mirror of
https://github.com/RetroShare/RetroShare.git
synced 2025-02-18 13:54:07 -05:00
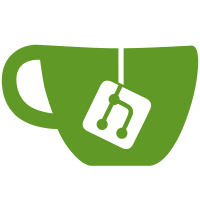
git-svn-id: http://svn.code.sf.net/p/retroshare/code/branches/v0.5-gxs-b1@5953 b45a01b8-16f6-495d-af2f-9b41ad6348cc
6666 lines
332 KiB
C
6666 lines
332 KiB
C
/* A recursive-descent parser generated by peg 0.1.9 */
|
|
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
#define YYRULECOUNT 237
|
|
|
|
/**********************************************************************
|
|
|
|
markdown_parser.leg - markdown parser in C using a PEG grammar.
|
|
(c) 2008 John MacFarlane (jgm at berkeley dot edu).
|
|
|
|
This program is free software; you can redistribute it and/or modify
|
|
it under the terms of the GNU General Public License or the MIT
|
|
license. See LICENSE for details.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
GNU General Public License for more details.
|
|
|
|
***********************************************************************/
|
|
|
|
#include <stdbool.h>
|
|
#include <assert.h>
|
|
#include "markdown_peg.h"
|
|
#include "utility_functions.h"
|
|
|
|
|
|
|
|
/**********************************************************************
|
|
|
|
Definitions for leg parser generator.
|
|
YY_INPUT is the function the parser calls to get new input.
|
|
We take all new input from (static) charbuf.
|
|
|
|
***********************************************************************/
|
|
|
|
|
|
|
|
# define YYSTYPE element *
|
|
#ifdef __DEBUG__
|
|
# define YY_DEBUG 1
|
|
#endif
|
|
|
|
#define YY_INPUT(buf, result, max_size) \
|
|
{ \
|
|
int yyc; \
|
|
if (charbuf && *charbuf != '\0') { \
|
|
yyc= *charbuf++; \
|
|
} else { \
|
|
yyc= EOF; \
|
|
} \
|
|
result= (EOF == yyc) ? 0 : (*(buf)= yyc, 1); \
|
|
}
|
|
|
|
#define YY_RULE(T) T
|
|
|
|
|
|
/**********************************************************************
|
|
|
|
PEG grammar and parser actions for markdown syntax.
|
|
|
|
***********************************************************************/
|
|
|
|
|
|
#ifndef YY_LOCAL
|
|
#define YY_LOCAL(T) static T
|
|
#endif
|
|
#ifndef YY_ACTION
|
|
#define YY_ACTION(T) static T
|
|
#endif
|
|
#ifndef YY_RULE
|
|
#define YY_RULE(T) static T
|
|
#endif
|
|
#ifndef YY_PARSE
|
|
#define YY_PARSE(T) T
|
|
#endif
|
|
#ifndef YYPARSE
|
|
#define YYPARSE yyparse
|
|
#endif
|
|
#ifndef YYPARSEFROM
|
|
#define YYPARSEFROM yyparsefrom
|
|
#endif
|
|
#ifndef YY_INPUT
|
|
#define YY_INPUT(buf, result, max_size) \
|
|
{ \
|
|
int yyc= getchar(); \
|
|
result= (EOF == yyc) ? 0 : (*(buf)= yyc, 1); \
|
|
yyprintf((stderr, "<%c>", yyc)); \
|
|
}
|
|
#endif
|
|
#ifndef YY_BEGIN
|
|
#define YY_BEGIN ( ctx->begin= ctx->pos, 1)
|
|
#endif
|
|
#ifndef YY_END
|
|
#define YY_END ( ctx->end= ctx->pos, 1)
|
|
#endif
|
|
#ifdef YY_DEBUG
|
|
# define yyprintf(args) fprintf args
|
|
#else
|
|
# define yyprintf(args)
|
|
#endif
|
|
#ifndef YYSTYPE
|
|
#define YYSTYPE int
|
|
#endif
|
|
|
|
#ifndef YY_PART
|
|
|
|
typedef struct _yycontext yycontext;
|
|
typedef void (*yyaction)(yycontext *ctx, char *yytext, int yyleng);
|
|
typedef struct _yythunk { int begin, end; yyaction action; struct _yythunk *next; } yythunk;
|
|
|
|
struct _yycontext {
|
|
char *buf;
|
|
int buflen;
|
|
int pos;
|
|
int limit;
|
|
char *text;
|
|
int textlen;
|
|
int begin;
|
|
int end;
|
|
int textmax;
|
|
yythunk *thunks;
|
|
int thunkslen;
|
|
int thunkpos;
|
|
YYSTYPE yy;
|
|
YYSTYPE *val;
|
|
YYSTYPE *vals;
|
|
int valslen;
|
|
#ifdef YY_CTX_MEMBERS
|
|
YY_CTX_MEMBERS
|
|
#endif
|
|
};
|
|
|
|
#ifdef YY_CTX_LOCAL
|
|
#define YY_CTX_PARAM_ yycontext *yyctx,
|
|
#define YY_CTX_PARAM yycontext *yyctx
|
|
#define YY_CTX_ARG_ yyctx,
|
|
#define YY_CTX_ARG yyctx
|
|
#else
|
|
#define YY_CTX_PARAM_
|
|
#define YY_CTX_PARAM
|
|
#define YY_CTX_ARG_
|
|
#define YY_CTX_ARG
|
|
yycontext yyctx0;
|
|
yycontext *yyctx= &yyctx0;
|
|
#endif
|
|
|
|
YY_LOCAL(int) yyrefill(yycontext *ctx)
|
|
{
|
|
int yyn;
|
|
while (ctx->buflen - ctx->pos < 512)
|
|
{
|
|
ctx->buflen *= 2;
|
|
ctx->buf= (char *)realloc(ctx->buf, ctx->buflen);
|
|
}
|
|
YY_INPUT((ctx->buf + ctx->pos), yyn, (ctx->buflen - ctx->pos));
|
|
if (!yyn) return 0;
|
|
ctx->limit += yyn;
|
|
return 1;
|
|
}
|
|
|
|
YY_LOCAL(int) yymatchDot(yycontext *ctx)
|
|
{
|
|
if (ctx->pos >= ctx->limit && !yyrefill(ctx)) return 0;
|
|
++ctx->pos;
|
|
return 1;
|
|
}
|
|
|
|
YY_LOCAL(int) yymatchChar(yycontext *ctx, int c)
|
|
{
|
|
if (ctx->pos >= ctx->limit && !yyrefill(ctx)) return 0;
|
|
if ((unsigned char)ctx->buf[ctx->pos] == c)
|
|
{
|
|
++ctx->pos;
|
|
yyprintf((stderr, " ok yymatchChar(ctx, %c) @ %s\n", c, ctx->buf+ctx->pos));
|
|
return 1;
|
|
}
|
|
yyprintf((stderr, " fail yymatchChar(ctx, %c) @ %s\n", c, ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
|
|
YY_LOCAL(int) yymatchString(yycontext *ctx, char *s)
|
|
{
|
|
int yysav= ctx->pos;
|
|
while (*s)
|
|
{
|
|
if (ctx->pos >= ctx->limit && !yyrefill(ctx)) return 0;
|
|
if (ctx->buf[ctx->pos] != *s)
|
|
{
|
|
ctx->pos= yysav;
|
|
return 0;
|
|
}
|
|
++s;
|
|
++ctx->pos;
|
|
}
|
|
return 1;
|
|
}
|
|
|
|
YY_LOCAL(int) yymatchClass(yycontext *ctx, unsigned char *bits)
|
|
{
|
|
int c;
|
|
if (ctx->pos >= ctx->limit && !yyrefill(ctx)) return 0;
|
|
c= (unsigned char)ctx->buf[ctx->pos];
|
|
if (bits[c >> 3] & (1 << (c & 7)))
|
|
{
|
|
++ctx->pos;
|
|
yyprintf((stderr, " ok yymatchClass @ %s\n", ctx->buf+ctx->pos));
|
|
return 1;
|
|
}
|
|
yyprintf((stderr, " fail yymatchClass @ %s\n", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
|
|
YY_LOCAL(void) yyDo(yycontext *ctx, yyaction action, int begin, int end)
|
|
{
|
|
while (ctx->thunkpos >= ctx->thunkslen)
|
|
{
|
|
ctx->thunkslen *= 2;
|
|
ctx->thunks= (yythunk *)realloc(ctx->thunks, sizeof(yythunk) * ctx->thunkslen);
|
|
}
|
|
ctx->thunks[ctx->thunkpos].begin= begin;
|
|
ctx->thunks[ctx->thunkpos].end= end;
|
|
ctx->thunks[ctx->thunkpos].action= action;
|
|
++ctx->thunkpos;
|
|
}
|
|
|
|
YY_LOCAL(int) yyText(yycontext *ctx, int begin, int end)
|
|
{
|
|
int yyleng= end - begin;
|
|
if (yyleng <= 0)
|
|
yyleng= 0;
|
|
else
|
|
{
|
|
while (ctx->textlen < (yyleng + 1))
|
|
{
|
|
ctx->textlen *= 2;
|
|
ctx->text= (char *)realloc(ctx->text, ctx->textlen);
|
|
}
|
|
memcpy(ctx->text, ctx->buf + begin, yyleng);
|
|
}
|
|
ctx->text[yyleng]= '\0';
|
|
return yyleng;
|
|
}
|
|
|
|
YY_LOCAL(void) yyDone(yycontext *ctx)
|
|
{
|
|
int pos;
|
|
for (pos= 0; pos < ctx->thunkpos; ++pos)
|
|
{
|
|
yythunk *thunk= &ctx->thunks[pos];
|
|
int yyleng= thunk->end ? yyText(ctx, thunk->begin, thunk->end) : thunk->begin;
|
|
yyprintf((stderr, "DO [%d] %p %s\n", pos, thunk->action, ctx->text));
|
|
thunk->action(ctx, ctx->text, yyleng);
|
|
}
|
|
ctx->thunkpos= 0;
|
|
}
|
|
|
|
YY_LOCAL(void) yyCommit(yycontext *ctx)
|
|
{
|
|
if ((ctx->limit -= ctx->pos))
|
|
{
|
|
memmove(ctx->buf, ctx->buf + ctx->pos, ctx->limit);
|
|
}
|
|
ctx->begin -= ctx->pos;
|
|
ctx->end -= ctx->pos;
|
|
ctx->pos= ctx->thunkpos= 0;
|
|
}
|
|
|
|
YY_LOCAL(int) yyAccept(yycontext *ctx, int tp0)
|
|
{
|
|
if (tp0)
|
|
{
|
|
fprintf(stderr, "accept denied at %d\n", tp0);
|
|
return 0;
|
|
}
|
|
else
|
|
{
|
|
yyDone(ctx);
|
|
yyCommit(ctx);
|
|
}
|
|
return 1;
|
|
}
|
|
|
|
YY_LOCAL(void) yyPush(yycontext *ctx, char *text, int count) { ctx->val += count; }
|
|
YY_LOCAL(void) yyPop(yycontext *ctx, char *text, int count) { ctx->val -= count; }
|
|
YY_LOCAL(void) yySet(yycontext *ctx, char *text, int count) { ctx->val[count]= ctx->yy; }
|
|
|
|
#endif /* YY_PART */
|
|
|
|
#define YYACCEPT yyAccept(ctx, yythunkpos0)
|
|
|
|
YY_RULE(int) yy_Notes(yycontext *ctx); /* 237 */
|
|
YY_RULE(int) yy_RawNoteBlock(yycontext *ctx); /* 236 */
|
|
YY_RULE(int) yy_RawNoteReference(yycontext *ctx); /* 235 */
|
|
YY_RULE(int) yy_DoubleQuoteEnd(yycontext *ctx); /* 234 */
|
|
YY_RULE(int) yy_DoubleQuoteStart(yycontext *ctx); /* 233 */
|
|
YY_RULE(int) yy_SingleQuoteEnd(yycontext *ctx); /* 232 */
|
|
YY_RULE(int) yy_SingleQuoteStart(yycontext *ctx); /* 231 */
|
|
YY_RULE(int) yy_EnDash(yycontext *ctx); /* 230 */
|
|
YY_RULE(int) yy_EmDash(yycontext *ctx); /* 229 */
|
|
YY_RULE(int) yy_Apostrophe(yycontext *ctx); /* 228 */
|
|
YY_RULE(int) yy_DoubleQuoted(yycontext *ctx); /* 227 */
|
|
YY_RULE(int) yy_SingleQuoted(yycontext *ctx); /* 226 */
|
|
YY_RULE(int) yy_Dash(yycontext *ctx); /* 225 */
|
|
YY_RULE(int) yy_Ellipsis(yycontext *ctx); /* 224 */
|
|
YY_RULE(int) yy_Digit(yycontext *ctx); /* 223 */
|
|
YY_RULE(int) yy_ExtendedSpecialChar(yycontext *ctx); /* 222 */
|
|
YY_RULE(int) yy_AlphanumericAscii(yycontext *ctx); /* 221 */
|
|
YY_RULE(int) yy_Quoted(yycontext *ctx); /* 220 */
|
|
YY_RULE(int) yy_HtmlTag(yycontext *ctx); /* 219 */
|
|
YY_RULE(int) yy_Ticks5(yycontext *ctx); /* 218 */
|
|
YY_RULE(int) yy_Ticks4(yycontext *ctx); /* 217 */
|
|
YY_RULE(int) yy_Ticks3(yycontext *ctx); /* 216 */
|
|
YY_RULE(int) yy_Ticks2(yycontext *ctx); /* 215 */
|
|
YY_RULE(int) yy_Ticks1(yycontext *ctx); /* 214 */
|
|
YY_RULE(int) yy_SkipBlock(yycontext *ctx); /* 213 */
|
|
YY_RULE(int) yy_References(yycontext *ctx); /* 212 */
|
|
YY_RULE(int) yy_EmptyTitle(yycontext *ctx); /* 211 */
|
|
YY_RULE(int) yy_RefTitleParens(yycontext *ctx); /* 210 */
|
|
YY_RULE(int) yy_RefTitleDouble(yycontext *ctx); /* 209 */
|
|
YY_RULE(int) yy_RefTitleSingle(yycontext *ctx); /* 208 */
|
|
YY_RULE(int) yy_RefTitle(yycontext *ctx); /* 207 */
|
|
YY_RULE(int) yy_RefSrc(yycontext *ctx); /* 206 */
|
|
YY_RULE(int) yy_AutoLinkEmail(yycontext *ctx); /* 205 */
|
|
YY_RULE(int) yy_AutoLinkUrl(yycontext *ctx); /* 204 */
|
|
YY_RULE(int) yy_TitleDouble(yycontext *ctx); /* 203 */
|
|
YY_RULE(int) yy_TitleSingle(yycontext *ctx); /* 202 */
|
|
YY_RULE(int) yy_Nonspacechar(yycontext *ctx); /* 201 */
|
|
YY_RULE(int) yy_SourceContents(yycontext *ctx); /* 200 */
|
|
YY_RULE(int) yy_Title(yycontext *ctx); /* 199 */
|
|
YY_RULE(int) yy_Source(yycontext *ctx); /* 198 */
|
|
YY_RULE(int) yy_Label(yycontext *ctx); /* 197 */
|
|
YY_RULE(int) yy_ReferenceLinkSingle(yycontext *ctx); /* 196 */
|
|
YY_RULE(int) yy_ReferenceLinkDouble(yycontext *ctx); /* 195 */
|
|
YY_RULE(int) yy_AutoLink(yycontext *ctx); /* 194 */
|
|
YY_RULE(int) yy_ReferenceLink(yycontext *ctx); /* 193 */
|
|
YY_RULE(int) yy_ExplicitLink(yycontext *ctx); /* 192 */
|
|
YY_RULE(int) yy_StrongUl(yycontext *ctx); /* 191 */
|
|
YY_RULE(int) yy_StrongStar(yycontext *ctx); /* 190 */
|
|
YY_RULE(int) yy_Whitespace(yycontext *ctx); /* 189 */
|
|
YY_RULE(int) yy_EmphUl(yycontext *ctx); /* 188 */
|
|
YY_RULE(int) yy_EmphStar(yycontext *ctx); /* 187 */
|
|
YY_RULE(int) yy_StarLine(yycontext *ctx); /* 186 */
|
|
YY_RULE(int) yy_UlLine(yycontext *ctx); /* 185 */
|
|
YY_RULE(int) yy_SpecialChar(yycontext *ctx); /* 184 */
|
|
YY_RULE(int) yy_Eof(yycontext *ctx); /* 183 */
|
|
YY_RULE(int) yy_NormalEndline(yycontext *ctx); /* 182 */
|
|
YY_RULE(int) yy_TerminalEndline(yycontext *ctx); /* 181 */
|
|
YY_RULE(int) yy_LineBreak(yycontext *ctx); /* 180 */
|
|
YY_RULE(int) yy_CharEntity(yycontext *ctx); /* 179 */
|
|
YY_RULE(int) yy_DecEntity(yycontext *ctx); /* 178 */
|
|
YY_RULE(int) yy_HexEntity(yycontext *ctx); /* 177 */
|
|
YY_RULE(int) yy_AposChunk(yycontext *ctx); /* 176 */
|
|
YY_RULE(int) yy_Alphanumeric(yycontext *ctx); /* 175 */
|
|
YY_RULE(int) yy_StrChunk(yycontext *ctx); /* 174 */
|
|
YY_RULE(int) yy_NormalChar(yycontext *ctx); /* 173 */
|
|
YY_RULE(int) yy_Symbol(yycontext *ctx); /* 172 */
|
|
YY_RULE(int) yy_Smart(yycontext *ctx); /* 171 */
|
|
YY_RULE(int) yy_EscapedChar(yycontext *ctx); /* 170 */
|
|
YY_RULE(int) yy_Entity(yycontext *ctx); /* 169 */
|
|
YY_RULE(int) yy_RawHtml(yycontext *ctx); /* 168 */
|
|
YY_RULE(int) yy_Code(yycontext *ctx); /* 167 */
|
|
YY_RULE(int) yy_InlineNote(yycontext *ctx); /* 166 */
|
|
YY_RULE(int) yy_NoteReference(yycontext *ctx); /* 165 */
|
|
YY_RULE(int) yy_Link(yycontext *ctx); /* 164 */
|
|
YY_RULE(int) yy_Image(yycontext *ctx); /* 163 */
|
|
YY_RULE(int) yy_Emph(yycontext *ctx); /* 162 */
|
|
YY_RULE(int) yy_Strong(yycontext *ctx); /* 161 */
|
|
YY_RULE(int) yy_Space(yycontext *ctx); /* 160 */
|
|
YY_RULE(int) yy_UlOrStarLine(yycontext *ctx); /* 159 */
|
|
YY_RULE(int) yy_Str(yycontext *ctx); /* 158 */
|
|
YY_RULE(int) yy_InStyleTags(yycontext *ctx); /* 157 */
|
|
YY_RULE(int) yy_StyleClose(yycontext *ctx); /* 156 */
|
|
YY_RULE(int) yy_StyleOpen(yycontext *ctx); /* 155 */
|
|
YY_RULE(int) yy_HtmlBlockType(yycontext *ctx); /* 154 */
|
|
YY_RULE(int) yy_HtmlBlockSelfClosing(yycontext *ctx); /* 153 */
|
|
YY_RULE(int) yy_HtmlComment(yycontext *ctx); /* 152 */
|
|
YY_RULE(int) yy_HtmlBlockInTags(yycontext *ctx); /* 151 */
|
|
YY_RULE(int) yy_HtmlBlockScript(yycontext *ctx); /* 150 */
|
|
YY_RULE(int) yy_HtmlBlockCloseScript(yycontext *ctx); /* 149 */
|
|
YY_RULE(int) yy_HtmlBlockOpenScript(yycontext *ctx); /* 148 */
|
|
YY_RULE(int) yy_HtmlBlockTr(yycontext *ctx); /* 147 */
|
|
YY_RULE(int) yy_HtmlBlockCloseTr(yycontext *ctx); /* 146 */
|
|
YY_RULE(int) yy_HtmlBlockOpenTr(yycontext *ctx); /* 145 */
|
|
YY_RULE(int) yy_HtmlBlockThead(yycontext *ctx); /* 144 */
|
|
YY_RULE(int) yy_HtmlBlockCloseThead(yycontext *ctx); /* 143 */
|
|
YY_RULE(int) yy_HtmlBlockOpenThead(yycontext *ctx); /* 142 */
|
|
YY_RULE(int) yy_HtmlBlockTh(yycontext *ctx); /* 141 */
|
|
YY_RULE(int) yy_HtmlBlockCloseTh(yycontext *ctx); /* 140 */
|
|
YY_RULE(int) yy_HtmlBlockOpenTh(yycontext *ctx); /* 139 */
|
|
YY_RULE(int) yy_HtmlBlockTfoot(yycontext *ctx); /* 138 */
|
|
YY_RULE(int) yy_HtmlBlockCloseTfoot(yycontext *ctx); /* 137 */
|
|
YY_RULE(int) yy_HtmlBlockOpenTfoot(yycontext *ctx); /* 136 */
|
|
YY_RULE(int) yy_HtmlBlockTd(yycontext *ctx); /* 135 */
|
|
YY_RULE(int) yy_HtmlBlockCloseTd(yycontext *ctx); /* 134 */
|
|
YY_RULE(int) yy_HtmlBlockOpenTd(yycontext *ctx); /* 133 */
|
|
YY_RULE(int) yy_HtmlBlockTbody(yycontext *ctx); /* 132 */
|
|
YY_RULE(int) yy_HtmlBlockCloseTbody(yycontext *ctx); /* 131 */
|
|
YY_RULE(int) yy_HtmlBlockOpenTbody(yycontext *ctx); /* 130 */
|
|
YY_RULE(int) yy_HtmlBlockLi(yycontext *ctx); /* 129 */
|
|
YY_RULE(int) yy_HtmlBlockCloseLi(yycontext *ctx); /* 128 */
|
|
YY_RULE(int) yy_HtmlBlockOpenLi(yycontext *ctx); /* 127 */
|
|
YY_RULE(int) yy_HtmlBlockFrameset(yycontext *ctx); /* 126 */
|
|
YY_RULE(int) yy_HtmlBlockCloseFrameset(yycontext *ctx); /* 125 */
|
|
YY_RULE(int) yy_HtmlBlockOpenFrameset(yycontext *ctx); /* 124 */
|
|
YY_RULE(int) yy_HtmlBlockDt(yycontext *ctx); /* 123 */
|
|
YY_RULE(int) yy_HtmlBlockCloseDt(yycontext *ctx); /* 122 */
|
|
YY_RULE(int) yy_HtmlBlockOpenDt(yycontext *ctx); /* 121 */
|
|
YY_RULE(int) yy_HtmlBlockDd(yycontext *ctx); /* 120 */
|
|
YY_RULE(int) yy_HtmlBlockCloseDd(yycontext *ctx); /* 119 */
|
|
YY_RULE(int) yy_HtmlBlockOpenDd(yycontext *ctx); /* 118 */
|
|
YY_RULE(int) yy_HtmlBlockUl(yycontext *ctx); /* 117 */
|
|
YY_RULE(int) yy_HtmlBlockCloseUl(yycontext *ctx); /* 116 */
|
|
YY_RULE(int) yy_HtmlBlockOpenUl(yycontext *ctx); /* 115 */
|
|
YY_RULE(int) yy_HtmlBlockTable(yycontext *ctx); /* 114 */
|
|
YY_RULE(int) yy_HtmlBlockCloseTable(yycontext *ctx); /* 113 */
|
|
YY_RULE(int) yy_HtmlBlockOpenTable(yycontext *ctx); /* 112 */
|
|
YY_RULE(int) yy_HtmlBlockPre(yycontext *ctx); /* 111 */
|
|
YY_RULE(int) yy_HtmlBlockClosePre(yycontext *ctx); /* 110 */
|
|
YY_RULE(int) yy_HtmlBlockOpenPre(yycontext *ctx); /* 109 */
|
|
YY_RULE(int) yy_HtmlBlockP(yycontext *ctx); /* 108 */
|
|
YY_RULE(int) yy_HtmlBlockCloseP(yycontext *ctx); /* 107 */
|
|
YY_RULE(int) yy_HtmlBlockOpenP(yycontext *ctx); /* 106 */
|
|
YY_RULE(int) yy_HtmlBlockOl(yycontext *ctx); /* 105 */
|
|
YY_RULE(int) yy_HtmlBlockCloseOl(yycontext *ctx); /* 104 */
|
|
YY_RULE(int) yy_HtmlBlockOpenOl(yycontext *ctx); /* 103 */
|
|
YY_RULE(int) yy_HtmlBlockNoscript(yycontext *ctx); /* 102 */
|
|
YY_RULE(int) yy_HtmlBlockCloseNoscript(yycontext *ctx); /* 101 */
|
|
YY_RULE(int) yy_HtmlBlockOpenNoscript(yycontext *ctx); /* 100 */
|
|
YY_RULE(int) yy_HtmlBlockNoframes(yycontext *ctx); /* 99 */
|
|
YY_RULE(int) yy_HtmlBlockCloseNoframes(yycontext *ctx); /* 98 */
|
|
YY_RULE(int) yy_HtmlBlockOpenNoframes(yycontext *ctx); /* 97 */
|
|
YY_RULE(int) yy_HtmlBlockMenu(yycontext *ctx); /* 96 */
|
|
YY_RULE(int) yy_HtmlBlockCloseMenu(yycontext *ctx); /* 95 */
|
|
YY_RULE(int) yy_HtmlBlockOpenMenu(yycontext *ctx); /* 94 */
|
|
YY_RULE(int) yy_HtmlBlockH6(yycontext *ctx); /* 93 */
|
|
YY_RULE(int) yy_HtmlBlockCloseH6(yycontext *ctx); /* 92 */
|
|
YY_RULE(int) yy_HtmlBlockOpenH6(yycontext *ctx); /* 91 */
|
|
YY_RULE(int) yy_HtmlBlockH5(yycontext *ctx); /* 90 */
|
|
YY_RULE(int) yy_HtmlBlockCloseH5(yycontext *ctx); /* 89 */
|
|
YY_RULE(int) yy_HtmlBlockOpenH5(yycontext *ctx); /* 88 */
|
|
YY_RULE(int) yy_HtmlBlockH4(yycontext *ctx); /* 87 */
|
|
YY_RULE(int) yy_HtmlBlockCloseH4(yycontext *ctx); /* 86 */
|
|
YY_RULE(int) yy_HtmlBlockOpenH4(yycontext *ctx); /* 85 */
|
|
YY_RULE(int) yy_HtmlBlockH3(yycontext *ctx); /* 84 */
|
|
YY_RULE(int) yy_HtmlBlockCloseH3(yycontext *ctx); /* 83 */
|
|
YY_RULE(int) yy_HtmlBlockOpenH3(yycontext *ctx); /* 82 */
|
|
YY_RULE(int) yy_HtmlBlockH2(yycontext *ctx); /* 81 */
|
|
YY_RULE(int) yy_HtmlBlockCloseH2(yycontext *ctx); /* 80 */
|
|
YY_RULE(int) yy_HtmlBlockOpenH2(yycontext *ctx); /* 79 */
|
|
YY_RULE(int) yy_HtmlBlockH1(yycontext *ctx); /* 78 */
|
|
YY_RULE(int) yy_HtmlBlockCloseH1(yycontext *ctx); /* 77 */
|
|
YY_RULE(int) yy_HtmlBlockOpenH1(yycontext *ctx); /* 76 */
|
|
YY_RULE(int) yy_HtmlBlockForm(yycontext *ctx); /* 75 */
|
|
YY_RULE(int) yy_HtmlBlockCloseForm(yycontext *ctx); /* 74 */
|
|
YY_RULE(int) yy_HtmlBlockOpenForm(yycontext *ctx); /* 73 */
|
|
YY_RULE(int) yy_HtmlBlockFieldset(yycontext *ctx); /* 72 */
|
|
YY_RULE(int) yy_HtmlBlockCloseFieldset(yycontext *ctx); /* 71 */
|
|
YY_RULE(int) yy_HtmlBlockOpenFieldset(yycontext *ctx); /* 70 */
|
|
YY_RULE(int) yy_HtmlBlockDl(yycontext *ctx); /* 69 */
|
|
YY_RULE(int) yy_HtmlBlockCloseDl(yycontext *ctx); /* 68 */
|
|
YY_RULE(int) yy_HtmlBlockOpenDl(yycontext *ctx); /* 67 */
|
|
YY_RULE(int) yy_HtmlBlockDiv(yycontext *ctx); /* 66 */
|
|
YY_RULE(int) yy_HtmlBlockCloseDiv(yycontext *ctx); /* 65 */
|
|
YY_RULE(int) yy_HtmlBlockOpenDiv(yycontext *ctx); /* 64 */
|
|
YY_RULE(int) yy_HtmlBlockDir(yycontext *ctx); /* 63 */
|
|
YY_RULE(int) yy_HtmlBlockCloseDir(yycontext *ctx); /* 62 */
|
|
YY_RULE(int) yy_HtmlBlockOpenDir(yycontext *ctx); /* 61 */
|
|
YY_RULE(int) yy_HtmlBlockCenter(yycontext *ctx); /* 60 */
|
|
YY_RULE(int) yy_HtmlBlockCloseCenter(yycontext *ctx); /* 59 */
|
|
YY_RULE(int) yy_HtmlBlockOpenCenter(yycontext *ctx); /* 58 */
|
|
YY_RULE(int) yy_HtmlBlockBlockquote(yycontext *ctx); /* 57 */
|
|
YY_RULE(int) yy_HtmlBlockCloseBlockquote(yycontext *ctx); /* 56 */
|
|
YY_RULE(int) yy_HtmlBlockOpenBlockquote(yycontext *ctx); /* 55 */
|
|
YY_RULE(int) yy_HtmlBlockAddress(yycontext *ctx); /* 54 */
|
|
YY_RULE(int) yy_HtmlBlockCloseAddress(yycontext *ctx); /* 53 */
|
|
YY_RULE(int) yy_HtmlAttribute(yycontext *ctx); /* 52 */
|
|
YY_RULE(int) yy_Spnl(yycontext *ctx); /* 51 */
|
|
YY_RULE(int) yy_HtmlBlockOpenAddress(yycontext *ctx); /* 50 */
|
|
YY_RULE(int) yy_OptionallyIndentedLine(yycontext *ctx); /* 49 */
|
|
YY_RULE(int) yy_Indent(yycontext *ctx); /* 48 */
|
|
YY_RULE(int) yy_ListBlockLine(yycontext *ctx); /* 47 */
|
|
YY_RULE(int) yy_ListContinuationBlock(yycontext *ctx); /* 46 */
|
|
YY_RULE(int) yy_ListBlock(yycontext *ctx); /* 45 */
|
|
YY_RULE(int) yy_ListItem(yycontext *ctx); /* 44 */
|
|
YY_RULE(int) yy_Enumerator(yycontext *ctx); /* 43 */
|
|
YY_RULE(int) yy_ListItemTight(yycontext *ctx); /* 42 */
|
|
YY_RULE(int) yy_ListLoose(yycontext *ctx); /* 41 */
|
|
YY_RULE(int) yy_ListTight(yycontext *ctx); /* 40 */
|
|
YY_RULE(int) yy_Spacechar(yycontext *ctx); /* 39 */
|
|
YY_RULE(int) yy_Bullet(yycontext *ctx); /* 38 */
|
|
YY_RULE(int) yy_VerbatimChunk(yycontext *ctx); /* 37 */
|
|
YY_RULE(int) yy_IndentedLine(yycontext *ctx); /* 36 */
|
|
YY_RULE(int) yy_NonblankIndentedLine(yycontext *ctx); /* 35 */
|
|
YY_RULE(int) yy_Line(yycontext *ctx); /* 34 */
|
|
YY_RULE(int) yy_BlockQuoteRaw(yycontext *ctx); /* 33 */
|
|
YY_RULE(int) yy_Endline(yycontext *ctx); /* 32 */
|
|
YY_RULE(int) yy_RawLine(yycontext *ctx); /* 31 */
|
|
YY_RULE(int) yy_SetextBottom2(yycontext *ctx); /* 30 */
|
|
YY_RULE(int) yy_SetextBottom1(yycontext *ctx); /* 29 */
|
|
YY_RULE(int) yy_SetextHeading2(yycontext *ctx); /* 28 */
|
|
YY_RULE(int) yy_SetextHeading1(yycontext *ctx); /* 27 */
|
|
YY_RULE(int) yy_SetextHeading(yycontext *ctx); /* 26 */
|
|
YY_RULE(int) yy_AtxHeading(yycontext *ctx); /* 25 */
|
|
YY_RULE(int) yy_AtxStart(yycontext *ctx); /* 24 */
|
|
YY_RULE(int) yy_Inline(yycontext *ctx); /* 23 */
|
|
YY_RULE(int) yy_Sp(yycontext *ctx); /* 22 */
|
|
YY_RULE(int) yy_Newline(yycontext *ctx); /* 21 */
|
|
YY_RULE(int) yy_AtxInline(yycontext *ctx); /* 20 */
|
|
YY_RULE(int) yy_Inlines(yycontext *ctx); /* 19 */
|
|
YY_RULE(int) yy_NonindentSpace(yycontext *ctx); /* 18 */
|
|
YY_RULE(int) yy_Plain(yycontext *ctx); /* 17 */
|
|
YY_RULE(int) yy_Para(yycontext *ctx); /* 16 */
|
|
YY_RULE(int) yy_StyleBlock(yycontext *ctx); /* 15 */
|
|
YY_RULE(int) yy_HtmlBlock(yycontext *ctx); /* 14 */
|
|
YY_RULE(int) yy_BulletList(yycontext *ctx); /* 13 */
|
|
YY_RULE(int) yy_OrderedList(yycontext *ctx); /* 12 */
|
|
YY_RULE(int) yy_Heading(yycontext *ctx); /* 11 */
|
|
YY_RULE(int) yy_HorizontalRule(yycontext *ctx); /* 10 */
|
|
YY_RULE(int) yy_Reference(yycontext *ctx); /* 9 */
|
|
YY_RULE(int) yy_Note(yycontext *ctx); /* 8 */
|
|
YY_RULE(int) yy_Verbatim(yycontext *ctx); /* 7 */
|
|
YY_RULE(int) yy_BlockQuote(yycontext *ctx); /* 6 */
|
|
YY_RULE(int) yy_BlankLine(yycontext *ctx); /* 5 */
|
|
YY_RULE(int) yy_Block(yycontext *ctx); /* 4 */
|
|
