mirror of
https://github.com/RetroShare/RetroShare.git
synced 2025-01-15 17:37:12 -05:00
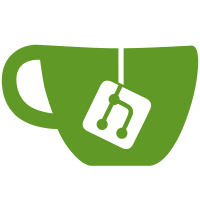
Fix bug in array-like containers serialization which could cause almost infinite loop on malformed input Implement VLQ integer serialization Unify sequence containers serialization code Add support for VLQ serialization also for string size Use VLQ compression for file links Add templated function to fix endiannes for all integer types Use bitset to print flags in binary form Unify serialization code for integral types Serialize 64bit integers types to JSON object with both string and integer representation, so it is posible to have this representation also for containers types like std::vetor or std::map this breaks retrocompatibility but is necessary to support clients written in languages which doesn't have 64 bit integers support such as JavaScript or Dart
60 lines
2.7 KiB
C++
60 lines
2.7 KiB
C++
/*******************************************************************************
|
|
* *
|
|
* libretroshare base64 encoding utilities *
|
|
* *
|
|
* Copyright (C) 2020 Gioacchino Mazzurco <gio@eigenlab.org> *
|
|
* Copyright (C) 2020 Asociación Civil Altermundi <info@altermundi.net> *
|
|
* *
|
|
* This program is free software: you can redistribute it and/or modify *
|
|
* it under the terms of the GNU Lesser General Public License as *
|
|
* published by the Free Software Foundation, either version 3 of the *
|
|
* License, or (at your option) any later version. *
|
|
* *
|
|
* This program is distributed in the hope that it will be useful, *
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of *
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the *
|
|
* GNU Lesser General Public License for more details. *
|
|
* *
|
|
* You should have received a copy of the GNU Lesser General Public License *
|
|
* along with this program. If not, see <https://www.gnu.org/licenses/>. *
|
|
* *
|
|
*******************************************************************************/
|
|
#pragma once
|
|
|
|
/**
|
|
* @file
|
|
* This file provide convenient integer endiannes conversion utilities.
|
|
* Instead of providing them with different names for each type (a la C htonl,
|
|
* htons, ntohl, ntohs ), which make them uncomfortable to use, expose a
|
|
* templated function `rs_endian_fix` to reorder integers representation byets
|
|
* when sending or receiving from the network. */
|
|
|
|
/* enforce LITTLE_ENDIAN on Windows */
|
|
#ifdef WINDOWS_SYS
|
|
# define BYTE_ORDER 1234
|
|
# define LITTLE_ENDIAN 1234
|
|
# define BIG_ENDIAN 4321
|
|
#else
|
|
#include <arpa/inet.h>
|
|
#endif
|
|
|
|
template<typename INTTYPE> inline INTTYPE rs_endian_fix(INTTYPE val)
|
|
{
|
|
#if BYTE_ORDER == LITTLE_ENDIAN
|
|
INTTYPE swapped = 0;
|
|
for (size_t i = 0; i < sizeof(INTTYPE); ++i)
|
|
swapped |= (val >> (8*(sizeof(INTTYPE)-i-1)) & 0xFF) << (8*i);
|
|
return swapped;
|
|
#else
|
|
return hostI;
|
|
#endif
|
|
};
|
|
|
|
#ifndef BYTE_ORDER
|
|
# error "ENDIAN determination Failed (BYTE_ORDER not defined)"
|
|
#endif
|
|
|
|
#if !(BYTE_ORDER == BIG_ENDIAN || BYTE_ORDER == LITTLE_ENDIAN)
|
|
# error "ENDIAN determination Failed (unkown BYTE_ORDER value)"
|
|
#endif
|