mirror of
https://github.com/RetroShare/RetroShare.git
synced 2025-07-12 01:09:26 -04:00
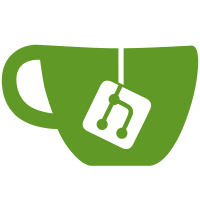
Bugfixes for 'sleep' issues under windows. Extra debugging for transfer module. Fixed channel directory creation. (extra 'break' statement) Fixed very slow transfers on Windows platform (uninitialised variable) Ready for win/linux/osx release now. git-svn-id: http://svn.code.sf.net/p/retroshare/code/trunk@830 b45a01b8-16f6-495d-af2f-9b41ad6348cc
122 lines
2.7 KiB
C++
122 lines
2.7 KiB
C++
#include "ftfilecreator.h"
|
|
|
|
#include "util/utest.h"
|
|
#include <stdlib.h>
|
|
|
|
#include "util/rswin.h"
|
|
|
|
INITTEST();
|
|
|
|
static int test_timeout(ftFileCreator *creator);
|
|
static int test_fill(ftFileCreator *creator);
|
|
|
|
int main()
|
|
{
|
|
/* use ftcreator to create a file on tmp drive */
|
|
ftFileCreator fcreator("/tmp/rs-ftfc-test.dta",100000,"hash",0);
|
|
|
|
test_timeout(&fcreator);
|
|
test_fill(&fcreator);
|
|
|
|
FINALREPORT("RsTlvItem Stack Tests");
|
|
|
|
return TESTRESULT();
|
|
}
|
|
|
|
|
|
int test_timeout(ftFileCreator *creator)
|
|
{
|
|
uint32_t chunk = 1000;
|
|
uint64_t offset = 0;
|
|
int max_timeout = 30;
|
|
int max_offset = chunk * max_timeout;
|
|
int i;
|
|
std::cerr << "60 second test of chunk queue.";
|
|
std::cerr << std::endl;
|
|
|
|
for(i = 0; i < max_timeout; i++)
|
|
{
|
|
creator->getMissingChunk(offset, chunk);
|
|
std::cerr << "Allocated Offset: " << offset << " chunk: " << chunk << std::endl;
|
|
|
|
CHECK(offset <= max_offset);
|
|
sleep(1);
|
|
}
|
|
|
|
std::cerr << "Expect Repeats now";
|
|
std::cerr << std::endl;
|
|
|
|
for(i = 0; i < max_timeout; i++)
|
|
{
|
|
sleep(1);
|
|
creator->getMissingChunk(offset, chunk);
|
|
std::cerr << "Allocated Offset: " << offset << " chunk: " << chunk << std::endl;
|
|
|
|
CHECK(offset <= max_offset);
|
|
}
|
|
REPORT("Chunk Queue");
|
|
|
|
return 1;
|
|
}
|
|
|
|
|
|
int test_fill(ftFileCreator *creator)
|
|
{
|
|
uint32_t chunk = 1000;
|
|
uint64_t offset = 0;
|
|
|
|
uint64_t init_size = creator->getFileSize();
|
|
uint64_t init_trans = creator->getRecvd();
|
|
std::cerr << "Initial FileSize: " << init_size << std::endl;
|
|
std::cerr << "Initial Transferred:" << init_trans << std::endl;
|
|
|
|
while(creator->getMissingChunk(offset, chunk))
|
|
{
|
|
if (chunk == 0)
|
|
{
|
|
std::cerr << "Missing Data already Alloced... wait";
|
|
std::cerr << std::endl;
|
|
sleep(1);
|
|
chunk = 1000;
|
|
continue;
|
|
}
|
|
|
|
/* give it to them */
|
|
void *data = malloc(chunk);
|
|
/* fill with ascending numbers */
|
|
for(int i = 0; i < chunk; i++)
|
|
{
|
|
((uint8_t *) data)[i] = 'a' + i % 27;
|
|
if (i % 27 == 26)
|
|
{
|
|
((uint8_t *) data)[i] = '\n';
|
|
}
|
|
}
|
|
|
|
creator->addFileData(offset, chunk, data);
|
|
free(data);
|
|
#ifndef WINDOWS_SYS
|
|
/********************************** WINDOWS/UNIX SPECIFIC PART ******************/
|
|
usleep(250000); /* 1/4 of sec */
|
|
#else
|
|
/********************************** WINDOWS/UNIX SPECIFIC PART ******************/
|
|
Sleep(250); /* 1/4 of sec */
|
|
#endif
|
|
/********************************** WINDOWS/UNIX SPECIFIC PART ******************/
|
|
|
|
chunk = 1000; /* reset chunk size */
|
|
|
|
}
|
|
|
|
uint64_t end_size = creator->getFileSize();
|
|
uint64_t end_trans = creator->getRecvd();
|
|
std::cerr << "End FileSize: " << end_size << std::endl;
|
|
std::cerr << "End Transferred:" << end_trans << std::endl;
|
|
CHECK(init_size == end_size);
|
|
CHECK(end_trans == end_size);
|
|
|
|
REPORT("Test Fill");
|
|
|
|
return 1;
|
|
}
|
|
|