YY_RULE(int) yy_StartList(yycontext *ctx); /* 3 */
|
|
YY_RULE(int) yy_BOM(yycontext *ctx); /* 2 */
|
|
YY_RULE(int) yy_Doc(yycontext *ctx); /* 1 */
|
|
|
|
YY_ACTION(void) yy_3_RawNoteBlock(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_3_RawNoteBlock\n"));
|
|
yy = mk_str_from_list(a, true);
|
|
yy->key = RAW;
|
|
;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_RawNoteBlock(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_RawNoteBlock\n"));
|
|
a = cons(mk_str(yytext), a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_RawNoteBlock(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_RawNoteBlock\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_Notes(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_Notes\n"));
|
|
notes = reverse(a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_Notes(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Notes\n"));
|
|
a = cons(b, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_InlineNote(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_InlineNote\n"));
|
|
yy = mk_list(NOTE, a);
|
|
yy->contents.str = 0; ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_InlineNote(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_InlineNote\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_3_Note(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define ref ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_3_Note\n"));
|
|
yy = mk_list(NOTE, a);
|
|
yy->contents.str = strdup(ref->contents.str);
|
|
;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
#undef ref
|
|
}
|
|
YY_ACTION(void) yy_2_Note(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define ref ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_Note\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
#undef ref
|
|
}
|
|
YY_ACTION(void) yy_1_Note(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define ref ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Note\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
#undef ref
|
|
}
|
|
YY_ACTION(void) yy_1_RawNoteReference(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_RawNoteReference\n"));
|
|
yy = mk_str(yytext); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_NoteReference(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define ref ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_NoteReference\n"));
|
|
element *match;
|
|
if (find_note(&match, ref->contents.str)) {
|
|
yy = mk_element(NOTE);
|
|
assert(match->children != NULL);
|
|
yy->children = match->children;
|
|
yy->contents.str = 0;
|
|
} else {
|
|
char *s;
|
|
s = malloc(strlen(ref->contents.str) + 4);
|
|
sprintf(s, "[^%s]", ref->contents.str);
|
|
yy = mk_str(s);
|
|
free(s);
|
|
}
|
|
;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef ref
|
|
}
|
|
YY_ACTION(void) yy_2_DoubleQuoted(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_DoubleQuoted\n"));
|
|
yy = mk_list(DOUBLEQUOTED, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_DoubleQuoted(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_DoubleQuoted\n"));
|
|
a = cons(b, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_SingleQuoted(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_SingleQuoted\n"));
|
|
yy = mk_list(SINGLEQUOTED, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_SingleQuoted(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_SingleQuoted\n"));
|
|
a = cons(b, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_EmDash(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_EmDash\n"));
|
|
yy = mk_element(EMDASH); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_EnDash(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_EnDash\n"));
|
|
yy = mk_element(ENDASH); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_Ellipsis(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Ellipsis\n"));
|
|
yy = mk_element(ELLIPSIS); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_Apostrophe(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Apostrophe\n"));
|
|
yy = mk_element(APOSTROPHE); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_Line(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Line\n"));
|
|
yy = mk_str(yytext); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_StartList(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_StartList\n"));
|
|
yy = NULL; ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_RawHtml(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_RawHtml\n"));
|
|
if (extension(EXT_FILTER_HTML)) {
|
|
yy = mk_list(LIST, NULL);
|
|
} else {
|
|
yy = mk_str(yytext);
|
|
yy->key = HTML;
|
|
}
|
|
;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_Code(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Code\n"));
|
|
yy = mk_str(yytext); yy->key = CODE; ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_2_References(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_References\n"));
|
|
references = reverse(a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_References(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_References\n"));
|
|
a = cons(b, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_RefTitle(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_RefTitle\n"));
|
|
yy = mk_str(yytext); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_RefSrc(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_RefSrc\n"));
|
|
yy = mk_str(yytext);
|
|
yy->key = HTML; ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_2_Label(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_Label\n"));
|
|
yy = mk_list(LIST, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_Label(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Label\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_Reference(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define t ctx->val[-1]
|
|
#define s ctx->val[-2]
|
|
#define l ctx->val[-3]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Reference\n"));
|
|
yy = mk_link(l->children, s->contents.str, t->contents.str);
|
|
free_element(s);
|
|
free_element(t);
|
|
free(l);
|
|
yy->key = REFERENCE; ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef t
|
|
#undef s
|
|
#undef l
|
|
}
|
|
YY_ACTION(void) yy_1_AutoLinkEmail(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_AutoLinkEmail\n"));
|
|
char *mailto = malloc(strlen(yytext) + 8);
|
|
sprintf(mailto, "mailto:%s", yytext);
|
|
yy = mk_link(mk_str(yytext), mailto, "");
|
|
free(mailto);
|
|
;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_AutoLinkUrl(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_AutoLinkUrl\n"));
|
|
yy = mk_link(mk_str(yytext), yytext, ""); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_Title(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Title\n"));
|
|
yy = mk_str(yytext); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_Source(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Source\n"));
|
|
yy = mk_str(yytext); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_ExplicitLink(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define t ctx->val[-1]
|
|
#define s ctx->val[-2]
|
|
#define l ctx->val[-3]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_ExplicitLink\n"));
|
|
yy = mk_link(l->children, s->contents.str, t->contents.str);
|
|
free_element(s);
|
|
free_element(t);
|
|
free(l); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef t
|
|
#undef s
|
|
#undef l
|
|
}
|
|
YY_ACTION(void) yy_1_ReferenceLinkSingle(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_ReferenceLinkSingle\n"));
|
|
link match;
|
|
if (find_reference(&match, a->children)) {
|
|
yy = mk_link(a->children, match.url, match.title);
|
|
free(a);
|
|
}
|
|
else {
|
|
element *result;
|
|
result = mk_element(LIST);
|
|
result->children = cons(mk_str("["), cons(a, cons(mk_str("]"), mk_str(yytext))));
|
|
yy = result;
|
|
}
|
|
;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_ReferenceLinkDouble(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_ReferenceLinkDouble\n"));
|
|
link match;
|
|
if (find_reference(&match, b->children)) {
|
|
yy = mk_link(a->children, match.url, match.title);
|
|
free(a);
|
|
free_element_list(b);
|
|
} else {
|
|
element *result;
|
|
result = mk_element(LIST);
|
|
result->children = cons(mk_str("["), cons(a, cons(mk_str("]"), cons(mk_str(yytext),
|
|
cons(mk_str("["), cons(b, mk_str("]")))))));
|
|
yy = result;
|
|
}
|
|
;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_Image(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Image\n"));
|
|
if (yy->key == LINK) {
|
|
yy->key = IMAGE;
|
|
} else {
|
|
element *result;
|
|
result = yy;
|
|
yy->children = cons(mk_str("!"), result->children);
|
|
} ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_2_StrongUl(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_StrongUl\n"));
|
|
yy = mk_list(STRONG, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_StrongUl(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_StrongUl\n"));
|
|
a = cons(b, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_StrongStar(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_StrongStar\n"));
|
|
yy = mk_list(STRONG, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_StrongStar(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_StrongStar\n"));
|
|
a = cons(b, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_3_EmphUl(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_3_EmphUl\n"));
|
|
yy = mk_list(EMPH, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_EmphUl(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_EmphUl\n"));
|
|
a = cons(b, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_EmphUl(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_EmphUl\n"));
|
|
a = cons(b, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_3_EmphStar(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_3_EmphStar\n"));
|
|
yy = mk_list(EMPH, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_EmphStar(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_EmphStar\n"));
|
|
a = cons(b, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_EmphStar(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_EmphStar\n"));
|
|
a = cons(b, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_UlOrStarLine(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_UlOrStarLine\n"));
|
|
yy = mk_str(yytext); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_Symbol(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Symbol\n"));
|
|
yy = mk_str(yytext); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_LineBreak(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_LineBreak\n"));
|
|
yy = mk_element(LINEBREAK); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_TerminalEndline(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_TerminalEndline\n"));
|
|
yy = NULL; ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_NormalEndline(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_NormalEndline\n"));
|
|
yy = mk_str("\n");
|
|
yy->key = SPACE; ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_Entity(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Entity\n"));
|
|
yy = mk_str(yytext); yy->key = HTML; ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_EscapedChar(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_EscapedChar\n"));
|
|
yy = mk_str(yytext); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_AposChunk(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_AposChunk\n"));
|
|
yy = mk_element(APOSTROPHE); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_StrChunk(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_StrChunk\n"));
|
|
yy = mk_str(yytext); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_3_Str(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_3_Str\n"));
|
|
if (a->next == NULL) { yy = a; } else { yy = mk_list(LIST, a); } ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_Str(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_Str\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_Str(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Str\n"));
|
|
a = cons(mk_str(yytext), a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_Space(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Space\n"));
|
|
yy = mk_str(" ");
|
|
yy->key = SPACE; ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_3_Inlines(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define c ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_3_Inlines\n"));
|
|
yy = mk_list(LIST, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef c
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_Inlines(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define c ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_Inlines\n"));
|
|
a = cons(c, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef c
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_Inlines(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define c ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Inlines\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef c
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_StyleBlock(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_StyleBlock\n"));
|
|
if (extension(EXT_FILTER_STYLES)) {
|
|
yy = mk_list(LIST, NULL);
|
|
} else {
|
|
yy = mk_str(yytext);
|
|
yy->key = HTMLBLOCK;
|
|
}
|
|
;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_HtmlBlock(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_HtmlBlock\n"));
|
|
if (extension(EXT_FILTER_HTML)) {
|
|
yy = mk_list(LIST, NULL);
|
|
} else {
|
|
yy = mk_str(yytext);
|
|
yy->key = HTMLBLOCK;
|
|
}
|
|
;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_OrderedList(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_OrderedList\n"));
|
|
yy->key = ORDEREDLIST; ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_3_ListContinuationBlock(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_3_ListContinuationBlock\n"));
|
|
yy = mk_str_from_list(a, false); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_ListContinuationBlock(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_ListContinuationBlock\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_ListContinuationBlock(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_ListContinuationBlock\n"));
|
|
if (strlen(yytext) == 0)
|
|
a = cons(mk_str("\001"), a); /* block separator */
|
|
else
|
|
a = cons(mk_str(yytext), a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_3_ListBlock(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_3_ListBlock\n"));
|
|
yy = mk_str_from_list(a, false); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_ListBlock(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_ListBlock\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_ListBlock(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_ListBlock\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_3_ListItemTight(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_3_ListItemTight\n"));
|
|
element *raw;
|
|
raw = mk_str_from_list(a, false);
|
|
raw->key = RAW;
|
|
yy = mk_element(LISTITEM);
|
|
yy->children = raw;
|
|
;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_ListItemTight(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_ListItemTight\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_ListItemTight(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_ListItemTight\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_3_ListItem(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_3_ListItem\n"));
|
|
element *raw;
|
|
raw = mk_str_from_list(a, false);
|
|
raw->key = RAW;
|
|
yy = mk_element(LISTITEM);
|
|
yy->children = raw;
|
|
;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_ListItem(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_ListItem\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_ListItem(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_ListItem\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_ListLoose(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_ListLoose\n"));
|
|
yy = mk_list(LIST, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_ListLoose(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define b ctx->val[-1]
|
|
#define a ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_ListLoose\n"));
|
|
element *li;
|
|
li = b->children;
|
|
li->contents.str = realloc(li->contents.str, strlen(li->contents.str) + 3);
|
|
strcat(li->contents.str, "\n\n"); /* In loose list, \n\n added to end of each element */
|
|
a = cons(b, a);
|
|
;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef b
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_ListTight(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_ListTight\n"));
|
|
yy = mk_list(LIST, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_ListTight(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_ListTight\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_BulletList(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_BulletList\n"));
|
|
yy->key = BULLETLIST; ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_HorizontalRule(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_HorizontalRule\n"));
|
|
yy = mk_element(HRULE); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_2_Verbatim(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_Verbatim\n"));
|
|
yy = mk_str_from_list(a, false);
|
|
yy->key = VERBATIM; ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_Verbatim(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Verbatim\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_3_VerbatimChunk(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_3_VerbatimChunk\n"));
|
|
yy = mk_str_from_list(a, false); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_VerbatimChunk(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_VerbatimChunk\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_VerbatimChunk(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_VerbatimChunk\n"));
|
|
a = cons(mk_str("\n"), a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_4_BlockQuoteRaw(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_4_BlockQuoteRaw\n"));
|
|
yy = mk_str_from_list(a, true);
|
|
yy->key = RAW;
|
|
;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_3_BlockQuoteRaw(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_3_BlockQuoteRaw\n"));
|
|
a = cons(mk_str("\n"), a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_BlockQuoteRaw(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_BlockQuoteRaw\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_BlockQuoteRaw(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_BlockQuoteRaw\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_BlockQuote(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_BlockQuote\n"));
|
|
yy = mk_element(BLOCKQUOTE);
|
|
yy->children = a;
|
|
;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_SetextHeading2(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_SetextHeading2\n"));
|
|
yy = mk_list(H2, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_SetextHeading2(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_SetextHeading2\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_SetextHeading1(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_SetextHeading1\n"));
|
|
yy = mk_list(H1, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_SetextHeading1(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_SetextHeading1\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_AtxHeading(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define s ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_AtxHeading\n"));
|
|
yy = mk_list(s->key, a);
|
|
free(s); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
#undef s
|
|
}
|
|
YY_ACTION(void) yy_1_AtxHeading(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define s ctx->val[-2]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_AtxHeading\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
#undef s
|
|
}
|
|
YY_ACTION(void) yy_1_AtxStart(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_AtxStart\n"));
|
|
yy = mk_element(H1 + (strlen(yytext) - 1)); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
}
|
|
YY_ACTION(void) yy_1_Plain(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Plain\n"));
|
|
yy = a; yy->key = PLAIN; ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_Para(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Para\n"));
|
|
yy = a; yy->key = PARA; ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_2_Doc(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_2_Doc\n"));
|
|
parse_result = reverse(a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
YY_ACTION(void) yy_1_Doc(yycontext *ctx, char *yytext, int yyleng)
|
|
{
|
|
#define a ctx->val[-1]
|
|
#define yy ctx->yy
|
|
#define yypos ctx->pos
|
|
#define yythunkpos ctx->thunkpos
|
|
yyprintf((stderr, "do yy_1_Doc\n"));
|
|
a = cons(yy, a); ;
|
|
#undef yythunkpos
|
|
#undef yypos
|
|
#undef yy
|
|
#undef a
|
|
}
|
|
|
|
YY_RULE(int) yy_Notes(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 2, 0);
|
|
yyprintf((stderr, "%s\n", "Notes")); if (!yy_StartList(ctx)) goto l1; yyDo(ctx, yySet, -2, 0);
|
|
l2:;
|
|
{ int yypos3= ctx->pos, yythunkpos3= ctx->thunkpos;
|
|
{ int yypos4= ctx->pos, yythunkpos4= ctx->thunkpos; if (!yy_Note(ctx)) goto l5; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_Notes, ctx->begin, ctx->end); goto l4;
|
|
l5:; ctx->pos= yypos4; ctx->thunkpos= yythunkpos4; if (!yy_SkipBlock(ctx)) goto l3;
|
|
}
|
|
