|
|
|
#ifndef _MainWindow_H
|
|
#define _MainWindow_H
|
|
|
|
#include <QtGui>
|
|
#include <QMainWindow>
|
|
#include <QFileDialog>
|
|
#include <QSystemTrayIcon>
|
|
|
|
#ifdef UNFINISHED
|
|
#include "unfinished/ApplicationWindow.h"
|
|
#endif
|
|
|
|
#include "NetworkDialog.h"
|
|
#include "PeersDialog.h"
|
|
#include "SearchDialog.h"
|
|
#include "TransfersDialog.h"
|
|
#include "MessagesDialog.h"
|
|
#include "SharedFilesDialog.h"
|
|
#include "MessengerWindow.h"
|
|
#include "PluginsPage.h"
|
|
#include "ForumsDialog.h"
|
|
|
|
#ifndef RS_RELEASE_VERSION
|
|
#include "ChannelFeed.h"
|
|
#endif
|
|
|
|
#include "bwgraph/bwgraph.h"
|
|
#include "help/browser/helpbrowser.h"
|
|
|
|
#include "ui_MainWindow.h"
|
|
#include "gui/common/rwindow.h"
|
|
#include "idle/idle.h"
|
|
|
|
class PeerStatus;
|
|
class NATStatus;
|
|
class RatesStatus;
|
|
class ForumsDialog;
|
|
|
|
#ifndef RS_RELEASE_VERSION
|
|
class LinksDialog;
|
|
class NewsFeed;
|
|
#endif
|
|
|
|
#ifdef BLOGS
|
|
class BlogsDialog;
|
|
#endif
|
|
|
|
class MainWindow : public RWindow
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
/** Main dialog pages. */
|
|
enum Page {
|
|
Network = 0, /** Network page. */
|
|
Friends, /** Peers page. */
|
|
Search, /** Search page. */
|
|
Transfers, /** Transfers page. */
|
|
SharedDirectories, /** Shared Directories page. */
|
|
Messages, /** Messages page. */
|
|
#ifndef RS_RELEASE_VERSION
|
|
Links, /** Links page. */
|
|
Channels, /** Channels page. */
|
|
#endif
|
|
Forums, /** Forums page. */
|
|
#ifdef BLOGS
|
|
Blogs, /** Blogs page. */
|
|
#endif
|
|
};
|
|
|
|
/** Create main window */
|
|
static MainWindow *Create ();
|
|
|
|
/** Destructor. */
|
|
~MainWindow();
|
|
|
|
/** Shows the MainWindow dialog with focus set to the given page. */
|
|
static void showWindow(Page page);
|
|
/** Set focus to the given page. */
|
|
static void activatePage (Page page);
|
|
|
|
/* A Bit of a Hack... but public variables for
|
|
* the dialogs, so we can add them to the
|
|
* Notify Class...
|
|
*/
|
|
|
|
NetworkDialog *networkDialog;
|
|
PeersDialog *peersDialog;
|
|
SearchDialog *searchDialog;
|
|
TransfersDialog *transfersDialog;
|
|
ChatDialog *chatDialog;
|
|
MessagesDialog *messagesDialog;
|
|
SharedFilesDialog *sharedfilesDialog;
|
|
MessengerWindow *messengerWindow;
|
|
ForumsDialog *forumsDialog;
|
|
Idle *idle;
|
|
|
|
#ifndef RS_RELEASE_VERSION
|
|
ChannelFeed *channelFeed;
|
|
LinksDialog *linksDialog;
|
|
NewsFeed *newsFeed;
|
|
#endif
|
|
|
|
#ifdef BLOGS
|
|
BlogsDialog *blogsFeed;
|
|
#endif
|
|
|
|
#ifdef UNFINISHED
|
|
ApplicationWindow *applicationWindow;
|
|
#endif
|
|
PluginsPage* pluginsPage ;
|
|
|
|
|
|
public slots:
|
|
void updateHashingInfo(const QString&) ;
|
|
void displayErrorMessage(int,int,const QString&) ;
|
|
void postModDirectories(bool update_local);
|
|
void displayDiskSpaceWarning(int loc,int size_limit_mb) ;
|
|
|
|
protected:
|
|
/** Default Constructor */
|
|
MainWindow(QWidget *parent = 0, Qt::WFlags flags = 0);
|
|
|
|
void closeEvent(QCloseEvent *);
|
|
|
|
/** Called when the user changes the UI translation. */
|
|
virtual void retranslateUi();
|
|
|
|
private slots:
|
|
|
|
void updateMenu();
|
|
void updateStatus();
|
|
|
|
void toggleVisibility(QSystemTrayIcon::ActivationReason e);
|
|
void toggleVisibilitycontextmenu();
|
|
|
|
|
|
/** Toolbar fns. */
|
|
void addFriend();
|
|
void showMessengerWindow();
|
|
#ifdef UNFINISHED
|
|
void showApplWindow();
|
|
#endif
|
|
|
|
void showabout();
|
|
void openShareManager();
|
|
void displaySystrayMsg(const QString&,const QString&) ;
|
|
|
|
/** Displays the help browser and displays the most recently viewed help
|
|
* topic. */
|
|
void showHelpDialog();
|
|
/** Called when a child window requests the given help <b>topic</b>. */
|
|
void showHelpDialog(const QString &topic);
|
|
|
|
void showMess();
|
|
void showSettings();
|
|
void setStyle();
|
|
|
|
/** Called when user attempts to quit via quit button*/
|
|
void doQuit();
|
|
|
|
void on_actionQuick_Start_Wizard_activated();
|
|
|
|
|
|
private:
|
|
|
|
/** Create the actions on the tray menu or menubar */
|
|
void createActions();
|
|
|
|
void createTrayIcon();
|
|
|
|
static MainWindow *_instance;
|
|
|
|
/** Defines the actions for the tray menu */
|
|
QAction* _settingsAct;
|
|
QAction* _bandwidthAct;
|
|
QAction* _messengerwindowAct;
|
|
QAction* _messagesAct;
|
|
QAction* _smplayerAct;
|
|
QAction* _helpAct;
|
|
#ifdef UNFINISHED
|
|
QAction* _appAct;
|
|
#endif
|
|
|
|
/** A BandwidthGraph object which handles monitoring RetroShare bandwidth usage */
|
|
BandwidthGraph* _bandwidthGraph;
|
|
|
|
/** Creates a new action for a Main page. */
|
|
QAction* createPageAction(QIcon img, QString text, QActionGroup *group);
|
|
/** Adds a new action to the toolbar. */
|
|
void addAction(QAction *action, const char *slot = 0);
|
|
|
|
void loadStyleSheet(const QString &sheetName);
|
|
|
|
QSystemTrayIcon *trayIcon;
|
|
QAction *toggleVisibilityAction, *toolAct;
|
|
QMenu *trayMenu;
|
|
|
|
PeerStatus *peerstatus;
|
|
NATStatus *natstatus;
|
|
RatesStatus *ratesstatus;
|
|
|
|
QLabel *_hashing_info_label ;
|
|
|
|
QAction *messageAction ;
|
|
|
|
/** Qt Designer generated object */
|
|
Ui::MainWindow ui;
|
|
};
|
|
|
|
#endif
|
|
|