l4:; goto l2;
|
|
l3:; ctx->pos= yypos3; ctx->thunkpos= yythunkpos3;
|
|
} yyDo(ctx, yy_2_Notes, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Notes", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 2, 0);
|
|
return 1;
|
|
l1:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Notes", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_RawNoteBlock(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "RawNoteBlock")); if (!yy_StartList(ctx)) goto l6; yyDo(ctx, yySet, -1, 0);
|
|
{ int yypos9= ctx->pos, yythunkpos9= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l9; goto l6;
|
|
l9:; ctx->pos= yypos9; ctx->thunkpos= yythunkpos9;
|
|
} if (!yy_OptionallyIndentedLine(ctx)) goto l6; yyDo(ctx, yy_1_RawNoteBlock, ctx->begin, ctx->end);
|
|
l7:;
|
|
{ int yypos8= ctx->pos, yythunkpos8= ctx->thunkpos;
|
|
{ int yypos10= ctx->pos, yythunkpos10= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l10; goto l8;
|
|
l10:; ctx->pos= yypos10; ctx->thunkpos= yythunkpos10;
|
|
} if (!yy_OptionallyIndentedLine(ctx)) goto l8; yyDo(ctx, yy_1_RawNoteBlock, ctx->begin, ctx->end); goto l7;
|
|
l8:; ctx->pos= yypos8; ctx->thunkpos= yythunkpos8;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l6;
|
|
l11:;
|
|
{ int yypos12= ctx->pos, yythunkpos12= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l12; goto l11;
|
|
l12:; ctx->pos= yypos12; ctx->thunkpos= yythunkpos12;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l6; yyDo(ctx, yy_2_RawNoteBlock, ctx->begin, ctx->end); yyDo(ctx, yy_3_RawNoteBlock, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "RawNoteBlock", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l6:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "RawNoteBlock", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_RawNoteReference(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "RawNoteReference")); if (!yymatchString(ctx, "[^")) goto l13; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l13;
|
|
{ int yypos16= ctx->pos, yythunkpos16= ctx->thunkpos; if (!yy_Newline(ctx)) goto l16; goto l13;
|
|
l16:; ctx->pos= yypos16; ctx->thunkpos= yythunkpos16;
|
|
}
|
|
{ int yypos17= ctx->pos, yythunkpos17= ctx->thunkpos; if (!yymatchChar(ctx, ']')) goto l17; goto l13;
|
|
l17:; ctx->pos= yypos17; ctx->thunkpos= yythunkpos17;
|
|
} if (!yymatchDot(ctx)) goto l13;
|
|
l14:;
|
|
{ int yypos15= ctx->pos, yythunkpos15= ctx->thunkpos;
|
|
{ int yypos18= ctx->pos, yythunkpos18= ctx->thunkpos; if (!yy_Newline(ctx)) goto l18; goto l15;
|
|
l18:; ctx->pos= yypos18; ctx->thunkpos= yythunkpos18;
|
|
}
|
|
{ int yypos19= ctx->pos, yythunkpos19= ctx->thunkpos; if (!yymatchChar(ctx, ']')) goto l19; goto l15;
|
|
l19:; ctx->pos= yypos19; ctx->thunkpos= yythunkpos19;
|
|
} if (!yymatchDot(ctx)) goto l15; goto l14;
|
|
l15:; ctx->pos= yypos15; ctx->thunkpos= yythunkpos15;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l13; if (!yymatchChar(ctx, ']')) goto l13; yyDo(ctx, yy_1_RawNoteReference, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "RawNoteReference", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l13:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "RawNoteReference", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_DoubleQuoteEnd(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "DoubleQuoteEnd")); if (!yymatchChar(ctx, '"')) goto l20;
|
|
yyprintf((stderr, " ok %s @ %s\n", "DoubleQuoteEnd", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l20:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "DoubleQuoteEnd", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_DoubleQuoteStart(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "DoubleQuoteStart")); if (!yymatchChar(ctx, '"')) goto l21;
|
|
yyprintf((stderr, " ok %s @ %s\n", "DoubleQuoteStart", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l21:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "DoubleQuoteStart", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_SingleQuoteEnd(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "SingleQuoteEnd")); if (!yymatchChar(ctx, '\'')) goto l22;
|
|
{ int yypos23= ctx->pos, yythunkpos23= ctx->thunkpos; if (!yy_Alphanumeric(ctx)) goto l23; goto l22;
|
|
l23:; ctx->pos= yypos23; ctx->thunkpos= yythunkpos23;
|
|
}
|
|
yyprintf((stderr, " ok %s @ %s\n", "SingleQuoteEnd", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l22:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "SingleQuoteEnd", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_SingleQuoteStart(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "SingleQuoteStart")); if (!yymatchChar(ctx, '\'')) goto l24;
|
|
{ int yypos25= ctx->pos, yythunkpos25= ctx->thunkpos;
|
|
{ int yypos26= ctx->pos, yythunkpos26= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l27; goto l26;
|
|
l27:; ctx->pos= yypos26; ctx->thunkpos= yythunkpos26; if (!yy_Newline(ctx)) goto l25;
|
|
}
|
|
l26:; goto l24;
|
|
l25:; ctx->pos= yypos25; ctx->thunkpos= yythunkpos25;
|
|
}
|
|
yyprintf((stderr, " ok %s @ %s\n", "SingleQuoteStart", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l24:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "SingleQuoteStart", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_EnDash(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "EnDash")); if (!yymatchChar(ctx, '-')) goto l28;
|
|
{ int yypos29= ctx->pos, yythunkpos29= ctx->thunkpos; if (!yy_Digit(ctx)) goto l28; ctx->pos= yypos29; ctx->thunkpos= yythunkpos29;
|
|
} yyDo(ctx, yy_1_EnDash, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "EnDash", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l28:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "EnDash", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_EmDash(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "EmDash"));
|
|
{ int yypos31= ctx->pos, yythunkpos31= ctx->thunkpos; if (!yymatchString(ctx, "---")) goto l32; goto l31;
|
|
l32:; ctx->pos= yypos31; ctx->thunkpos= yythunkpos31; if (!yymatchString(ctx, "--")) goto l30;
|
|
}
|
|
l31:; yyDo(ctx, yy_1_EmDash, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "EmDash", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l30:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "EmDash", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Apostrophe(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Apostrophe")); if (!yymatchChar(ctx, '\'')) goto l33; yyDo(ctx, yy_1_Apostrophe, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Apostrophe", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l33:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Apostrophe", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_DoubleQuoted(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 2, 0);
|
|
yyprintf((stderr, "%s\n", "DoubleQuoted")); if (!yy_DoubleQuoteStart(ctx)) goto l34; if (!yy_StartList(ctx)) goto l34; yyDo(ctx, yySet, -2, 0);
|
|
{ int yypos37= ctx->pos, yythunkpos37= ctx->thunkpos; if (!yy_DoubleQuoteEnd(ctx)) goto l37; goto l34;
|
|
l37:; ctx->pos= yypos37; ctx->thunkpos= yythunkpos37;
|
|
} if (!yy_Inline(ctx)) goto l34; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_DoubleQuoted, ctx->begin, ctx->end);
|
|
l35:;
|
|
{ int yypos36= ctx->pos, yythunkpos36= ctx->thunkpos;
|
|
{ int yypos38= ctx->pos, yythunkpos38= ctx->thunkpos; if (!yy_DoubleQuoteEnd(ctx)) goto l38; goto l36;
|
|
l38:; ctx->pos= yypos38; ctx->thunkpos= yythunkpos38;
|
|
} if (!yy_Inline(ctx)) goto l36; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_DoubleQuoted, ctx->begin, ctx->end); goto l35;
|
|
l36:; ctx->pos= yypos36; ctx->thunkpos= yythunkpos36;
|
|
} if (!yy_DoubleQuoteEnd(ctx)) goto l34; yyDo(ctx, yy_2_DoubleQuoted, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "DoubleQuoted", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 2, 0);
|
|
return 1;
|
|
l34:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "DoubleQuoted", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_SingleQuoted(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 2, 0);
|
|
yyprintf((stderr, "%s\n", "SingleQuoted")); if (!yy_SingleQuoteStart(ctx)) goto l39; if (!yy_StartList(ctx)) goto l39; yyDo(ctx, yySet, -2, 0);
|
|
{ int yypos42= ctx->pos, yythunkpos42= ctx->thunkpos; if (!yy_SingleQuoteEnd(ctx)) goto l42; goto l39;
|
|
l42:; ctx->pos= yypos42; ctx->thunkpos= yythunkpos42;
|
|
} if (!yy_Inline(ctx)) goto l39; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_SingleQuoted, ctx->begin, ctx->end);
|
|
l40:;
|
|
{ int yypos41= ctx->pos, yythunkpos41= ctx->thunkpos;
|
|
{ int yypos43= ctx->pos, yythunkpos43= ctx->thunkpos; if (!yy_SingleQuoteEnd(ctx)) goto l43; goto l41;
|
|
l43:; ctx->pos= yypos43; ctx->thunkpos= yythunkpos43;
|
|
} if (!yy_Inline(ctx)) goto l41; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_SingleQuoted, ctx->begin, ctx->end); goto l40;
|
|
l41:; ctx->pos= yypos41; ctx->thunkpos= yythunkpos41;
|
|
} if (!yy_SingleQuoteEnd(ctx)) goto l39; yyDo(ctx, yy_2_SingleQuoted, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "SingleQuoted", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 2, 0);
|
|
return 1;
|
|
l39:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "SingleQuoted", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Dash(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Dash"));
|
|
{ int yypos45= ctx->pos, yythunkpos45= ctx->thunkpos; if (!yy_EmDash(ctx)) goto l46; goto l45;
|
|
l46:; ctx->pos= yypos45; ctx->thunkpos= yythunkpos45; if (!yy_EnDash(ctx)) goto l44;
|
|
}
|
|
l45:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Dash", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l44:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Dash", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Ellipsis(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Ellipsis"));
|
|
{ int yypos48= ctx->pos, yythunkpos48= ctx->thunkpos; if (!yymatchString(ctx, "...")) goto l49; goto l48;
|
|
l49:; ctx->pos= yypos48; ctx->thunkpos= yythunkpos48; if (!yymatchString(ctx, ". . .")) goto l47;
|
|
}
|
|
l48:; yyDo(ctx, yy_1_Ellipsis, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Ellipsis", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l47:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Ellipsis", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Digit(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Digit")); if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\000\000\377\003\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l50;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Digit", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l50:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Digit", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_ExtendedSpecialChar(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "ExtendedSpecialChar"));
|
|
{ int yypos52= ctx->pos, yythunkpos52= ctx->thunkpos; yyText(ctx, ctx->begin, ctx->end); if (!( extension(EXT_SMART) )) goto l53;
|
|
{ int yypos54= ctx->pos, yythunkpos54= ctx->thunkpos; if (!yymatchChar(ctx, '.')) goto l55; goto l54;
|
|
l55:; ctx->pos= yypos54; ctx->thunkpos= yythunkpos54; if (!yymatchChar(ctx, '-')) goto l56; goto l54;
|
|
l56:; ctx->pos= yypos54; ctx->thunkpos= yythunkpos54; if (!yymatchChar(ctx, '\'')) goto l57; goto l54;
|
|
l57:; ctx->pos= yypos54; ctx->thunkpos= yythunkpos54; if (!yymatchChar(ctx, '"')) goto l53;
|
|
}
|
|
l54:; goto l52;
|
|
l53:; ctx->pos= yypos52; ctx->thunkpos= yythunkpos52; yyText(ctx, ctx->begin, ctx->end); if (!( extension(EXT_NOTES) )) goto l51; if (!yymatchChar(ctx, '^')) goto l51;
|
|
}
|
|
l52:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "ExtendedSpecialChar", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l51:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "ExtendedSpecialChar", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_AlphanumericAscii(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "AlphanumericAscii")); if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\000\000\377\003\376\377\377\007\376\377\377\007\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l58;
|
|
yyprintf((stderr, " ok %s @ %s\n", "AlphanumericAscii", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l58:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "AlphanumericAscii", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Quoted(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Quoted"));
|
|
{ int yypos60= ctx->pos, yythunkpos60= ctx->thunkpos; if (!yymatchChar(ctx, '"')) goto l61;
|
|
l62:;
|
|
{ int yypos63= ctx->pos, yythunkpos63= ctx->thunkpos;
|
|
{ int yypos64= ctx->pos, yythunkpos64= ctx->thunkpos; if (!yymatchChar(ctx, '"')) goto l64; goto l63;
|
|
l64:; ctx->pos= yypos64; ctx->thunkpos= yythunkpos64;
|
|
} if (!yymatchDot(ctx)) goto l63; goto l62;
|
|
l63:; ctx->pos= yypos63; ctx->thunkpos= yythunkpos63;
|
|
} if (!yymatchChar(ctx, '"')) goto l61; goto l60;
|
|
l61:; ctx->pos= yypos60; ctx->thunkpos= yythunkpos60; if (!yymatchChar(ctx, '\'')) goto l59;
|
|
l65:;
|
|
{ int yypos66= ctx->pos, yythunkpos66= ctx->thunkpos;
|
|
{ int yypos67= ctx->pos, yythunkpos67= ctx->thunkpos; if (!yymatchChar(ctx, '\'')) goto l67; goto l66;
|
|
l67:; ctx->pos= yypos67; ctx->thunkpos= yythunkpos67;
|
|
} if (!yymatchDot(ctx)) goto l66; goto l65;
|
|
l66:; ctx->pos= yypos66; ctx->thunkpos= yythunkpos66;
|
|
} if (!yymatchChar(ctx, '\'')) goto l59;
|
|
}
|
|
l60:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Quoted", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l59:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Quoted", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlTag(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlTag")); if (!yymatchChar(ctx, '<')) goto l68; if (!yy_Spnl(ctx)) goto l68;
|
|
{ int yypos69= ctx->pos, yythunkpos69= ctx->thunkpos; if (!yymatchChar(ctx, '/')) goto l69; goto l70;
|
|
l69:; ctx->pos= yypos69; ctx->thunkpos= yythunkpos69;
|
|
}
|
|
l70:; if (!yy_AlphanumericAscii(ctx)) goto l68;
|
|
l71:;
|
|
{ int yypos72= ctx->pos, yythunkpos72= ctx->thunkpos; if (!yy_AlphanumericAscii(ctx)) goto l72; goto l71;
|
|
l72:; ctx->pos= yypos72; ctx->thunkpos= yythunkpos72;
|
|
} if (!yy_Spnl(ctx)) goto l68;
|
|
l73:;
|
|
{ int yypos74= ctx->pos, yythunkpos74= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l74; goto l73;
|
|
l74:; ctx->pos= yypos74; ctx->thunkpos= yythunkpos74;
|
|
}
|
|
{ int yypos75= ctx->pos, yythunkpos75= ctx->thunkpos; if (!yymatchChar(ctx, '/')) goto l75; goto l76;
|
|
l75:; ctx->pos= yypos75; ctx->thunkpos= yythunkpos75;
|
|
}
|
|
l76:; if (!yy_Spnl(ctx)) goto l68; if (!yymatchChar(ctx, '>')) goto l68;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlTag", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l68:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlTag", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Ticks5(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Ticks5")); if (!yymatchString(ctx, "`````")) goto l77;
|
|
{ int yypos78= ctx->pos, yythunkpos78= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l78; goto l77;
|
|
l78:; ctx->pos= yypos78; ctx->thunkpos= yythunkpos78;
|
|
}
|
|
yyprintf((stderr, " ok %s @ %s\n", "Ticks5", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l77:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Ticks5", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Ticks4(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Ticks4")); if (!yymatchString(ctx, "````")) goto l79;
|
|
{ int yypos80= ctx->pos, yythunkpos80= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l80; goto l79;
|
|
l80:; ctx->pos= yypos80; ctx->thunkpos= yythunkpos80;
|
|
}
|
|
yyprintf((stderr, " ok %s @ %s\n", "Ticks4", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l79:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Ticks4", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Ticks3(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Ticks3")); if (!yymatchString(ctx, "```")) goto l81;
|
|
{ int yypos82= ctx->pos, yythunkpos82= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l82; goto l81;
|
|
l82:; ctx->pos= yypos82; ctx->thunkpos= yythunkpos82;
|
|
}
|
|
yyprintf((stderr, " ok %s @ %s\n", "Ticks3", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l81:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Ticks3", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Ticks2(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Ticks2")); if (!yymatchString(ctx, "``")) goto l83;
|
|
{ int yypos84= ctx->pos, yythunkpos84= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l84; goto l83;
|
|
l84:; ctx->pos= yypos84; ctx->thunkpos= yythunkpos84;
|
|
}
|
|
yyprintf((stderr, " ok %s @ %s\n", "Ticks2", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l83:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Ticks2", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Ticks1(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Ticks1")); if (!yymatchChar(ctx, '`')) goto l85;
|
|
{ int yypos86= ctx->pos, yythunkpos86= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l86; goto l85;
|
|
l86:; ctx->pos= yypos86; ctx->thunkpos= yythunkpos86;
|
|
}
|
|
yyprintf((stderr, " ok %s @ %s\n", "Ticks1", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l85:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Ticks1", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_SkipBlock(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "SkipBlock"));
|
|
{ int yypos88= ctx->pos, yythunkpos88= ctx->thunkpos; if (!yy_HtmlBlock(ctx)) goto l89; goto l88;
|
|
l89:; ctx->pos= yypos88; ctx->thunkpos= yythunkpos88;
|
|
{ int yypos93= ctx->pos, yythunkpos93= ctx->thunkpos; if (!yymatchChar(ctx, '#')) goto l93; goto l90;
|
|
l93:; ctx->pos= yypos93; ctx->thunkpos= yythunkpos93;
|
|
}
|
|
{ int yypos94= ctx->pos, yythunkpos94= ctx->thunkpos; if (!yy_SetextBottom1(ctx)) goto l94; goto l90;
|
|
l94:; ctx->pos= yypos94; ctx->thunkpos= yythunkpos94;
|
|
}
|
|
{ int yypos95= ctx->pos, yythunkpos95= ctx->thunkpos; if (!yy_SetextBottom2(ctx)) goto l95; goto l90;
|
|
l95:; ctx->pos= yypos95; ctx->thunkpos= yythunkpos95;
|
|
}
|
|
{ int yypos96= ctx->pos, yythunkpos96= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l96; goto l90;
|
|
l96:; ctx->pos= yypos96; ctx->thunkpos= yythunkpos96;
|
|
} if (!yy_RawLine(ctx)) goto l90;
|
|
l91:;
|
|
{ int yypos92= ctx->pos, yythunkpos92= ctx->thunkpos;
|
|
{ int yypos97= ctx->pos, yythunkpos97= ctx->thunkpos; if (!yymatchChar(ctx, '#')) goto l97; goto l92;
|
|
l97:; ctx->pos= yypos97; ctx->thunkpos= yythunkpos97;
|
|
}
|
|
{ int yypos98= ctx->pos, yythunkpos98= ctx->thunkpos; if (!yy_SetextBottom1(ctx)) goto l98; goto l92;
|
|
l98:; ctx->pos= yypos98; ctx->thunkpos= yythunkpos98;
|
|
}
|
|
{ int yypos99= ctx->pos, yythunkpos99= ctx->thunkpos; if (!yy_SetextBottom2(ctx)) goto l99; goto l92;
|
|
l99:; ctx->pos= yypos99; ctx->thunkpos= yythunkpos99;
|
|
}
|
|
{ int yypos100= ctx->pos, yythunkpos100= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l100; goto l92;
|
|
l100:; ctx->pos= yypos100; ctx->thunkpos= yythunkpos100;
|
|
} if (!yy_RawLine(ctx)) goto l92; goto l91;
|
|
l92:; ctx->pos= yypos92; ctx->thunkpos= yythunkpos92;
|
|
}
|
|
l101:;
|
|
{ int yypos102= ctx->pos, yythunkpos102= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l102; goto l101;
|
|
l102:; ctx->pos= yypos102; ctx->thunkpos= yythunkpos102;
|
|
} goto l88;
|
|
l90:; ctx->pos= yypos88; ctx->thunkpos= yythunkpos88; if (!yy_BlankLine(ctx)) goto l103;
|
|
l104:;
|
|
{ int yypos105= ctx->pos, yythunkpos105= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l105; goto l104;
|
|
l105:; ctx->pos= yypos105; ctx->thunkpos= yythunkpos105;
|
|
} goto l88;
|
|
l103:; ctx->pos= yypos88; ctx->thunkpos= yythunkpos88; if (!yy_RawLine(ctx)) goto l87;
|
|
}
|
|
l88:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "SkipBlock", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l87:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "SkipBlock", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_References(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 2, 0);
|
|
yyprintf((stderr, "%s\n", "References")); if (!yy_StartList(ctx)) goto l106; yyDo(ctx, yySet, -2, 0);
|
|
l107:;
|
|
{ int yypos108= ctx->pos, yythunkpos108= ctx->thunkpos;
|
|
{ int yypos109= ctx->pos, yythunkpos109= ctx->thunkpos; if (!yy_Reference(ctx)) goto l110; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_References, ctx->begin, ctx->end); goto l109;
|
|
l110:; ctx->pos= yypos109; ctx->thunkpos= yythunkpos109; if (!yy_SkipBlock(ctx)) goto l108;
|
|
}
|
|
l109:; goto l107;
|
|
l108:; ctx->pos= yypos108; ctx->thunkpos= yythunkpos108;
|
|
} yyDo(ctx, yy_2_References, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "References", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 2, 0);
|
|
return 1;
|
|
l106:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "References", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_EmptyTitle(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "EmptyTitle")); yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l111; if (!yymatchString(ctx, "")) goto l111; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l111;
|
|
yyprintf((stderr, " ok %s @ %s\n", "EmptyTitle", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l111:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "EmptyTitle", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_RefTitleParens(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "RefTitleParens")); if (!yy_Spnl(ctx)) goto l112; if (!yymatchChar(ctx, '(')) goto l112; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l112;
|
|
l113:;
|
|
{ int yypos114= ctx->pos, yythunkpos114= ctx->thunkpos;
|
|
{ int yypos115= ctx->pos, yythunkpos115= ctx->thunkpos;
|
|
{ int yypos116= ctx->pos, yythunkpos116= ctx->thunkpos; if (!yymatchChar(ctx, ')')) goto l117; if (!yy_Sp(ctx)) goto l117; if (!yy_Newline(ctx)) goto l117; goto l116;
|
|
l117:; ctx->pos= yypos116; ctx->thunkpos= yythunkpos116; if (!yy_Newline(ctx)) goto l115;
|
|
}
|
|
l116:; goto l114;
|
|
l115:; ctx->pos= yypos115; ctx->thunkpos= yythunkpos115;
|
|
} if (!yymatchDot(ctx)) goto l114; goto l113;
|
|
l114:; ctx->pos= yypos114; ctx->thunkpos= yythunkpos114;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l112; if (!yymatchChar(ctx, ')')) goto l112;
|
|
yyprintf((stderr, " ok %s @ %s\n", "RefTitleParens", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l112:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "RefTitleParens", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_RefTitleDouble(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "RefTitleDouble")); if (!yy_Spnl(ctx)) goto l118; if (!yymatchChar(ctx, '"')) goto l118; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l118;
|
|
l119:;
|
|
{ int yypos120= ctx->pos, yythunkpos120= ctx->thunkpos;
|
|
{ int yypos121= ctx->pos, yythunkpos121= ctx->thunkpos;
|
|
{ int yypos122= ctx->pos, yythunkpos122= ctx->thunkpos; if (!yymatchChar(ctx, '"')) goto l123; if (!yy_Sp(ctx)) goto l123; if (!yy_Newline(ctx)) goto l123; goto l122;
|
|
l123:; ctx->pos= yypos122; ctx->thunkpos= yythunkpos122; if (!yy_Newline(ctx)) goto l121;
|
|
}
|
|
l122:; goto l120;
|
|
l121:; ctx->pos= yypos121; ctx->thunkpos= yythunkpos121;
|
|
} if (!yymatchDot(ctx)) goto l120; goto l119;
|
|
l120:; ctx->pos= yypos120; ctx->thunkpos= yythunkpos120;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l118; if (!yymatchChar(ctx, '"')) goto l118;
|
|
yyprintf((stderr, " ok %s @ %s\n", "RefTitleDouble", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l118:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "RefTitleDouble", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_RefTitleSingle(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "RefTitleSingle")); if (!yy_Spnl(ctx)) goto l124; if (!yymatchChar(ctx, '\'')) goto l124; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l124;
|
|
l125:;
|
|
{ int yypos126= ctx->pos, yythunkpos126= ctx->thunkpos;
|
|
{ int yypos127= ctx->pos, yythunkpos127= ctx->thunkpos;
|
|
{ int yypos128= ctx->pos, yythunkpos128= ctx->thunkpos; if (!yymatchChar(ctx, '\'')) goto l129; if (!yy_Sp(ctx)) goto l129; if (!yy_Newline(ctx)) goto l129; goto l128;
|
|
l129:; ctx->pos= yypos128; ctx->thunkpos= yythunkpos128; if (!yy_Newline(ctx)) goto l127;
|
|
}
|
|
l128:; goto l126;
|
|
l127:; ctx->pos= yypos127; ctx->thunkpos= yythunkpos127;
|
|
} if (!yymatchDot(ctx)) goto l126; goto l125;
|
|
l126:; ctx->pos= yypos126; ctx->thunkpos= yythunkpos126;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l124; if (!yymatchChar(ctx, '\'')) goto l124;
|
|
yyprintf((stderr, " ok %s @ %s\n", "RefTitleSingle", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l124:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "RefTitleSingle", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_RefTitle(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "RefTitle"));
|
|
{ int yypos131= ctx->pos, yythunkpos131= ctx->thunkpos; if (!yy_RefTitleSingle(ctx)) goto l132; goto l131;
|
|
l132:; ctx->pos= yypos131; ctx->thunkpos= yythunkpos131; if (!yy_RefTitleDouble(ctx)) goto l133; goto l131;
|
|
l133:; ctx->pos= yypos131; ctx->thunkpos= yythunkpos131; if (!yy_RefTitleParens(ctx)) goto l134; goto l131;
|
|
l134:; ctx->pos= yypos131; ctx->thunkpos= yythunkpos131; if (!yy_EmptyTitle(ctx)) goto l130;
|
|
}
|
|
l131:; yyDo(ctx, yy_1_RefTitle, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "RefTitle", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l130:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "RefTitle", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_RefSrc(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "RefSrc")); yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l135; if (!yy_Nonspacechar(ctx)) goto l135;
|
|
l136:;
|
|
{ int yypos137= ctx->pos, yythunkpos137= ctx->thunkpos; if (!yy_Nonspacechar(ctx)) goto l137; goto l136;
|
|
l137:; ctx->pos= yypos137; ctx->thunkpos= yythunkpos137;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l135; yyDo(ctx, yy_1_RefSrc, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "RefSrc", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l135:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "RefSrc", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_AutoLinkEmail(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "AutoLinkEmail")); if (!yymatchChar(ctx, '<')) goto l138;
|
|
{ int yypos139= ctx->pos, yythunkpos139= ctx->thunkpos; if (!yymatchString(ctx, "mailto:")) goto l139; goto l140;
|
|
l139:; ctx->pos= yypos139; ctx->thunkpos= yythunkpos139;
|
|
}
|
|
l140:; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l138; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\062\350\377\003\376\377\377\207\376\377\377\107\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l138;
|
|
l141:;
|
|
{ int yypos142= ctx->pos, yythunkpos142= ctx->thunkpos; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\062\350\377\003\376\377\377\207\376\377\377\107\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l142; goto l141;
|
|
l142:; ctx->pos= yypos142; ctx->thunkpos= yythunkpos142;
|
|
} if (!yymatchChar(ctx, '@')) goto l138;
|
|
{ int yypos145= ctx->pos, yythunkpos145= ctx->thunkpos; if (!yy_Newline(ctx)) goto l145; goto l138;
|
|
l145:; ctx->pos= yypos145; ctx->thunkpos= yythunkpos145;
|
|
}
|
|
{ int yypos146= ctx->pos, yythunkpos146= ctx->thunkpos; if (!yymatchChar(ctx, '>')) goto l146; goto l138;
|
|
l146:; ctx->pos= yypos146; ctx->thunkpos= yythunkpos146;
|
|
} if (!yymatchDot(ctx)) goto l138;
|
|
l143:;
|
|
{ int yypos144= ctx->pos, yythunkpos144= ctx->thunkpos;
|
|
{ int yypos147= ctx->pos, yythunkpos147= ctx->thunkpos; if (!yy_Newline(ctx)) goto l147; goto l144;
|
|
l147:; ctx->pos= yypos147; ctx->thunkpos= yythunkpos147;
|
|
}
|
|
{ int yypos148= ctx->pos, yythunkpos148= ctx->thunkpos; if (!yymatchChar(ctx, '>')) goto l148; goto l144;
|
|
l148:; ctx->pos= yypos148; ctx->thunkpos= yythunkpos148;
|
|
} if (!yymatchDot(ctx)) goto l144; goto l143;
|
|
l144:; ctx->pos= yypos144; ctx->thunkpos= yythunkpos144;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l138; if (!yymatchChar(ctx, '>')) goto l138; yyDo(ctx, yy_1_AutoLinkEmail, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "AutoLinkEmail", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l138:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "AutoLinkEmail", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_AutoLinkUrl(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "AutoLinkUrl")); if (!yymatchChar(ctx, '<')) goto l149; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l149; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\000\000\000\000\376\377\377\007\376\377\377\007\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l149;
|
|
l150:;
|
|
{ int yypos151= ctx->pos, yythunkpos151= ctx->thunkpos; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\000\000\000\000\376\377\377\007\376\377\377\007\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l151; goto l150;
|
|
l151:; ctx->pos= yypos151; ctx->thunkpos= yythunkpos151;
|
|
} if (!yymatchString(ctx, "://")) goto l149;
|
|
{ int yypos154= ctx->pos, yythunkpos154= ctx->thunkpos; if (!yy_Newline(ctx)) goto l154; goto l149;
|
|
l154:; ctx->pos= yypos154; ctx->thunkpos= yythunkpos154;
|
|
}
|
|
{ int yypos155= ctx->pos, yythunkpos155= ctx->thunkpos; if (!yymatchChar(ctx, '>')) goto l155; goto l149;
|
|
l155:; ctx->pos= yypos155; ctx->thunkpos= yythunkpos155;
|
|
} if (!yymatchDot(ctx)) goto l149;
|
|
l152:;
|
|
{ int yypos153= ctx->pos, yythunkpos153= ctx->thunkpos;
|
|
{ int yypos156= ctx->pos, yythunkpos156= ctx->thunkpos; if (!yy_Newline(ctx)) goto l156; goto l153;
|
|
l156:; ctx->pos= yypos156; ctx->thunkpos= yythunkpos156;
|
|
}
|
|
{ int yypos157= ctx->pos, yythunkpos157= ctx->thunkpos; if (!yymatchChar(ctx, '>')) goto l157; goto l153;
|
|
l157:; ctx->pos= yypos157; ctx->thunkpos= yythunkpos157;
|
|
} if (!yymatchDot(ctx)) goto l153; goto l152;
|
|
l153:; ctx->pos= yypos153; ctx->thunkpos= yythunkpos153;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l149; if (!yymatchChar(ctx, '>')) goto l149; yyDo(ctx, yy_1_AutoLinkUrl, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "AutoLinkUrl", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l149:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "AutoLinkUrl", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_TitleDouble(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "TitleDouble")); if (!yymatchChar(ctx, '"')) goto l158; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l158;
|
|
l159:;
|
|
{ int yypos160= ctx->pos, yythunkpos160= ctx->thunkpos;
|
|
{ int yypos161= ctx->pos, yythunkpos161= ctx->thunkpos; if (!yymatchChar(ctx, '"')) goto l161; if (!yy_Sp(ctx)) goto l161;
|
|
{ int yypos162= ctx->pos, yythunkpos162= ctx->thunkpos; if (!yymatchChar(ctx, ')')) goto l163; goto l162;
|
|
l163:; ctx->pos= yypos162; ctx->thunkpos= yythunkpos162; if (!yy_Newline(ctx)) goto l161;
|
|
}
|
|
l162:; goto l160;
|
|
l161:; ctx->pos= yypos161; ctx->thunkpos= yythunkpos161;
|
|
} if (!yymatchDot(ctx)) goto l160; goto l159;
|
|
l160:; ctx->pos= yypos160; ctx->thunkpos= yythunkpos160;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l158; if (!yymatchChar(ctx, '"')) goto l158;
|
|
yyprintf((stderr, " ok %s @ %s\n", "TitleDouble", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l158:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "TitleDouble", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_TitleSingle(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "TitleSingle")); if (!yymatchChar(ctx, '\'')) goto l164; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l164;
|
|
l165:;
|
|
{ int yypos166= ctx->pos, yythunkpos166= ctx->thunkpos;
|
|
{ int yypos167= ctx->pos, yythunkpos167= ctx->thunkpos; if (!yymatchChar(ctx, '\'')) goto l167; if (!yy_Sp(ctx)) goto l167;
|
|
{ int yypos168= ctx->pos, yythunkpos168= ctx->thunkpos; if (!yymatchChar(ctx, ')')) goto l169; goto l168;
|
|
l169:; ctx->pos= yypos168; ctx->thunkpos= yythunkpos168; if (!yy_Newline(ctx)) goto l167;
|
|
}
|
|
l168:; goto l166;
|
|
l167:; ctx->pos= yypos167; ctx->thunkpos= yythunkpos167;
|
|
} if (!yymatchDot(ctx)) goto l166; goto l165;
|
|
l166:; ctx->pos= yypos166; ctx->thunkpos= yythunkpos166;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l164; if (!yymatchChar(ctx, '\'')) goto l164;
|
|
yyprintf((stderr, " ok %s @ %s\n", "TitleSingle", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l164:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "TitleSingle", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Nonspacechar(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Nonspacechar"));
|
|
{ int yypos171= ctx->pos, yythunkpos171= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l171; goto l170;
|
|
l171:; ctx->pos= yypos171; ctx->thunkpos= yythunkpos171;
|
|
}
|
|
{ int yypos172= ctx->pos, yythunkpos172= ctx->thunkpos; if (!yy_Newline(ctx)) goto l172; goto l170;
|
|
l172:; ctx->pos= yypos172; ctx->thunkpos= yythunkpos172;
|
|
} if (!yymatchDot(ctx)) goto l170;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Nonspacechar", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l170:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Nonspacechar", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_SourceContents(yycontext *ctx)
|
|
{
|
|
yyprintf((stderr, "%s\n", "SourceContents"));
|
|
l174:;
|
|
{ int yypos175= ctx->pos, yythunkpos175= ctx->thunkpos;
|
|
{ int yypos176= ctx->pos, yythunkpos176= ctx->thunkpos;
|
|
{ int yypos180= ctx->pos, yythunkpos180= ctx->thunkpos; if (!yymatchChar(ctx, '(')) goto l180; goto l177;
|
|
l180:; ctx->pos= yypos180; ctx->thunkpos= yythunkpos180;
|
|
}
|
|
{ int yypos181= ctx->pos, yythunkpos181= ctx->thunkpos; if (!yymatchChar(ctx, ')')) goto l181; goto l177;
|
|
l181:; ctx->pos= yypos181; ctx->thunkpos= yythunkpos181;
|
|
}
|
|
{ int yypos182= ctx->pos, yythunkpos182= ctx->thunkpos; if (!yymatchChar(ctx, '>')) goto l182; goto l177;
|
|
l182:; ctx->pos= yypos182; ctx->thunkpos= yythunkpos182;
|
|
} if (!yy_Nonspacechar(ctx)) goto l177;
|
|
l178:;
|
|
{ int yypos179= ctx->pos, yythunkpos179= ctx->thunkpos;
|
|
{ int yypos183= ctx->pos, yythunkpos183= ctx->thunkpos; if (!yymatchChar(ctx, '(')) goto l183; goto l179;
|
|
l183:; ctx->pos= yypos183; ctx->thunkpos= yythunkpos183;
|
|
}
|
|
{ int yypos184= ctx->pos, yythunkpos184= ctx->thunkpos; if (!yymatchChar(ctx, ')')) goto l184; goto l179;
|
|
l184:; ctx->pos= yypos184; ctx->thunkpos= yythunkpos184;
|
|
}
|
|
{ int yypos185= ctx->pos, yythunkpos185= ctx->thunkpos; if (!yymatchChar(ctx, '>')) goto l185; goto l179;
|
|
l185:; ctx->pos= yypos185; ctx->thunkpos= yythunkpos185;
|
|
} if (!yy_Nonspacechar(ctx)) goto l179; goto l178;
|
|
l179:; ctx->pos= yypos179; ctx->thunkpos= yythunkpos179;
|
|
} goto l176;
|
|
l177:; ctx->pos= yypos176; ctx->thunkpos= yythunkpos176; if (!yymatchChar(ctx, '(')) goto l175; if (!yy_SourceContents(ctx)) goto l175; if (!yymatchChar(ctx, ')')) goto l175;
|
|
}
|
|
l176:; goto l174;
|
|
l175:; ctx->pos= yypos175; ctx->thunkpos= yythunkpos175;
|
|
}
|
|
yyprintf((stderr, " ok %s @ %s\n", "SourceContents", ctx->buf+ctx->pos));
|
|
return 1;
|
|
}
|
|
YY_RULE(int) yy_Title(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Title"));
|
|
{ int yypos187= ctx->pos, yythunkpos187= ctx->thunkpos; if (!yy_TitleSingle(ctx)) goto l188; goto l187;
|
|
l188:; ctx->pos= yypos187; ctx->thunkpos= yythunkpos187; if (!yy_TitleDouble(ctx)) goto l189; goto l187;
|
|
l189:; ctx->pos= yypos187; ctx->thunkpos= yythunkpos187; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l186; if (!yymatchString(ctx, "")) goto l186; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l186;
|
|
}
|
|
l187:; yyDo(ctx, yy_1_Title, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Title", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l186:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Title", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Source(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Source"));
|
|
{ int yypos191= ctx->pos, yythunkpos191= ctx->thunkpos; if (!yymatchChar(ctx, '<')) goto l192; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l192; if (!yy_SourceContents(ctx)) goto l192; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l192; if (!yymatchChar(ctx, '>')) goto l192; goto l191;
|
|
l192:; ctx->pos= yypos191; ctx->thunkpos= yythunkpos191; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l190; if (!yy_SourceContents(ctx)) goto l190; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l190;
|
|
}
|
|
l191:; yyDo(ctx, yy_1_Source, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Source", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l190:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Source", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Label(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "Label")); if (!yymatchChar(ctx, '[')) goto l193;
|
|
{ int yypos194= ctx->pos, yythunkpos194= ctx->thunkpos;
|
|
{ int yypos196= ctx->pos, yythunkpos196= ctx->thunkpos; if (!yymatchChar(ctx, '^')) goto l196; goto l195;
|
|
l196:; ctx->pos= yypos196; ctx->thunkpos= yythunkpos196;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!( extension(EXT_NOTES) )) goto l195; goto l194;
|
|
l195:; ctx->pos= yypos194; ctx->thunkpos= yythunkpos194;
|
|
{ int yypos197= ctx->pos, yythunkpos197= ctx->thunkpos; if (!yymatchDot(ctx)) goto l193; ctx->pos= yypos197; ctx->thunkpos= yythunkpos197;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!( !extension(EXT_NOTES) )) goto l193;
|
|
}
|
|
l194:; if (!yy_StartList(ctx)) goto l193; yyDo(ctx, yySet, -1, 0);
|
|
l198:;
|
|
{ int yypos199= ctx->pos, yythunkpos199= ctx->thunkpos;
|
|
{ int yypos200= ctx->pos, yythunkpos200= ctx->thunkpos; if (!yymatchChar(ctx, ']')) goto l200; goto l199;
|
|
l200:; ctx->pos= yypos200; ctx->thunkpos= yythunkpos200;
|
|
} if (!yy_Inline(ctx)) goto l199; yyDo(ctx, yy_1_Label, ctx->begin, ctx->end); goto l198;
|
|
l199:; ctx->pos= yypos199; ctx->thunkpos= yythunkpos199;
|
|
} if (!yymatchChar(ctx, ']')) goto l193; yyDo(ctx, yy_2_Label, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Label", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l193:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Label", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_ReferenceLinkSingle(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "ReferenceLinkSingle")); if (!yy_Label(ctx)) goto l201; yyDo(ctx, yySet, -1, 0); yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l201;
|
|
{ int yypos202= ctx->pos, yythunkpos202= ctx->thunkpos; if (!yy_Spnl(ctx)) goto l202; if (!yymatchString(ctx, "[]")) goto l202; goto l203;
|
|
l202:; ctx->pos= yypos202; ctx->thunkpos= yythunkpos202;
|
|
}
|
|
l203:; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l201; yyDo(ctx, yy_1_ReferenceLinkSingle, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "ReferenceLinkSingle", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l201:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "ReferenceLinkSingle", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_ReferenceLinkDouble(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 2, 0);
|
|
yyprintf((stderr, "%s\n", "ReferenceLinkDouble")); if (!yy_Label(ctx)) goto l204; yyDo(ctx, yySet, -2, 0); yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l204; if (!yy_Spnl(ctx)) goto l204; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l204;
|
|
{ int yypos205= ctx->pos, yythunkpos205= ctx->thunkpos; if (!yymatchString(ctx, "[]")) goto l205; goto l204;
|
|
l205:; ctx->pos= yypos205; ctx->thunkpos= yythunkpos205;
|
|
} if (!yy_Label(ctx)) goto l204; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_ReferenceLinkDouble, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "ReferenceLinkDouble", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 2, 0);
|
|
return 1;
|
|
l204:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "ReferenceLinkDouble", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_AutoLink(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "AutoLink"));
|
|
{ int yypos207= ctx->pos, yythunkpos207= ctx->thunkpos; if (!yy_AutoLinkUrl(ctx)) goto l208; goto l207;
|
|
l208:; ctx->pos= yypos207; ctx->thunkpos= yythunkpos207; if (!yy_AutoLinkEmail(ctx)) goto l206;
|
|
}
|
|
l207:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "AutoLink", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l206:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "AutoLink", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_ReferenceLink(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "ReferenceLink"));
|
|
{ int yypos210= ctx->pos, yythunkpos210= ctx->thunkpos; if (!yy_ReferenceLinkDouble(ctx)) goto l211; goto l210;
|
|
l211:; ctx->pos= yypos210; ctx->thunkpos= yythunkpos210; if (!yy_ReferenceLinkSingle(ctx)) goto l209;
|
|
}
|
|
l210:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "ReferenceLink", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l209:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "ReferenceLink", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_ExplicitLink(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 3, 0);
|
|
yyprintf((stderr, "%s\n", "ExplicitLink")); if (!yy_Label(ctx)) goto l212; yyDo(ctx, yySet, -3, 0); if (!yymatchChar(ctx, '(')) goto l212; if (!yy_Sp(ctx)) goto l212; if (!yy_Source(ctx)) goto l212; yyDo(ctx, yySet, -2, 0); if (!yy_Spnl(ctx)) goto l212; if (!yy_Title(ctx)) goto l212; yyDo(ctx, yySet, -1, 0); if (!yy_Sp(ctx)) goto l212; if (!yymatchChar(ctx, ')')) goto l212; yyDo(ctx, yy_1_ExplicitLink, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "ExplicitLink", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 3, 0);
|
|
return 1;
|
|
l212:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "ExplicitLink", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_StrongUl(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 2, 0);
|
|
yyprintf((stderr, "%s\n", "StrongUl")); if (!yymatchString(ctx, "__")) goto l213;
|
|
{ int yypos214= ctx->pos, yythunkpos214= ctx->thunkpos; if (!yy_Whitespace(ctx)) goto l214; goto l213;
|
|
l214:; ctx->pos= yypos214; ctx->thunkpos= yythunkpos214;
|
|
} if (!yy_StartList(ctx)) goto l213; yyDo(ctx, yySet, -2, 0);
|
|
{ int yypos217= ctx->pos, yythunkpos217= ctx->thunkpos; if (!yymatchString(ctx, "__")) goto l217; goto l213;
|
|
l217:; ctx->pos= yypos217; ctx->thunkpos= yythunkpos217;
|
|
} if (!yy_Inline(ctx)) goto l213; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_StrongUl, ctx->begin, ctx->end);
|
|
l215:;
|
|
{ int yypos216= ctx->pos, yythunkpos216= ctx->thunkpos;
|
|
{ int yypos218= ctx->pos, yythunkpos218= ctx->thunkpos; if (!yymatchString(ctx, "__")) goto l218; goto l216;
|
|
l218:; ctx->pos= yypos218; ctx->thunkpos= yythunkpos218;
|
|
} if (!yy_Inline(ctx)) goto l216; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_StrongUl, ctx->begin, ctx->end); goto l215;
|
|
l216:; ctx->pos= yypos216; ctx->thunkpos= yythunkpos216;
|
|
} if (!yymatchString(ctx, "__")) goto l213; yyDo(ctx, yy_2_StrongUl, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "StrongUl", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 2, 0);
|
|
return 1;
|
|
l213:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "StrongUl", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_StrongStar(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 2, 0);
|
|
yyprintf((stderr, "%s\n", "StrongStar")); if (!yymatchString(ctx, "**")) goto l219;
|
|
{ int yypos220= ctx->pos, yythunkpos220= ctx->thunkpos; if (!yy_Whitespace(ctx)) goto l220; goto l219;
|
|
l220:; ctx->pos= yypos220; ctx->thunkpos= yythunkpos220;
|
|
} if (!yy_StartList(ctx)) goto l219; yyDo(ctx, yySet, -2, 0);
|
|
{ int yypos223= ctx->pos, yythunkpos223= ctx->thunkpos; if (!yymatchString(ctx, "**")) goto l223; goto l219;
|
|
l223:; ctx->pos= yypos223; ctx->thunkpos= yythunkpos223;
|
|
} if (!yy_Inline(ctx)) goto l219; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_StrongStar, ctx->begin, ctx->end);
|
|
l221:;
|
|
{ int yypos222= ctx->pos, yythunkpos222= ctx->thunkpos;
|
|
{ int yypos224= ctx->pos, yythunkpos224= ctx->thunkpos; if (!yymatchString(ctx, "**")) goto l224; goto l222;
|
|
l224:; ctx->pos= yypos224; ctx->thunkpos= yythunkpos224;
|
|
} if (!yy_Inline(ctx)) goto l222; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_StrongStar, ctx->begin, ctx->end); goto l221;
|
|
l222:; ctx->pos= yypos222; ctx->thunkpos= yythunkpos222;
|
|
} if (!yymatchString(ctx, "**")) goto l219; yyDo(ctx, yy_2_StrongStar, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "StrongStar", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 2, 0);
|
|
return 1;
|
|
l219:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "StrongStar", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Whitespace(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Whitespace"));
|
|
{ int yypos226= ctx->pos, yythunkpos226= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l227; goto l226;
|
|
l227:; ctx->pos= yypos226; ctx->thunkpos= yythunkpos226; if (!yy_Newline(ctx)) goto l225;
|
|
}
|
|
l226:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Whitespace", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l225:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Whitespace", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_EmphUl(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 2, 0);
|
|
yyprintf((stderr, "%s\n", "EmphUl")); if (!yymatchChar(ctx, '_')) goto l228;
|
|
{ int yypos229= ctx->pos, yythunkpos229= ctx->thunkpos; if (!yy_Whitespace(ctx)) goto l229; goto l228;
|
|
l229:; ctx->pos= yypos229; ctx->thunkpos= yythunkpos229;
|
|
} if (!yy_StartList(ctx)) goto l228; yyDo(ctx, yySet, -2, 0);
|
|
{ int yypos232= ctx->pos, yythunkpos232= ctx->thunkpos;
|
|
{ int yypos234= ctx->pos, yythunkpos234= ctx->thunkpos; if (!yymatchChar(ctx, '_')) goto l234; goto l233;
|
|
l234:; ctx->pos= yypos234; ctx->thunkpos= yythunkpos234;
|
|
} if (!yy_Inline(ctx)) goto l233; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_EmphUl, ctx->begin, ctx->end); goto l232;
|
|
l233:; ctx->pos= yypos232; ctx->thunkpos= yythunkpos232; if (!yy_StrongUl(ctx)) goto l228; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_2_EmphUl, ctx->begin, ctx->end);
|
|
}
|
|
l232:;
|
|
l230:;
|
|
{ int yypos231= ctx->pos, yythunkpos231= ctx->thunkpos;
|
|
{ int yypos235= ctx->pos, yythunkpos235= ctx->thunkpos;
|
|
{ int yypos237= ctx->pos, yythunkpos237= ctx->thunkpos; if (!yymatchChar(ctx, '_')) goto l237; goto l236;
|
|
l237:; ctx->pos= yypos237; ctx->thunkpos= yythunkpos237;
|
|
} if (!yy_Inline(ctx)) goto l236; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_EmphUl, ctx->begin, ctx->end); goto l235;
|
|
l236:; ctx->pos= yypos235; ctx->thunkpos= yythunkpos235; if (!yy_StrongUl(ctx)) goto l231; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_2_EmphUl, ctx->begin, ctx->end);
|
|
}
|
|
l235:; goto l230;
|
|
l231:; ctx->pos= yypos231; ctx->thunkpos= yythunkpos231;
|
|
} if (!yymatchChar(ctx, '_')) goto l228; yyDo(ctx, yy_3_EmphUl, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "EmphUl", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 2, 0);
|
|
return 1;
|
|
l228:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "EmphUl", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_EmphStar(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 2, 0);
|
|
yyprintf((stderr, "%s\n", "EmphStar")); if (!yymatchChar(ctx, '*')) goto l238;
|
|
{ int yypos239= ctx->pos, yythunkpos239= ctx->thunkpos; if (!yy_Whitespace(ctx)) goto l239; goto l238;
|
|
l239:; ctx->pos= yypos239; ctx->thunkpos= yythunkpos239;
|
|
} if (!yy_StartList(ctx)) goto l238; yyDo(ctx, yySet, -2, 0);
|
|
{ int yypos242= ctx->pos, yythunkpos242= ctx->thunkpos;
|
|
{ int yypos244= ctx->pos, yythunkpos244= ctx->thunkpos; if (!yymatchChar(ctx, '*')) goto l244; goto l243;
|
|
l244:; ctx->pos= yypos244; ctx->thunkpos= yythunkpos244;
|
|
} if (!yy_Inline(ctx)) goto l243; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_EmphStar, ctx->begin, ctx->end); goto l242;
|
|
l243:; ctx->pos= yypos242; ctx->thunkpos= yythunkpos242; if (!yy_StrongStar(ctx)) goto l238; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_2_EmphStar, ctx->begin, ctx->end);
|
|
}
|
|
l242:;
|
|
l240:;
|
|
{ int yypos241= ctx->pos, yythunkpos241= ctx->thunkpos;
|
|
{ int yypos245= ctx->pos, yythunkpos245= ctx->thunkpos;
|
|
{ int yypos247= ctx->pos, yythunkpos247= ctx->thunkpos; if (!yymatchChar(ctx, '*')) goto l247; goto l246;
|
|
l247:; ctx->pos= yypos247; ctx->thunkpos= yythunkpos247;
|
|
} if (!yy_Inline(ctx)) goto l246; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_EmphStar, ctx->begin, ctx->end); goto l245;
|
|
l246:; ctx->pos= yypos245; ctx->thunkpos= yythunkpos245; if (!yy_StrongStar(ctx)) goto l241; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_2_EmphStar, ctx->begin, ctx->end);
|
|
}
|
|
l245:; goto l240;
|
|
l241:; ctx->pos= yypos241; ctx->thunkpos= yythunkpos241;
|
|
} if (!yymatchChar(ctx, '*')) goto l238; yyDo(ctx, yy_3_EmphStar, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "EmphStar", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 2, 0);
|
|
return 1;
|
|
l238:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "EmphStar", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_StarLine(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "StarLine"));
|
|
{ int yypos249= ctx->pos, yythunkpos249= ctx->thunkpos; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l250; if (!yymatchString(ctx, "****")) goto l250;
|
|
l251:;
|
|
{ int yypos252= ctx->pos, yythunkpos252= ctx->thunkpos; if (!yymatchChar(ctx, '*')) goto l252; goto l251;
|
|
l252:; ctx->pos= yypos252; ctx->thunkpos= yythunkpos252;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l250; goto l249;
|
|
l250:; ctx->pos= yypos249; ctx->thunkpos= yythunkpos249; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l248; if (!yy_Spacechar(ctx)) goto l248; if (!yymatchChar(ctx, '*')) goto l248;
|
|
l253:;
|
|
{ int yypos254= ctx->pos, yythunkpos254= ctx->thunkpos; if (!yymatchChar(ctx, '*')) goto l254; goto l253;
|
|
l254:; ctx->pos= yypos254; ctx->thunkpos= yythunkpos254;
|
|
}
|
|
{ int yypos255= ctx->pos, yythunkpos255= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l248; ctx->pos= yypos255; ctx->thunkpos= yythunkpos255;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l248;
|
|
}
|
|
l249:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "StarLine", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l248:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "StarLine", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_UlLine(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "UlLine"));
|
|
{ int yypos257= ctx->pos, yythunkpos257= ctx->thunkpos; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l258; if (!yymatchString(ctx, "____")) goto l258;
|
|
l259:;
|
|
{ int yypos260= ctx->pos, yythunkpos260= ctx->thunkpos; if (!yymatchChar(ctx, '_')) goto l260; goto l259;
|
|
l260:; ctx->pos= yypos260; ctx->thunkpos= yythunkpos260;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l258; goto l257;
|
|
l258:; ctx->pos= yypos257; ctx->thunkpos= yythunkpos257; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l256; if (!yy_Spacechar(ctx)) goto l256; if (!yymatchChar(ctx, '_')) goto l256;
|
|
l261:;
|
|
{ int yypos262= ctx->pos, yythunkpos262= ctx->thunkpos; if (!yymatchChar(ctx, '_')) goto l262; goto l261;
|
|
l262:; ctx->pos= yypos262; ctx->thunkpos= yythunkpos262;
|
|
}
|
|
{ int yypos263= ctx->pos, yythunkpos263= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l256; ctx->pos= yypos263; ctx->thunkpos= yythunkpos263;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l256;
|
|
}
|
|
l257:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "UlLine", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l256:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "UlLine", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_SpecialChar(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "SpecialChar"));
|
|
{ int yypos265= ctx->pos, yythunkpos265= ctx->thunkpos; if (!yymatchChar(ctx, '*')) goto l266; goto l265;
|
|
l266:; ctx->pos= yypos265; ctx->thunkpos= yythunkpos265; if (!yymatchChar(ctx, '_')) goto l267; goto l265;
|
|
l267:; ctx->pos= yypos265; ctx->thunkpos= yythunkpos265; if (!yymatchChar(ctx, '`')) goto l268; goto l265;
|
|
l268:; ctx->pos= yypos265; ctx->thunkpos= yythunkpos265; if (!yymatchChar(ctx, '&')) goto l269; goto l265;
|
|
l269:; ctx->pos= yypos265; ctx->thunkpos= yythunkpos265; if (!yymatchChar(ctx, '[')) goto l270; goto l265;
|
|
l270:; ctx->pos= yypos265; ctx->thunkpos= yythunkpos265; if (!yymatchChar(ctx, ']')) goto l271; goto l265;
|
|
l271:; ctx->pos= yypos265; ctx->thunkpos= yythunkpos265; if (!yymatchChar(ctx, '(')) goto l272; goto l265;
|
|
l272:; ctx->pos= yypos265; ctx->thunkpos= yythunkpos265; if (!yymatchChar(ctx, ')')) goto l273; goto l265;
|
|
l273:; ctx->pos= yypos265; ctx->thunkpos= yythunkpos265; if (!yymatchChar(ctx, '<')) goto l274; goto l265;
|
|
l274:; ctx->pos= yypos265; ctx->thunkpos= yythunkpos265; if (!yymatchChar(ctx, '!')) goto l275; goto l265;
|
|
l275:; ctx->pos= yypos265; ctx->thunkpos= yythunkpos265; if (!yymatchChar(ctx, '#')) goto l276; goto l265;
|
|
l276:; ctx->pos= yypos265; ctx->thunkpos= yythunkpos265; if (!yymatchChar(ctx, '\\')) goto l277; goto l265;
|
|
l277:; ctx->pos= yypos265; ctx->thunkpos= yythunkpos265; if (!yymatchChar(ctx, '\'')) goto l278; goto l265;
|
|
l278:; ctx->pos= yypos265; ctx->thunkpos= yythunkpos265; if (!yymatchChar(ctx, '"')) goto l279; goto l265;
|
|
l279:; ctx->pos= yypos265; ctx->thunkpos= yythunkpos265; if (!yy_ExtendedSpecialChar(ctx)) goto l264;
|
|
}
|
|
l265:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "SpecialChar", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l264:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "SpecialChar", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Eof(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Eof"));
|
|
{ int yypos281= ctx->pos, yythunkpos281= ctx->thunkpos; if (!yymatchDot(ctx)) goto l281; goto l280;
|
|
l281:; ctx->pos= yypos281; ctx->thunkpos= yythunkpos281;
|
|
}
|
|
yyprintf((stderr, " ok %s @ %s\n", "Eof", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l280:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Eof", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_NormalEndline(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "NormalEndline")); if (!yy_Sp(ctx)) goto l282; if (!yy_Newline(ctx)) goto l282;
|
|
{ int yypos283= ctx->pos, yythunkpos283= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l283; goto l282;
|
|
l283:; ctx->pos= yypos283; ctx->thunkpos= yythunkpos283;
|
|
}
|
|
{ int yypos284= ctx->pos, yythunkpos284= ctx->thunkpos; if (!yymatchChar(ctx, '>')) goto l284; goto l282;
|
|
l284:; ctx->pos= yypos284; ctx->thunkpos= yythunkpos284;
|
|
}
|
|
{ int yypos285= ctx->pos, yythunkpos285= ctx->thunkpos; if (!yy_AtxStart(ctx)) goto l285; goto l282;
|
|
l285:; ctx->pos= yypos285; ctx->thunkpos= yythunkpos285;
|
|
}
|
|
{ int yypos286= ctx->pos, yythunkpos286= ctx->thunkpos; if (!yy_Line(ctx)) goto l286;
|
|
{ int yypos287= ctx->pos, yythunkpos287= ctx->thunkpos; if (!yymatchChar(ctx, '=')) goto l288;
|
|
l289:;
|
|
{ int yypos290= ctx->pos, yythunkpos290= ctx->thunkpos; if (!yymatchChar(ctx, '=')) goto l290; goto l289;
|
|
l290:; ctx->pos= yypos290; ctx->thunkpos= yythunkpos290;
|
|
} goto l287;
|
|
l288:; ctx->pos= yypos287; ctx->thunkpos= yythunkpos287; if (!yymatchChar(ctx, '-')) goto l286;
|
|
l291:;
|
|
{ int yypos292= ctx->pos, yythunkpos292= ctx->thunkpos; if (!yymatchChar(ctx, '-')) goto l292; goto l291;
|
|
l292:; ctx->pos= yypos292; ctx->thunkpos= yythunkpos292;
|
|
}
|
|
}
|
|
l287:; if (!yy_Newline(ctx)) goto l286; goto l282;
|
|
l286:; ctx->pos= yypos286; ctx->thunkpos= yythunkpos286;
|
|
} yyDo(ctx, yy_1_NormalEndline, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "NormalEndline", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l282:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "NormalEndline", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_TerminalEndline(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "TerminalEndline")); if (!yy_Sp(ctx)) goto l293; if (!yy_Newline(ctx)) goto l293; if (!yy_Eof(ctx)) goto l293; yyDo(ctx, yy_1_TerminalEndline, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "TerminalEndline", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l293:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "TerminalEndline", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_LineBreak(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "LineBreak")); if (!yymatchString(ctx, " ")) goto l294; if (!yy_NormalEndline(ctx)) goto l294; yyDo(ctx, yy_1_LineBreak, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "LineBreak", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l294:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "LineBreak", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_CharEntity(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "CharEntity")); yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l295; if (!yymatchChar(ctx, '&')) goto l295; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\000\000\377\003\376\377\377\007\376\377\377\007\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l295;
|
|
l296:;
|
|
{ int yypos297= ctx->pos, yythunkpos297= ctx->thunkpos; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\000\000\377\003\376\377\377\007\376\377\377\007\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l297; goto l296;
|
|
l297:; ctx->pos= yypos297; ctx->thunkpos= yythunkpos297;
|
|
} if (!yymatchChar(ctx, ';')) goto l295; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l295;
|
|
yyprintf((stderr, " ok %s @ %s\n", "CharEntity", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l295:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "CharEntity", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_DecEntity(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "DecEntity")); yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l298; if (!yymatchChar(ctx, '&')) goto l298; if (!yymatchChar(ctx, '#')) goto l298; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\000\000\377\003\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l298;
|
|
l299:;
|
|
{ int yypos300= ctx->pos, yythunkpos300= ctx->thunkpos; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\000\000\377\003\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l300; goto l299;
|
|
l300:; ctx->pos= yypos300; ctx->thunkpos= yythunkpos300;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l298; if (!yymatchChar(ctx, ';')) goto l298; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l298;
|
|
yyprintf((stderr, " ok %s @ %s\n", "DecEntity", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l298:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "DecEntity", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HexEntity(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HexEntity")); yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l301; if (!yymatchChar(ctx, '&')) goto l301; if (!yymatchChar(ctx, '#')) goto l301; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\000\000\000\000\000\000\000\001\000\000\000\001\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l301; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\000\000\377\003\176\000\000\000\176\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l301;
|
|
l302:;
|
|
{ int yypos303= ctx->pos, yythunkpos303= ctx->thunkpos; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\000\000\377\003\176\000\000\000\176\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l303; goto l302;
|
|
l303:; ctx->pos= yypos303; ctx->thunkpos= yythunkpos303;
|
|
} if (!yymatchChar(ctx, ';')) goto l301; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l301;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HexEntity", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l301:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HexEntity", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_AposChunk(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "AposChunk")); yyText(ctx, ctx->begin, ctx->end); if (!( extension(EXT_SMART) )) goto l304; if (!yymatchChar(ctx, '\'')) goto l304;
|
|
{ int yypos305= ctx->pos, yythunkpos305= ctx->thunkpos; if (!yy_Alphanumeric(ctx)) goto l304; ctx->pos= yypos305; ctx->thunkpos= yythunkpos305;
|
|
} yyDo(ctx, yy_1_AposChunk, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "AposChunk", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l304:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "AposChunk", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Alphanumeric(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Alphanumeric"));
|
|
{ int yypos307= ctx->pos, yythunkpos307= ctx->thunkpos; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\000\000\377\003\376\377\377\007\376\377\377\007\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l308; goto l307;
|
|
l308:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\200")) goto l309; goto l307;
|
|
l309:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\201")) goto l310; goto l307;
|
|
l310:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\202")) goto l311; goto l307;
|
|
l311:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\203")) goto l312; goto l307;
|
|
l312:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\204")) goto l313; goto l307;
|
|
l313:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\205")) goto l314; goto l307;
|
|
l314:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\206")) goto l315; goto l307;
|
|
l315:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\207")) goto l316; goto l307;
|
|
l316:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\210")) goto l317; goto l307;
|
|
l317:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\211")) goto l318; goto l307;
|
|
l318:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\212")) goto l319; goto l307;
|
|
l319:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\213")) goto l320; goto l307;
|
|
l320:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\214")) goto l321; goto l307;
|
|
l321:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\215")) goto l322; goto l307;
|
|
l322:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\216")) goto l323; goto l307;
|
|
l323:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\217")) goto l324; goto l307;
|
|
l324:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\220")) goto l325; goto l307;
|
|
l325:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\221")) goto l326; goto l307;
|
|
l326:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\222")) goto l327; goto l307;
|
|
l327:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\223")) goto l328; goto l307;
|
|
l328:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\224")) goto l329; goto l307;
|
|
l329:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\225")) goto l330; goto l307;
|
|
l330:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\226")) goto l331; goto l307;
|
|
l331:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\227")) goto l332; goto l307;
|
|
l332:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\230")) goto l333; goto l307;
|
|
l333:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\231")) goto l334; goto l307;
|
|
l334:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\232")) goto l335; goto l307;
|
|
l335:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\233")) goto l336; goto l307;
|
|
l336:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\234")) goto l337; goto l307;
|
|
l337:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\235")) goto l338; goto l307;
|
|
l338:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\236")) goto l339; goto l307;
|
|
l339:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\237")) goto l340; goto l307;
|
|
l340:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\240")) goto l341; goto l307;
|
|
l341:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\241")) goto l342; goto l307;
|
|
l342:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\242")) goto l343; goto l307;
|
|
l343:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\243")) goto l344; goto l307;
|
|
l344:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\244")) goto l345; goto l307;
|
|
l345:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\245")) goto l346; goto l307;
|
|
l346:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\246")) goto l347; goto l307;
|
|
l347:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\247")) goto l348; goto l307;
|
|
l348:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\250")) goto l349; goto l307;
|
|
l349:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\251")) goto l350; goto l307;
|
|
l350:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\252")) goto l351; goto l307;
|
|
l351:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\253")) goto l352; goto l307;
|
|
l352:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\254")) goto l353; goto l307;
|
|
l353:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\255")) goto l354; goto l307;
|
|
l354:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\256")) goto l355; goto l307;
|
|
l355:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\257")) goto l356; goto l307;
|
|
l356:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\260")) goto l357; goto l307;
|
|
l357:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\261")) goto l358; goto l307;
|
|
l358:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\262")) goto l359; goto l307;
|
|
l359:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\263")) goto l360; goto l307;
|
|
l360:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\264")) goto l361; goto l307;
|
|
l361:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\265")) goto l362; goto l307;
|
|
l362:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\266")) goto l363; goto l307;
|
|
l363:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\267")) goto l364; goto l307;
|
|
l364:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\270")) goto l365; goto l307;
|
|
l365:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\271")) goto l366; goto l307;
|
|
l366:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\272")) goto l367; goto l307;
|
|
l367:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\273")) goto l368; goto l307;
|
|
l368:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\274")) goto l369; goto l307;
|
|
l369:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\275")) goto l370; goto l307;
|
|
l370:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\276")) goto l371; goto l307;
|
|
l371:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\277")) goto l372; goto l307;
|
|
l372:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\300")) goto l373; goto l307;
|
|
l373:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\301")) goto l374; goto l307;
|
|
l374:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\302")) goto l375; goto l307;
|
|
l375:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\303")) goto l376; goto l307;
|
|
l376:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\304")) goto l377; goto l307;
|
|
l377:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\305")) goto l378; goto l307;
|
|
l378:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\306")) goto l379; goto l307;
|
|
l379:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\307")) goto l380; goto l307;
|
|
l380:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\310")) goto l381; goto l307;
|
|
l381:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\311")) goto l382; goto l307;
|
|
l382:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\312")) goto l383; goto l307;
|
|
l383:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\313")) goto l384; goto l307;
|
|
l384:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\314")) goto l385; goto l307;
|
|
l385:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\315")) goto l386; goto l307;
|
|
l386:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\316")) goto l387; goto l307;
|
|
l387:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\317")) goto l388; goto l307;
|
|
l388:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\320")) goto l389; goto l307;
|
|
l389:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\321")) goto l390; goto l307;
|
|
l390:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\322")) goto l391; goto l307;
|
|
l391:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\323")) goto l392; goto l307;
|
|
l392:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\324")) goto l393; goto l307;
|
|
l393:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\325")) goto l394; goto l307;
|
|
l394:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\326")) goto l395; goto l307;
|
|
l395:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\327")) goto l396; goto l307;
|
|
l396:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\330")) goto l397; goto l307;
|
|
l397:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\331")) goto l398; goto l307;
|
|
l398:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\332")) goto l399; goto l307;
|
|
l399:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\333")) goto l400; goto l307;
|
|
l400:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\334")) goto l401; goto l307;
|
|
l401:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\335")) goto l402; goto l307;
|
|
l402:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\336")) goto l403; goto l307;
|
|
l403:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\337")) goto l404; goto l307;
|
|
l404:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\340")) goto l405; goto l307;
|
|
l405:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\341")) goto l406; goto l307;
|
|
l406:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\342")) goto l407; goto l307;
|
|
l407:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\343")) goto l408; goto l307;
|
|
l408:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\344")) goto l409; goto l307;
|
|
l409:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\345")) goto l410; goto l307;
|
|
l410:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\346")) goto l411; goto l307;
|
|
l411:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\347")) goto l412; goto l307;
|
|
l412:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\350")) goto l413; goto l307;
|
|
l413:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\351")) goto l414; goto l307;
|
|
l414:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\352")) goto l415; goto l307;
|
|
l415:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\353")) goto l416; goto l307;
|
|
l416:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\354")) goto l417; goto l307;
|
|
l417:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\355")) goto l418; goto l307;
|
|
l418:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\356")) goto l419; goto l307;
|
|
l419:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\357")) goto l420; goto l307;
|
|
l420:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\360")) goto l421; goto l307;
|
|
l421:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\361")) goto l422; goto l307;
|
|
l422:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\362")) goto l423; goto l307;
|
|
l423:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\363")) goto l424; goto l307;
|
|
l424:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\364")) goto l425; goto l307;
|
|
l425:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\365")) goto l426; goto l307;
|
|
l426:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\366")) goto l427; goto l307;
|
|
l427:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\367")) goto l428; goto l307;
|
|
l428:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\370")) goto l429; goto l307;
|
|
l429:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\371")) goto l430; goto l307;
|
|
l430:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\372")) goto l431; goto l307;
|
|
l431:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\373")) goto l432; goto l307;
|
|
l432:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\374")) goto l433; goto l307;
|
|
l433:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\375")) goto l434; goto l307;
|
|
l434:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\376")) goto l435; goto l307;
|
|
l435:; ctx->pos= yypos307; ctx->thunkpos= yythunkpos307; if (!yymatchString(ctx, "\377")) goto l306;
|
|
}
|
|
l307:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Alphanumeric", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l306:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Alphanumeric", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_StrChunk(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "StrChunk"));
|
|
{ int yypos437= ctx->pos, yythunkpos437= ctx->thunkpos; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l438;
|
|
{ int yypos441= ctx->pos, yythunkpos441= ctx->thunkpos; if (!yy_NormalChar(ctx)) goto l442; goto l441;
|
|
l442:; ctx->pos= yypos441; ctx->thunkpos= yythunkpos441; if (!yymatchChar(ctx, '_')) goto l438;
|
|
l443:;
|
|
{ int yypos444= ctx->pos, yythunkpos444= ctx->thunkpos; if (!yymatchChar(ctx, '_')) goto l444; goto l443;
|
|
l444:; ctx->pos= yypos444; ctx->thunkpos= yythunkpos444;
|
|
}
|
|
{ int yypos445= ctx->pos, yythunkpos445= ctx->thunkpos; if (!yy_Alphanumeric(ctx)) goto l438; ctx->pos= yypos445; ctx->thunkpos= yythunkpos445;
|
|
}
|
|
}
|
|
l441:;
|
|
l439:;
|
|
{ int yypos440= ctx->pos, yythunkpos440= ctx->thunkpos;
|
|
{ int yypos446= ctx->pos, yythunkpos446= ctx->thunkpos; if (!yy_NormalChar(ctx)) goto l447; goto l446;
|
|
l447:; ctx->pos= yypos446; ctx->thunkpos= yythunkpos446; if (!yymatchChar(ctx, '_')) goto l440;
|
|
l448:;
|
|
{ int yypos449= ctx->pos, yythunkpos449= ctx->thunkpos; if (!yymatchChar(ctx, '_')) goto l449; goto l448;
|
|
l449:; ctx->pos= yypos449; ctx->thunkpos= yythunkpos449;
|
|
}
|
|
{ int yypos450= ctx->pos, yythunkpos450= ctx->thunkpos; if (!yy_Alphanumeric(ctx)) goto l440; ctx->pos= yypos450; ctx->thunkpos= yythunkpos450;
|
|
}
|
|
}
|
|
l446:; goto l439;
|
|
l440:; ctx->pos= yypos440; ctx->thunkpos= yythunkpos440;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l438; yyDo(ctx, yy_1_StrChunk, ctx->begin, ctx->end); goto l437;
|
|
l438:; ctx->pos= yypos437; ctx->thunkpos= yythunkpos437; if (!yy_AposChunk(ctx)) goto l436;
|
|
}
|
|
l437:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "StrChunk", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l436:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "StrChunk", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_NormalChar(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "NormalChar"));
|
|
{ int yypos452= ctx->pos, yythunkpos452= ctx->thunkpos;
|
|
{ int yypos453= ctx->pos, yythunkpos453= ctx->thunkpos; if (!yy_SpecialChar(ctx)) goto l454; goto l453;
|
|
l454:; ctx->pos= yypos453; ctx->thunkpos= yythunkpos453; if (!yy_Spacechar(ctx)) goto l455; goto l453;
|
|
l455:; ctx->pos= yypos453; ctx->thunkpos= yythunkpos453; if (!yy_Newline(ctx)) goto l452;
|
|
}
|
|
l453:; goto l451;
|
|
l452:; ctx->pos= yypos452; ctx->thunkpos= yythunkpos452;
|
|
} if (!yymatchDot(ctx)) goto l451;
|
|
yyprintf((stderr, " ok %s @ %s\n", "NormalChar", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l451:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "NormalChar", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Symbol(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Symbol")); yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l456; if (!yy_SpecialChar(ctx)) goto l456; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l456; yyDo(ctx, yy_1_Symbol, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Symbol", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l456:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Symbol", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Smart(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Smart")); yyText(ctx, ctx->begin, ctx->end); if (!( extension(EXT_SMART) )) goto l457;
|
|
{ int yypos458= ctx->pos, yythunkpos458= ctx->thunkpos; if (!yy_Ellipsis(ctx)) goto l459; goto l458;
|
|
l459:; ctx->pos= yypos458; ctx->thunkpos= yythunkpos458; if (!yy_Dash(ctx)) goto l460; goto l458;
|
|
l460:; ctx->pos= yypos458; ctx->thunkpos= yythunkpos458; if (!yy_SingleQuoted(ctx)) goto l461; goto l458;
|
|
l461:; ctx->pos= yypos458; ctx->thunkpos= yythunkpos458; if (!yy_DoubleQuoted(ctx)) goto l462; goto l458;
|
|
l462:; ctx->pos= yypos458; ctx->thunkpos= yythunkpos458; if (!yy_Apostrophe(ctx)) goto l457;
|
|
}
|
|
l458:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Smart", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l457:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Smart", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_EscapedChar(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "EscapedChar")); if (!yymatchChar(ctx, '\\')) goto l463;
|
|
{ int yypos464= ctx->pos, yythunkpos464= ctx->thunkpos; if (!yy_Newline(ctx)) goto l464; goto l463;
|
|
l464:; ctx->pos= yypos464; ctx->thunkpos= yythunkpos464;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l463; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\012\157\000\120\000\000\000\270\001\000\000\070\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l463; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l463; yyDo(ctx, yy_1_EscapedChar, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "EscapedChar", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l463:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "EscapedChar", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Entity(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Entity"));
|
|
{ int yypos466= ctx->pos, yythunkpos466= ctx->thunkpos; if (!yy_HexEntity(ctx)) goto l467; goto l466;
|
|
l467:; ctx->pos= yypos466; ctx->thunkpos= yythunkpos466; if (!yy_DecEntity(ctx)) goto l468; goto l466;
|
|
l468:; ctx->pos= yypos466; ctx->thunkpos= yythunkpos466; if (!yy_CharEntity(ctx)) goto l465;
|
|
}
|
|
l466:; yyDo(ctx, yy_1_Entity, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Entity", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l465:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Entity", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_RawHtml(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "RawHtml")); yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l469;
|
|
{ int yypos470= ctx->pos, yythunkpos470= ctx->thunkpos; if (!yy_HtmlComment(ctx)) goto l471; goto l470;
|
|
l471:; ctx->pos= yypos470; ctx->thunkpos= yythunkpos470; if (!yy_HtmlBlockScript(ctx)) goto l472; goto l470;
|
|
l472:; ctx->pos= yypos470; ctx->thunkpos= yythunkpos470; if (!yy_HtmlTag(ctx)) goto l469;
|
|
}
|
|
l470:; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l469; yyDo(ctx, yy_1_RawHtml, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "RawHtml", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l469:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "RawHtml", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Code(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Code"));
|
|
{ int yypos474= ctx->pos, yythunkpos474= ctx->thunkpos; if (!yy_Ticks1(ctx)) goto l475; if (!yy_Sp(ctx)) goto l475; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l475;
|
|
{ int yypos478= ctx->pos, yythunkpos478= ctx->thunkpos;
|
|
{ int yypos482= ctx->pos, yythunkpos482= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l482; goto l479;
|
|
l482:; ctx->pos= yypos482; ctx->thunkpos= yythunkpos482;
|
|
} if (!yy_Nonspacechar(ctx)) goto l479;
|
|
l480:;
|
|
{ int yypos481= ctx->pos, yythunkpos481= ctx->thunkpos;
|
|
{ int yypos483= ctx->pos, yythunkpos483= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l483; goto l481;
|
|
l483:; ctx->pos= yypos483; ctx->thunkpos= yythunkpos483;
|
|
} if (!yy_Nonspacechar(ctx)) goto l481; goto l480;
|
|
l481:; ctx->pos= yypos481; ctx->thunkpos= yythunkpos481;
|
|
} goto l478;
|
|
l479:; ctx->pos= yypos478; ctx->thunkpos= yythunkpos478;
|
|
{ int yypos485= ctx->pos, yythunkpos485= ctx->thunkpos; if (!yy_Ticks1(ctx)) goto l485; goto l484;
|
|
l485:; ctx->pos= yypos485; ctx->thunkpos= yythunkpos485;
|
|
} if (!yymatchChar(ctx, '`')) goto l484;
|
|
l486:;
|
|
{ int yypos487= ctx->pos, yythunkpos487= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l487; goto l486;
|
|
l487:; ctx->pos= yypos487; ctx->thunkpos= yythunkpos487;
|
|
} goto l478;
|
|
l484:; ctx->pos= yypos478; ctx->thunkpos= yythunkpos478;
|
|
{ int yypos488= ctx->pos, yythunkpos488= ctx->thunkpos; if (!yy_Sp(ctx)) goto l488; if (!yy_Ticks1(ctx)) goto l488; goto l475;
|
|
l488:; ctx->pos= yypos488; ctx->thunkpos= yythunkpos488;
|
|
}
|
|
{ int yypos489= ctx->pos, yythunkpos489= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l490; goto l489;
|
|
l490:; ctx->pos= yypos489; ctx->thunkpos= yythunkpos489; if (!yy_Newline(ctx)) goto l475;
|
|
{ int yypos491= ctx->pos, yythunkpos491= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l491; goto l475;
|
|
l491:; ctx->pos= yypos491; ctx->thunkpos= yythunkpos491;
|
|
}
|
|
}
|
|
l489:;
|
|
}
|
|
l478:;
|
|
l476:;
|
|
{ int yypos477= ctx->pos, yythunkpos477= ctx->thunkpos;
|
|
{ int yypos492= ctx->pos, yythunkpos492= ctx->thunkpos;
|
|
{ int yypos496= ctx->pos, yythunkpos496= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l496; goto l493;
|
|
l496:; ctx->pos= yypos496; ctx->thunkpos= yythunkpos496;
|
|
} if (!yy_Nonspacechar(ctx)) goto l493;
|
|
l494:;
|
|
{ int yypos495= ctx->pos, yythunkpos495= ctx->thunkpos;
|
|
{ int yypos497= ctx->pos, yythunkpos497= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l497; goto l495;
|
|
l497:; ctx->pos= yypos497; ctx->thunkpos= yythunkpos497;
|
|
} if (!yy_Nonspacechar(ctx)) goto l495; goto l494;
|
|
l495:; ctx->pos= yypos495; ctx->thunkpos= yythunkpos495;
|
|
} goto l492;
|
|
l493:; ctx->pos= yypos492; ctx->thunkpos= yythunkpos492;
|
|
{ int yypos499= ctx->pos, yythunkpos499= ctx->thunkpos; if (!yy_Ticks1(ctx)) goto l499; goto l498;
|
|
l499:; ctx->pos= yypos499; ctx->thunkpos= yythunkpos499;
|
|
} if (!yymatchChar(ctx, '`')) goto l498;
|
|
l500:;
|
|
{ int yypos501= ctx->pos, yythunkpos501= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l501; goto l500;
|
|
l501:; ctx->pos= yypos501; ctx->thunkpos= yythunkpos501;
|
|
} goto l492;
|
|
l498:; ctx->pos= yypos492; ctx->thunkpos= yythunkpos492;
|
|
{ int yypos502= ctx->pos, yythunkpos502= ctx->thunkpos; if (!yy_Sp(ctx)) goto l502; if (!yy_Ticks1(ctx)) goto l502; goto l477;
|
|
l502:; ctx->pos= yypos502; ctx->thunkpos= yythunkpos502;
|
|
}
|
|
{ int yypos503= ctx->pos, yythunkpos503= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l504; goto l503;
|
|
l504:; ctx->pos= yypos503; ctx->thunkpos= yythunkpos503; if (!yy_Newline(ctx)) goto l477;
|
|
{ int yypos505= ctx->pos, yythunkpos505= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l505; goto l477;
|
|
l505:; ctx->pos= yypos505; ctx->thunkpos= yythunkpos505;
|
|
}
|
|
}
|
|
l503:;
|
|
}
|
|
l492:; goto l476;
|
|
l477:; ctx->pos= yypos477; ctx->thunkpos= yythunkpos477;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l475; if (!yy_Sp(ctx)) goto l475; if (!yy_Ticks1(ctx)) goto l475; goto l474;
|
|
l475:; ctx->pos= yypos474; ctx->thunkpos= yythunkpos474; if (!yy_Ticks2(ctx)) goto l506; if (!yy_Sp(ctx)) goto l506; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l506;
|
|
{ int yypos509= ctx->pos, yythunkpos509= ctx->thunkpos;
|
|
{ int yypos513= ctx->pos, yythunkpos513= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l513; goto l510;
|
|
l513:; ctx->pos= yypos513; ctx->thunkpos= yythunkpos513;
|
|
} if (!yy_Nonspacechar(ctx)) goto l510;
|
|
l511:;
|
|
{ int yypos512= ctx->pos, yythunkpos512= ctx->thunkpos;
|
|
{ int yypos514= ctx->pos, yythunkpos514= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l514; goto l512;
|
|
l514:; ctx->pos= yypos514; ctx->thunkpos= yythunkpos514;
|
|
} if (!yy_Nonspacechar(ctx)) goto l512; goto l511;
|
|
l512:; ctx->pos= yypos512; ctx->thunkpos= yythunkpos512;
|
|
} goto l509;
|
|
l510:; ctx->pos= yypos509; ctx->thunkpos= yythunkpos509;
|
|
{ int yypos516= ctx->pos, yythunkpos516= ctx->thunkpos; if (!yy_Ticks2(ctx)) goto l516; goto l515;
|
|
l516:; ctx->pos= yypos516; ctx->thunkpos= yythunkpos516;
|
|
} if (!yymatchChar(ctx, '`')) goto l515;
|
|
l517:;
|
|
{ int yypos518= ctx->pos, yythunkpos518= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l518; goto l517;
|
|
l518:; ctx->pos= yypos518; ctx->thunkpos= yythunkpos518;
|
|
} goto l509;
|
|
l515:; ctx->pos= yypos509; ctx->thunkpos= yythunkpos509;
|
|
{ int yypos519= ctx->pos, yythunkpos519= ctx->thunkpos; if (!yy_Sp(ctx)) goto l519; if (!yy_Ticks2(ctx)) goto l519; goto l506;
|
|
l519:; ctx->pos= yypos519; ctx->thunkpos= yythunkpos519;
|
|
}
|
|
{ int yypos520= ctx->pos, yythunkpos520= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l521; goto l520;
|
|
l521:; ctx->pos= yypos520; ctx->thunkpos= yythunkpos520; if (!yy_Newline(ctx)) goto l506;
|
|
{ int yypos522= ctx->pos, yythunkpos522= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l522; goto l506;
|
|
l522:; ctx->pos= yypos522; ctx->thunkpos= yythunkpos522;
|
|
}
|
|
}
|
|
l520:;
|
|
}
|
|
l509:;
|
|
l507:;
|
|
{ int yypos508= ctx->pos, yythunkpos508= ctx->thunkpos;
|
|
{ int yypos523= ctx->pos, yythunkpos523= ctx->thunkpos;
|
|
{ int yypos527= ctx->pos, yythunkpos527= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l527; goto l524;
|
|
l527:; ctx->pos= yypos527; ctx->thunkpos= yythunkpos527;
|
|
} if (!yy_Nonspacechar(ctx)) goto l524;
|
|
l525:;
|
|
{ int yypos526= ctx->pos, yythunkpos526= ctx->thunkpos;
|
|
{ int yypos528= ctx->pos, yythunkpos528= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l528; goto l526;
|
|
l528:; ctx->pos= yypos528; ctx->thunkpos= yythunkpos528;
|
|
} if (!yy_Nonspacechar(ctx)) goto l526; goto l525;
|
|
l526:; ctx->pos= yypos526; ctx->thunkpos= yythunkpos526;
|
|
} goto l523;
|
|
l524:; ctx->pos= yypos523; ctx->thunkpos= yythunkpos523;
|
|
{ int yypos530= ctx->pos, yythunkpos530= ctx->thunkpos; if (!yy_Ticks2(ctx)) goto l530; goto l529;
|
|
l530:; ctx->pos= yypos530; ctx->thunkpos= yythunkpos530;
|
|
} if (!yymatchChar(ctx, '`')) goto l529;
|
|
l531:;
|
|
{ int yypos532= ctx->pos, yythunkpos532= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l532; goto l531;
|
|
l532:; ctx->pos= yypos532; ctx->thunkpos= yythunkpos532;
|
|
} goto l523;
|
|
l529:; ctx->pos= yypos523; ctx->thunkpos= yythunkpos523;
|
|
{ int yypos533= ctx->pos, yythunkpos533= ctx->thunkpos; if (!yy_Sp(ctx)) goto l533; if (!yy_Ticks2(ctx)) goto l533; goto l508;
|
|
l533:; ctx->pos= yypos533; ctx->thunkpos= yythunkpos533;
|
|
}
|
|
{ int yypos534= ctx->pos, yythunkpos534= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l535; goto l534;
|
|
l535:; ctx->pos= yypos534; ctx->thunkpos= yythunkpos534; if (!yy_Newline(ctx)) goto l508;
|
|
{ int yypos536= ctx->pos, yythunkpos536= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l536; goto l508;
|
|
l536:; ctx->pos= yypos536; ctx->thunkpos= yythunkpos536;
|
|
}
|
|
}
|
|
l534:;
|
|
}
|
|
l523:; goto l507;
|
|
l508:; ctx->pos= yypos508; ctx->thunkpos= yythunkpos508;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l506; if (!yy_Sp(ctx)) goto l506; if (!yy_Ticks2(ctx)) goto l506; goto l474;
|
|
l506:; ctx->pos= yypos474; ctx->thunkpos= yythunkpos474; if (!yy_Ticks3(ctx)) goto l537; if (!yy_Sp(ctx)) goto l537; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l537;
|
|
{ int yypos540= ctx->pos, yythunkpos540= ctx->thunkpos;
|
|
{ int yypos544= ctx->pos, yythunkpos544= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l544; goto l541;
|
|
l544:; ctx->pos= yypos544; ctx->thunkpos= yythunkpos544;
|
|
} if (!yy_Nonspacechar(ctx)) goto l541;
|
|
l542:;
|
|
{ int yypos543= ctx->pos, yythunkpos543= ctx->thunkpos;
|
|
{ int yypos545= ctx->pos, yythunkpos545= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l545; goto l543;
|
|
l545:; ctx->pos= yypos545; ctx->thunkpos= yythunkpos545;
|
|
} if (!yy_Nonspacechar(ctx)) goto l543; goto l542;
|
|
l543:; ctx->pos= yypos543; ctx->thunkpos= yythunkpos543;
|
|
} goto l540;
|
|
l541:; ctx->pos= yypos540; ctx->thunkpos= yythunkpos540;
|
|
{ int yypos547= ctx->pos, yythunkpos547= ctx->thunkpos; if (!yy_Ticks3(ctx)) goto l547; goto l546;
|
|
l547:; ctx->pos= yypos547; ctx->thunkpos= yythunkpos547;
|
|
} if (!yymatchChar(ctx, '`')) goto l546;
|
|
l548:;
|
|
{ int yypos549= ctx->pos, yythunkpos549= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l549; goto l548;
|
|
l549:; ctx->pos= yypos549; ctx->thunkpos= yythunkpos549;
|
|
} goto l540;
|
|
l546:; ctx->pos= yypos540; ctx->thunkpos= yythunkpos540;
|
|
{ int yypos550= ctx->pos, yythunkpos550= ctx->thunkpos; if (!yy_Sp(ctx)) goto l550; if (!yy_Ticks3(ctx)) goto l550; goto l537;
|
|
l550:; ctx->pos= yypos550; ctx->thunkpos= yythunkpos550;
|
|
}
|
|
{ int yypos551= ctx->pos, yythunkpos551= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l552; goto l551;
|
|
l552:; ctx->pos= yypos551; ctx->thunkpos= yythunkpos551; if (!yy_Newline(ctx)) goto l537;
|
|
{ int yypos553= ctx->pos, yythunkpos553= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l553; goto l537;
|
|
l553:; ctx->pos= yypos553; ctx->thunkpos= yythunkpos553;
|
|
}
|
|
}
|
|
l551:;
|
|
}
|
|
l540:;
|
|
l538:;
|
|
{ int yypos539= ctx->pos, yythunkpos539= ctx->thunkpos;
|
|
{ int yypos554= ctx->pos, yythunkpos554= ctx->thunkpos;
|
|
{ int yypos558= ctx->pos, yythunkpos558= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l558; goto l555;
|
|
l558:; ctx->pos= yypos558; ctx->thunkpos= yythunkpos558;
|
|
} if (!yy_Nonspacechar(ctx)) goto l555;
|
|
l556:;
|
|
{ int yypos557= ctx->pos, yythunkpos557= ctx->thunkpos;
|
|
{ int yypos559= ctx->pos, yythunkpos559= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l559; goto l557;
|
|
l559:; ctx->pos= yypos559; ctx->thunkpos= yythunkpos559;
|
|
} if (!yy_Nonspacechar(ctx)) goto l557; goto l556;
|
|
l557:; ctx->pos= yypos557; ctx->thunkpos= yythunkpos557;
|
|
} goto l554;
|
|
l555:; ctx->pos= yypos554; ctx->thunkpos= yythunkpos554;
|
|
{ int yypos561= ctx->pos, yythunkpos561= ctx->thunkpos; if (!yy_Ticks3(ctx)) goto l561; goto l560;
|
|
l561:; ctx->pos= yypos561; ctx->thunkpos= yythunkpos561;
|
|
} if (!yymatchChar(ctx, '`')) goto l560;
|
|
l562:;
|
|
{ int yypos563= ctx->pos, yythunkpos563= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l563; goto l562;
|
|
l563:; ctx->pos= yypos563; ctx->thunkpos= yythunkpos563;
|
|
} goto l554;
|
|
l560:; ctx->pos= yypos554; ctx->thunkpos= yythunkpos554;
|
|
{ int yypos564= ctx->pos, yythunkpos564= ctx->thunkpos; if (!yy_Sp(ctx)) goto l564; if (!yy_Ticks3(ctx)) goto l564; goto l539;
|
|
l564:; ctx->pos= yypos564; ctx->thunkpos= yythunkpos564;
|
|
}
|
|
{ int yypos565= ctx->pos, yythunkpos565= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l566; goto l565;
|
|
l566:; ctx->pos= yypos565; ctx->thunkpos= yythunkpos565; if (!yy_Newline(ctx)) goto l539;
|
|
{ int yypos567= ctx->pos, yythunkpos567= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l567; goto l539;
|
|
l567:; ctx->pos= yypos567; ctx->thunkpos= yythunkpos567;
|
|
}
|
|
}
|
|
l565:;
|
|
}
|
|
l554:; goto l538;
|
|
l539:; ctx->pos= yypos539; ctx->thunkpos= yythunkpos539;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l537; if (!yy_Sp(ctx)) goto l537; if (!yy_Ticks3(ctx)) goto l537; goto l474;
|
|
l537:; ctx->pos= yypos474; ctx->thunkpos= yythunkpos474; if (!yy_Ticks4(ctx)) goto l568; if (!yy_Sp(ctx)) goto l568; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l568;
|
|
{ int yypos571= ctx->pos, yythunkpos571= ctx->thunkpos;
|
|
{ int yypos575= ctx->pos, yythunkpos575= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l575; goto l572;
|
|
l575:; ctx->pos= yypos575; ctx->thunkpos= yythunkpos575;
|
|
} if (!yy_Nonspacechar(ctx)) goto l572;
|
|
l573:;
|
|
{ int yypos574= ctx->pos, yythunkpos574= ctx->thunkpos;
|
|
{ int yypos576= ctx->pos, yythunkpos576= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l576; goto l574;
|
|
l576:; ctx->pos= yypos576; ctx->thunkpos= yythunkpos576;
|
|
} if (!yy_Nonspacechar(ctx)) goto l574; goto l573;
|
|
l574:; ctx->pos= yypos574; ctx->thunkpos= yythunkpos574;
|
|
} goto l571;
|
|
l572:; ctx->pos= yypos571; ctx->thunkpos= yythunkpos571;
|
|
{ int yypos578= ctx->pos, yythunkpos578= ctx->thunkpos; if (!yy_Ticks4(ctx)) goto l578; goto l577;
|
|
l578:; ctx->pos= yypos578; ctx->thunkpos= yythunkpos578;
|
|
} if (!yymatchChar(ctx, '`')) goto l577;
|
|
l579:;
|
|
{ int yypos580= ctx->pos, yythunkpos580= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l580; goto l579;
|
|
l580:; ctx->pos= yypos580; ctx->thunkpos= yythunkpos580;
|
|
} goto l571;
|
|
l577:; ctx->pos= yypos571; ctx->thunkpos= yythunkpos571;
|
|
{ int yypos581= ctx->pos, yythunkpos581= ctx->thunkpos; if (!yy_Sp(ctx)) goto l581; if (!yy_Ticks4(ctx)) goto l581; goto l568;
|
|
l581:; ctx->pos= yypos581; ctx->thunkpos= yythunkpos581;
|
|
}
|
|
{ int yypos582= ctx->pos, yythunkpos582= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l583; goto l582;
|
|
l583:; ctx->pos= yypos582; ctx->thunkpos= yythunkpos582; if (!yy_Newline(ctx)) goto l568;
|
|
{ int yypos584= ctx->pos, yythunkpos584= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l584; goto l568;
|
|
l584:; ctx->pos= yypos584; ctx->thunkpos= yythunkpos584;
|
|
}
|
|
}
|
|
l582:;
|
|
}
|
|
l571:;
|
|
l569:;
|
|
{ int yypos570= ctx->pos, yythunkpos570= ctx->thunkpos;
|
|
{ int yypos585= ctx->pos, yythunkpos585= ctx->thunkpos;
|
|
{ int yypos589= ctx->pos, yythunkpos589= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l589; goto l586;
|
|
l589:; ctx->pos= yypos589; ctx->thunkpos= yythunkpos589;
|
|
} if (!yy_Nonspacechar(ctx)) goto l586;
|
|
l587:;
|
|
{ int yypos588= ctx->pos, yythunkpos588= ctx->thunkpos;
|
|
{ int yypos590= ctx->pos, yythunkpos590= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l590; goto l588;
|
|
l590:; ctx->pos= yypos590; ctx->thunkpos= yythunkpos590;
|
|
} if (!yy_Nonspacechar(ctx)) goto l588; goto l587;
|
|
l588:; ctx->pos= yypos588; ctx->thunkpos= yythunkpos588;
|
|
} goto l585;
|
|
l586:; ctx->pos= yypos585; ctx->thunkpos= yythunkpos585;
|
|
{ int yypos592= ctx->pos, yythunkpos592= ctx->thunkpos; if (!yy_Ticks4(ctx)) goto l592; goto l591;
|
|
l592:; ctx->pos= yypos592; ctx->thunkpos= yythunkpos592;
|
|
} if (!yymatchChar(ctx, '`')) goto l591;
|
|
l593:;
|
|
{ int yypos594= ctx->pos, yythunkpos594= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l594; goto l593;
|
|
l594:; ctx->pos= yypos594; ctx->thunkpos= yythunkpos594;
|
|
} goto l585;
|
|
l591:; ctx->pos= yypos585; ctx->thunkpos= yythunkpos585;
|
|
{ int yypos595= ctx->pos, yythunkpos595= ctx->thunkpos; if (!yy_Sp(ctx)) goto l595; if (!yy_Ticks4(ctx)) goto l595; goto l570;
|
|
l595:; ctx->pos= yypos595; ctx->thunkpos= yythunkpos595;
|
|
}
|
|
{ int yypos596= ctx->pos, yythunkpos596= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l597; goto l596;
|
|
l597:; ctx->pos= yypos596; ctx->thunkpos= yythunkpos596; if (!yy_Newline(ctx)) goto l570;
|
|
{ int yypos598= ctx->pos, yythunkpos598= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l598; goto l570;
|
|
l598:; ctx->pos= yypos598; ctx->thunkpos= yythunkpos598;
|
|
}
|
|
}
|
|
l596:;
|
|
}
|
|
l585:; goto l569;
|
|
l570:; ctx->pos= yypos570; ctx->thunkpos= yythunkpos570;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l568; if (!yy_Sp(ctx)) goto l568; if (!yy_Ticks4(ctx)) goto l568; goto l474;
|
|
l568:; ctx->pos= yypos474; ctx->thunkpos= yythunkpos474; if (!yy_Ticks5(ctx)) goto l473; if (!yy_Sp(ctx)) goto l473; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l473;
|
|
{ int yypos601= ctx->pos, yythunkpos601= ctx->thunkpos;
|
|
{ int yypos605= ctx->pos, yythunkpos605= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l605; goto l602;
|
|
l605:; ctx->pos= yypos605; ctx->thunkpos= yythunkpos605;
|
|
} if (!yy_Nonspacechar(ctx)) goto l602;
|
|
l603:;
|
|
{ int yypos604= ctx->pos, yythunkpos604= ctx->thunkpos;
|
|
{ int yypos606= ctx->pos, yythunkpos606= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l606; goto l604;
|
|
l606:; ctx->pos= yypos606; ctx->thunkpos= yythunkpos606;
|
|
} if (!yy_Nonspacechar(ctx)) goto l604; goto l603;
|
|
l604:; ctx->pos= yypos604; ctx->thunkpos= yythunkpos604;
|
|
} goto l601;
|
|
l602:; ctx->pos= yypos601; ctx->thunkpos= yythunkpos601;
|
|
{ int yypos608= ctx->pos, yythunkpos608= ctx->thunkpos; if (!yy_Ticks5(ctx)) goto l608; goto l607;
|
|
l608:; ctx->pos= yypos608; ctx->thunkpos= yythunkpos608;
|
|
} if (!yymatchChar(ctx, '`')) goto l607;
|
|
l609:;
|
|
{ int yypos610= ctx->pos, yythunkpos610= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l610; goto l609;
|
|
l610:; ctx->pos= yypos610; ctx->thunkpos= yythunkpos610;
|
|
} goto l601;
|
|
l607:; ctx->pos= yypos601; ctx->thunkpos= yythunkpos601;
|
|
{ int yypos611= ctx->pos, yythunkpos611= ctx->thunkpos; if (!yy_Sp(ctx)) goto l611; if (!yy_Ticks5(ctx)) goto l611; goto l473;
|
|
l611:; ctx->pos= yypos611; ctx->thunkpos= yythunkpos611;
|
|
}
|
|
{ int yypos612= ctx->pos, yythunkpos612= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l613; goto l612;
|
|
l613:; ctx->pos= yypos612; ctx->thunkpos= yythunkpos612; if (!yy_Newline(ctx)) goto l473;
|
|
{ int yypos614= ctx->pos, yythunkpos614= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l614; goto l473;
|
|
l614:; ctx->pos= yypos614; ctx->thunkpos= yythunkpos614;
|
|
}
|
|
}
|
|
l612:;
|
|
}
|
|
l601:;
|
|
l599:;
|
|
{ int yypos600= ctx->pos, yythunkpos600= ctx->thunkpos;
|
|
{ int yypos615= ctx->pos, yythunkpos615= ctx->thunkpos;
|
|
{ int yypos619= ctx->pos, yythunkpos619= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l619; goto l616;
|
|
l619:; ctx->pos= yypos619; ctx->thunkpos= yythunkpos619;
|
|
} if (!yy_Nonspacechar(ctx)) goto l616;
|
|
l617:;
|
|
{ int yypos618= ctx->pos, yythunkpos618= ctx->thunkpos;
|
|
{ int yypos620= ctx->pos, yythunkpos620= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l620; goto l618;
|
|
l620:; ctx->pos= yypos620; ctx->thunkpos= yythunkpos620;
|
|
} if (!yy_Nonspacechar(ctx)) goto l618; goto l617;
|
|
l618:; ctx->pos= yypos618; ctx->thunkpos= yythunkpos618;
|
|
} goto l615;
|
|
l616:; ctx->pos= yypos615; ctx->thunkpos= yythunkpos615;
|
|
{ int yypos622= ctx->pos, yythunkpos622= ctx->thunkpos; if (!yy_Ticks5(ctx)) goto l622; goto l621;
|
|
l622:; ctx->pos= yypos622; ctx->thunkpos= yythunkpos622;
|
|
} if (!yymatchChar(ctx, '`')) goto l621;
|
|
l623:;
|
|
{ int yypos624= ctx->pos, yythunkpos624= ctx->thunkpos; if (!yymatchChar(ctx, '`')) goto l624; goto l623;
|
|
l624:; ctx->pos= yypos624; ctx->thunkpos= yythunkpos624;
|
|
} goto l615;
|
|
l621:; ctx->pos= yypos615; ctx->thunkpos= yythunkpos615;
|
|
{ int yypos625= ctx->pos, yythunkpos625= ctx->thunkpos; if (!yy_Sp(ctx)) goto l625; if (!yy_Ticks5(ctx)) goto l625; goto l600;
|
|
l625:; ctx->pos= yypos625; ctx->thunkpos= yythunkpos625;
|
|
}
|
|
{ int yypos626= ctx->pos, yythunkpos626= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l627; goto l626;
|
|
l627:; ctx->pos= yypos626; ctx->thunkpos= yythunkpos626; if (!yy_Newline(ctx)) goto l600;
|
|
{ int yypos628= ctx->pos, yythunkpos628= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l628; goto l600;
|
|
l628:; ctx->pos= yypos628; ctx->thunkpos= yythunkpos628;
|
|
}
|
|
}
|
|
l626:;
|
|
}
|
|
l615:; goto l599;
|
|
l600:; ctx->pos= yypos600; ctx->thunkpos= yythunkpos600;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l473; if (!yy_Sp(ctx)) goto l473; if (!yy_Ticks5(ctx)) goto l473;
|
|
}
|
|
l474:; yyDo(ctx, yy_1_Code, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Code", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l473:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Code", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_InlineNote(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "InlineNote")); yyText(ctx, ctx->begin, ctx->end); if (!( extension(EXT_NOTES) )) goto l629; if (!yymatchString(ctx, "^[")) goto l629; if (!yy_StartList(ctx)) goto l629; yyDo(ctx, yySet, -1, 0);
|
|
{ int yypos632= ctx->pos, yythunkpos632= ctx->thunkpos; if (!yymatchChar(ctx, ']')) goto l632; goto l629;
|
|
l632:; ctx->pos= yypos632; ctx->thunkpos= yythunkpos632;
|
|
} if (!yy_Inline(ctx)) goto l629; yyDo(ctx, yy_1_InlineNote, ctx->begin, ctx->end);
|
|
l630:;
|
|
{ int yypos631= ctx->pos, yythunkpos631= ctx->thunkpos;
|
|
{ int yypos633= ctx->pos, yythunkpos633= ctx->thunkpos; if (!yymatchChar(ctx, ']')) goto l633; goto l631;
|
|
l633:; ctx->pos= yypos633; ctx->thunkpos= yythunkpos633;
|
|
} if (!yy_Inline(ctx)) goto l631; yyDo(ctx, yy_1_InlineNote, ctx->begin, ctx->end); goto l630;
|
|
l631:; ctx->pos= yypos631; ctx->thunkpos= yythunkpos631;
|
|
} if (!yymatchChar(ctx, ']')) goto l629; yyDo(ctx, yy_2_InlineNote, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "InlineNote", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l629:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "InlineNote", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_NoteReference(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "NoteReference")); yyText(ctx, ctx->begin, ctx->end); if (!( extension(EXT_NOTES) )) goto l634; if (!yy_RawNoteReference(ctx)) goto l634; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_NoteReference, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "NoteReference", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l634:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "NoteReference", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Link(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Link"));
|
|
{ int yypos636= ctx->pos, yythunkpos636= ctx->thunkpos; if (!yy_ExplicitLink(ctx)) goto l637; goto l636;
|
|
l637:; ctx->pos= yypos636; ctx->thunkpos= yythunkpos636; if (!yy_ReferenceLink(ctx)) goto l638; goto l636;
|
|
l638:; ctx->pos= yypos636; ctx->thunkpos= yythunkpos636; if (!yy_AutoLink(ctx)) goto l635;
|
|
}
|
|
l636:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Link", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l635:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Link", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Image(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Image")); if (!yymatchChar(ctx, '!')) goto l639;
|
|
{ int yypos640= ctx->pos, yythunkpos640= ctx->thunkpos; if (!yy_ExplicitLink(ctx)) goto l641; goto l640;
|
|
l641:; ctx->pos= yypos640; ctx->thunkpos= yythunkpos640; if (!yy_ReferenceLink(ctx)) goto l639;
|
|
}
|
|
l640:; yyDo(ctx, yy_1_Image, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Image", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l639:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Image", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Emph(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Emph"));
|
|
{ int yypos643= ctx->pos, yythunkpos643= ctx->thunkpos; if (!yy_EmphStar(ctx)) goto l644; goto l643;
|
|
l644:; ctx->pos= yypos643; ctx->thunkpos= yythunkpos643; if (!yy_EmphUl(ctx)) goto l642;
|
|
}
|
|
l643:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Emph", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l642:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Emph", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Strong(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Strong"));
|
|
{ int yypos646= ctx->pos, yythunkpos646= ctx->thunkpos; if (!yy_StrongStar(ctx)) goto l647; goto l646;
|
|
l647:; ctx->pos= yypos646; ctx->thunkpos= yythunkpos646; if (!yy_StrongUl(ctx)) goto l645;
|
|
}
|
|
l646:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Strong", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l645:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Strong", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Space(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Space")); if (!yy_Spacechar(ctx)) goto l648;
|
|
l649:;
|
|
{ int yypos650= ctx->pos, yythunkpos650= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l650; goto l649;
|
|
l650:; ctx->pos= yypos650; ctx->thunkpos= yythunkpos650;
|
|
} yyDo(ctx, yy_1_Space, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Space", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l648:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Space", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_UlOrStarLine(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "UlOrStarLine"));
|
|
{ int yypos652= ctx->pos, yythunkpos652= ctx->thunkpos; if (!yy_UlLine(ctx)) goto l653; goto l652;
|
|
l653:; ctx->pos= yypos652; ctx->thunkpos= yythunkpos652; if (!yy_StarLine(ctx)) goto l651;
|
|
}
|
|
l652:; yyDo(ctx, yy_1_UlOrStarLine, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "UlOrStarLine", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l651:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "UlOrStarLine", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Str(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "Str")); if (!yy_StartList(ctx)) goto l654; yyDo(ctx, yySet, -1, 0); yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l654; if (!yy_NormalChar(ctx)) goto l654;
|
|
l655:;
|
|
{ int yypos656= ctx->pos, yythunkpos656= ctx->thunkpos; if (!yy_NormalChar(ctx)) goto l656; goto l655;
|
|
l656:; ctx->pos= yypos656; ctx->thunkpos= yythunkpos656;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l654; yyDo(ctx, yy_1_Str, ctx->begin, ctx->end);
|
|
l657:;
|
|
{ int yypos658= ctx->pos, yythunkpos658= ctx->thunkpos; if (!yy_StrChunk(ctx)) goto l658; yyDo(ctx, yy_2_Str, ctx->begin, ctx->end); goto l657;
|
|
l658:; ctx->pos= yypos658; ctx->thunkpos= yythunkpos658;
|
|
} yyDo(ctx, yy_3_Str, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Str", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l654:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Str", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_InStyleTags(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "InStyleTags")); if (!yy_StyleOpen(ctx)) goto l659;
|
|
l660:;
|
|
{ int yypos661= ctx->pos, yythunkpos661= ctx->thunkpos;
|
|
{ int yypos662= ctx->pos, yythunkpos662= ctx->thunkpos; if (!yy_StyleClose(ctx)) goto l662; goto l661;
|
|
l662:; ctx->pos= yypos662; ctx->thunkpos= yythunkpos662;
|
|
} if (!yymatchDot(ctx)) goto l661; goto l660;
|
|
l661:; ctx->pos= yypos661; ctx->thunkpos= yythunkpos661;
|
|
} if (!yy_StyleClose(ctx)) goto l659;
|
|
yyprintf((stderr, " ok %s @ %s\n", "InStyleTags", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l659:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "InStyleTags", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_StyleClose(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "StyleClose")); if (!yymatchChar(ctx, '<')) goto l663; if (!yy_Spnl(ctx)) goto l663; if (!yymatchChar(ctx, '/')) goto l663;
|
|
{ int yypos664= ctx->pos, yythunkpos664= ctx->thunkpos; if (!yymatchString(ctx, "style")) goto l665; goto l664;
|
|
l665:; ctx->pos= yypos664; ctx->thunkpos= yythunkpos664; if (!yymatchString(ctx, "STYLE")) goto l663;
|
|
}
|
|
l664:; if (!yy_Spnl(ctx)) goto l663; if (!yymatchChar(ctx, '>')) goto l663;
|
|
yyprintf((stderr, " ok %s @ %s\n", "StyleClose", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l663:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "StyleClose", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_StyleOpen(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "StyleOpen")); if (!yymatchChar(ctx, '<')) goto l666; if (!yy_Spnl(ctx)) goto l666;
|
|
{ int yypos667= ctx->pos, yythunkpos667= ctx->thunkpos; if (!yymatchString(ctx, "style")) goto l668; goto l667;
|
|
l668:; ctx->pos= yypos667; ctx->thunkpos= yythunkpos667; if (!yymatchString(ctx, "STYLE")) goto l666;
|
|
}
|
|
l667:; if (!yy_Spnl(ctx)) goto l666;
|
|
l669:;
|
|
{ int yypos670= ctx->pos, yythunkpos670= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l670; goto l669;
|
|
l670:; ctx->pos= yypos670; ctx->thunkpos= yythunkpos670;
|
|
} if (!yymatchChar(ctx, '>')) goto l666;
|
|
yyprintf((stderr, " ok %s @ %s\n", "StyleOpen", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l666:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "StyleOpen", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockType(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockType"));
|
|
{ int yypos672= ctx->pos, yythunkpos672= ctx->thunkpos; if (!yymatchString(ctx, "address")) goto l673; goto l672;
|
|
l673:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "blockquote")) goto l674; goto l672;
|
|
l674:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "center")) goto l675; goto l672;
|
|
l675:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "dir")) goto l676; goto l672;
|
|
l676:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "div")) goto l677; goto l672;
|
|
l677:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "dl")) goto l678; goto l672;
|
|
l678:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "fieldset")) goto l679; goto l672;
|
|
l679:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "form")) goto l680; goto l672;
|
|
l680:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "h1")) goto l681; goto l672;
|
|
l681:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "h2")) goto l682; goto l672;
|
|
l682:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "h3")) goto l683; goto l672;
|
|
l683:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "h4")) goto l684; goto l672;
|
|
l684:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "h5")) goto l685; goto l672;
|
|
l685:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "h6")) goto l686; goto l672;
|
|
l686:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "hr")) goto l687; goto l672;
|
|
l687:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "isindex")) goto l688; goto l672;
|
|
l688:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "menu")) goto l689; goto l672;
|
|
l689:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "noframes")) goto l690; goto l672;
|
|
l690:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "noscript")) goto l691; goto l672;
|
|
l691:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "ol")) goto l692; goto l672;
|
|
l692:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchChar(ctx, 'p')) goto l693; goto l672;
|
|
l693:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "pre")) goto l694; goto l672;
|
|
l694:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "table")) goto l695; goto l672;
|
|
l695:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "ul")) goto l696; goto l672;
|
|
l696:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "dd")) goto l697; goto l672;
|
|
l697:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "dt")) goto l698; goto l672;
|
|
l698:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "frameset")) goto l699; goto l672;
|
|
l699:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "li")) goto l700; goto l672;
|
|
l700:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "tbody")) goto l701; goto l672;
|
|
l701:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "td")) goto l702; goto l672;
|
|
l702:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "tfoot")) goto l703; goto l672;
|
|
l703:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "th")) goto l704; goto l672;
|
|
l704:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "thead")) goto l705; goto l672;
|
|
l705:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "tr")) goto l706; goto l672;
|
|
l706:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "script")) goto l707; goto l672;
|
|
l707:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "ADDRESS")) goto l708; goto l672;
|
|
l708:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "BLOCKQUOTE")) goto l709; goto l672;
|
|
l709:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "CENTER")) goto l710; goto l672;
|
|
l710:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "DIR")) goto l711; goto l672;
|
|
l711:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "DIV")) goto l712; goto l672;
|
|
l712:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "DL")) goto l713; goto l672;
|
|
l713:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "FIELDSET")) goto l714; goto l672;
|
|
l714:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "FORM")) goto l715; goto l672;
|
|
l715:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "H1")) goto l716; goto l672;
|
|
l716:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "H2")) goto l717; goto l672;
|
|
l717:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "H3")) goto l718; goto l672;
|
|
l718:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "H4")) goto l719; goto l672;
|
|
l719:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "H5")) goto l720; goto l672;
|
|
l720:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "H6")) goto l721; goto l672;
|
|
l721:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "HR")) goto l722; goto l672;
|
|
l722:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "ISINDEX")) goto l723; goto l672;
|
|
l723:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "MENU")) goto l724; goto l672;
|
|
l724:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "NOFRAMES")) goto l725; goto l672;
|
|
l725:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "NOSCRIPT")) goto l726; goto l672;
|
|
l726:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "OL")) goto l727; goto l672;
|
|
l727:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchChar(ctx, 'P')) goto l728; goto l672;
|
|
l728:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "PRE")) goto l729; goto l672;
|
|
l729:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "TABLE")) goto l730; goto l672;
|
|
l730:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "UL")) goto l731; goto l672;
|
|
l731:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "DD")) goto l732; goto l672;
|
|
l732:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "DT")) goto l733; goto l672;
|
|
l733:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "FRAMESET")) goto l734; goto l672;
|
|
l734:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "LI")) goto l735; goto l672;
|
|
l735:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "TBODY")) goto l736; goto l672;
|
|
l736:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "TD")) goto l737; goto l672;
|
|
l737:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "TFOOT")) goto l738; goto l672;
|
|
l738:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "TH")) goto l739; goto l672;
|
|
l739:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "THEAD")) goto l740; goto l672;
|
|
l740:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "TR")) goto l741; goto l672;
|
|
l741:; ctx->pos= yypos672; ctx->thunkpos= yythunkpos672; if (!yymatchString(ctx, "SCRIPT")) goto l671;
|
|
}
|
|
l672:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockType", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l671:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockType", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockSelfClosing(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockSelfClosing")); if (!yymatchChar(ctx, '<')) goto l742; if (!yy_Spnl(ctx)) goto l742; if (!yy_HtmlBlockType(ctx)) goto l742; if (!yy_Spnl(ctx)) goto l742;
|
|
l743:;
|
|
{ int yypos744= ctx->pos, yythunkpos744= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l744; goto l743;
|
|
l744:; ctx->pos= yypos744; ctx->thunkpos= yythunkpos744;
|
|
} if (!yymatchChar(ctx, '/')) goto l742; if (!yy_Spnl(ctx)) goto l742; if (!yymatchChar(ctx, '>')) goto l742;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockSelfClosing", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l742:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockSelfClosing", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlComment(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlComment")); if (!yymatchString(ctx, "<!--")) goto l745;
|
|
l746:;
|
|
{ int yypos747= ctx->pos, yythunkpos747= ctx->thunkpos;
|
|
{ int yypos748= ctx->pos, yythunkpos748= ctx->thunkpos; if (!yymatchString(ctx, "-->")) goto l748; goto l747;
|
|
l748:; ctx->pos= yypos748; ctx->thunkpos= yythunkpos748;
|
|
} if (!yymatchDot(ctx)) goto l747; goto l746;
|
|
l747:; ctx->pos= yypos747; ctx->thunkpos= yythunkpos747;
|
|
} if (!yymatchString(ctx, "-->")) goto l745;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlComment", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l745:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlComment", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockInTags(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockInTags"));
|
|
{ int yypos750= ctx->pos, yythunkpos750= ctx->thunkpos; if (!yy_HtmlBlockAddress(ctx)) goto l751; goto l750;
|
|
l751:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockBlockquote(ctx)) goto l752; goto l750;
|
|
l752:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockCenter(ctx)) goto l753; goto l750;
|
|
l753:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockDir(ctx)) goto l754; goto l750;
|
|
l754:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockDiv(ctx)) goto l755; goto l750;
|
|
l755:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockDl(ctx)) goto l756; goto l750;
|
|
l756:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockFieldset(ctx)) goto l757; goto l750;
|
|
l757:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockForm(ctx)) goto l758; goto l750;
|
|
l758:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockH1(ctx)) goto l759; goto l750;
|
|
l759:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockH2(ctx)) goto l760; goto l750;
|
|
l760:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockH3(ctx)) goto l761; goto l750;
|
|
l761:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockH4(ctx)) goto l762; goto l750;
|
|
l762:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockH5(ctx)) goto l763; goto l750;
|
|
l763:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockH6(ctx)) goto l764; goto l750;
|
|
l764:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockMenu(ctx)) goto l765; goto l750;
|
|
l765:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockNoframes(ctx)) goto l766; goto l750;
|
|
l766:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockNoscript(ctx)) goto l767; goto l750;
|
|
l767:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockOl(ctx)) goto l768; goto l750;
|
|
l768:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockP(ctx)) goto l769; goto l750;
|
|
l769:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockPre(ctx)) goto l770; goto l750;
|
|
l770:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockTable(ctx)) goto l771; goto l750;
|
|
l771:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockUl(ctx)) goto l772; goto l750;
|
|
l772:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockDd(ctx)) goto l773; goto l750;
|
|
l773:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockDt(ctx)) goto l774; goto l750;
|
|
l774:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockFrameset(ctx)) goto l775; goto l750;
|
|
l775:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockLi(ctx)) goto l776; goto l750;
|
|
l776:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockTbody(ctx)) goto l777; goto l750;
|
|
l777:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockTd(ctx)) goto l778; goto l750;
|
|
l778:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockTfoot(ctx)) goto l779; goto l750;
|
|
l779:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockTh(ctx)) goto l780; goto l750;
|
|
l780:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockThead(ctx)) goto l781; goto l750;
|
|
l781:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockTr(ctx)) goto l782; goto l750;
|
|
l782:; ctx->pos= yypos750; ctx->thunkpos= yythunkpos750; if (!yy_HtmlBlockScript(ctx)) goto l749;
|
|
}
|
|
l750:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockInTags", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l749:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockInTags", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockScript(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockScript")); if (!yy_HtmlBlockOpenScript(ctx)) goto l783;
|
|
l784:;
|
|
{ int yypos785= ctx->pos, yythunkpos785= ctx->thunkpos;
|
|
{ int yypos786= ctx->pos, yythunkpos786= ctx->thunkpos; if (!yy_HtmlBlockCloseScript(ctx)) goto l786; goto l785;
|
|
l786:; ctx->pos= yypos786; ctx->thunkpos= yythunkpos786;
|
|
} if (!yymatchDot(ctx)) goto l785; goto l784;
|
|
l785:; ctx->pos= yypos785; ctx->thunkpos= yythunkpos785;
|
|
} if (!yy_HtmlBlockCloseScript(ctx)) goto l783;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockScript", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l783:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockScript", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseScript(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseScript")); if (!yymatchChar(ctx, '<')) goto l787; if (!yy_Spnl(ctx)) goto l787; if (!yymatchChar(ctx, '/')) goto l787;
|
|
{ int yypos788= ctx->pos, yythunkpos788= ctx->thunkpos; if (!yymatchString(ctx, "script")) goto l789; goto l788;
|
|
l789:; ctx->pos= yypos788; ctx->thunkpos= yythunkpos788; if (!yymatchString(ctx, "SCRIPT")) goto l787;
|
|
}
|
|
l788:; if (!yy_Spnl(ctx)) goto l787; if (!yymatchChar(ctx, '>')) goto l787;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseScript", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l787:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseScript", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenScript(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenScript")); if (!yymatchChar(ctx, '<')) goto l790; if (!yy_Spnl(ctx)) goto l790;
|
|
{ int yypos791= ctx->pos, yythunkpos791= ctx->thunkpos; if (!yymatchString(ctx, "script")) goto l792; goto l791;
|
|
l792:; ctx->pos= yypos791; ctx->thunkpos= yythunkpos791; if (!yymatchString(ctx, "SCRIPT")) goto l790;
|
|
}
|
|
l791:; if (!yy_Spnl(ctx)) goto l790;
|
|
l793:;
|
|
{ int yypos794= ctx->pos, yythunkpos794= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l794; goto l793;
|
|
l794:; ctx->pos= yypos794; ctx->thunkpos= yythunkpos794;
|
|
} if (!yymatchChar(ctx, '>')) goto l790;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenScript", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l790:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenScript", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockTr(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockTr")); if (!yy_HtmlBlockOpenTr(ctx)) goto l795;
|
|
l796:;
|
|
{ int yypos797= ctx->pos, yythunkpos797= ctx->thunkpos;
|
|
{ int yypos798= ctx->pos, yythunkpos798= ctx->thunkpos; if (!yy_HtmlBlockTr(ctx)) goto l799; goto l798;
|
|
l799:; ctx->pos= yypos798; ctx->thunkpos= yythunkpos798;
|
|
{ int yypos800= ctx->pos, yythunkpos800= ctx->thunkpos; if (!yy_HtmlBlockCloseTr(ctx)) goto l800; goto l797;
|
|
l800:; ctx->pos= yypos800; ctx->thunkpos= yythunkpos800;
|
|
} if (!yymatchDot(ctx)) goto l797;
|
|
}
|
|
l798:; goto l796;
|
|
l797:; ctx->pos= yypos797; ctx->thunkpos= yythunkpos797;
|
|
} if (!yy_HtmlBlockCloseTr(ctx)) goto l795;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockTr", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l795:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockTr", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseTr(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseTr")); if (!yymatchChar(ctx, '<')) goto l801; if (!yy_Spnl(ctx)) goto l801; if (!yymatchChar(ctx, '/')) goto l801;
|
|
{ int yypos802= ctx->pos, yythunkpos802= ctx->thunkpos; if (!yymatchString(ctx, "tr")) goto l803; goto l802;
|
|
l803:; ctx->pos= yypos802; ctx->thunkpos= yythunkpos802; if (!yymatchString(ctx, "TR")) goto l801;
|
|
}
|
|
l802:; if (!yy_Spnl(ctx)) goto l801; if (!yymatchChar(ctx, '>')) goto l801;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseTr", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l801:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseTr", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenTr(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenTr")); if (!yymatchChar(ctx, '<')) goto l804; if (!yy_Spnl(ctx)) goto l804;
|
|
{ int yypos805= ctx->pos, yythunkpos805= ctx->thunkpos; if (!yymatchString(ctx, "tr")) goto l806; goto l805;
|
|
l806:; ctx->pos= yypos805; ctx->thunkpos= yythunkpos805; if (!yymatchString(ctx, "TR")) goto l804;
|
|
}
|
|
l805:; if (!yy_Spnl(ctx)) goto l804;
|
|
l807:;
|
|
{ int yypos808= ctx->pos, yythunkpos808= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l808; goto l807;
|
|
l808:; ctx->pos= yypos808; ctx->thunkpos= yythunkpos808;
|
|
} if (!yymatchChar(ctx, '>')) goto l804;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenTr", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l804:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenTr", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockThead(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockThead")); if (!yy_HtmlBlockOpenThead(ctx)) goto l809;
|
|
l810:;
|
|
{ int yypos811= ctx->pos, yythunkpos811= ctx->thunkpos;
|
|
{ int yypos812= ctx->pos, yythunkpos812= ctx->thunkpos; if (!yy_HtmlBlockThead(ctx)) goto l813; goto l812;
|
|
l813:; ctx->pos= yypos812; ctx->thunkpos= yythunkpos812;
|
|
{ int yypos814= ctx->pos, yythunkpos814= ctx->thunkpos; if (!yy_HtmlBlockCloseThead(ctx)) goto l814; goto l811;
|
|
l814:; ctx->pos= yypos814; ctx->thunkpos= yythunkpos814;
|
|
} if (!yymatchDot(ctx)) goto l811;
|
|
}
|
|
l812:; goto l810;
|
|
l811:; ctx->pos= yypos811; ctx->thunkpos= yythunkpos811;
|
|
} if (!yy_HtmlBlockCloseThead(ctx)) goto l809;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockThead", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l809:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockThead", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseThead(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseThead")); if (!yymatchChar(ctx, '<')) goto l815; if (!yy_Spnl(ctx)) goto l815; if (!yymatchChar(ctx, '/')) goto l815;
|
|
{ int yypos816= ctx->pos, yythunkpos816= ctx->thunkpos; if (!yymatchString(ctx, "thead")) goto l817; goto l816;
|
|
l817:; ctx->pos= yypos816; ctx->thunkpos= yythunkpos816; if (!yymatchString(ctx, "THEAD")) goto l815;
|
|
}
|
|
l816:; if (!yy_Spnl(ctx)) goto l815; if (!yymatchChar(ctx, '>')) goto l815;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseThead", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l815:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseThead", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenThead(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenThead")); if (!yymatchChar(ctx, '<')) goto l818; if (!yy_Spnl(ctx)) goto l818;
|
|
{ int yypos819= ctx->pos, yythunkpos819= ctx->thunkpos; if (!yymatchString(ctx, "thead")) goto l820; goto l819;
|
|
l820:; ctx->pos= yypos819; ctx->thunkpos= yythunkpos819; if (!yymatchString(ctx, "THEAD")) goto l818;
|
|
}
|
|
l819:; if (!yy_Spnl(ctx)) goto l818;
|
|
l821:;
|
|
{ int yypos822= ctx->pos, yythunkpos822= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l822; goto l821;
|
|
l822:; ctx->pos= yypos822; ctx->thunkpos= yythunkpos822;
|
|
} if (!yymatchChar(ctx, '>')) goto l818;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenThead", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l818:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenThead", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockTh(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockTh")); if (!yy_HtmlBlockOpenTh(ctx)) goto l823;
|
|
l824:;
|
|
{ int yypos825= ctx->pos, yythunkpos825= ctx->thunkpos;
|
|
{ int yypos826= ctx->pos, yythunkpos826= ctx->thunkpos; if (!yy_HtmlBlockTh(ctx)) goto l827; goto l826;
|
|
l827:; ctx->pos= yypos826; ctx->thunkpos= yythunkpos826;
|
|
{ int yypos828= ctx->pos, yythunkpos828= ctx->thunkpos; if (!yy_HtmlBlockCloseTh(ctx)) goto l828; goto l825;
|
|
l828:; ctx->pos= yypos828; ctx->thunkpos= yythunkpos828;
|
|
} if (!yymatchDot(ctx)) goto l825;
|
|
}
|
|
l826:; goto l824;
|
|
l825:; ctx->pos= yypos825; ctx->thunkpos= yythunkpos825;
|
|
} if (!yy_HtmlBlockCloseTh(ctx)) goto l823;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockTh", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l823:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockTh", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseTh(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseTh")); if (!yymatchChar(ctx, '<')) goto l829; if (!yy_Spnl(ctx)) goto l829; if (!yymatchChar(ctx, '/')) goto l829;
|
|
{ int yypos830= ctx->pos, yythunkpos830= ctx->thunkpos; if (!yymatchString(ctx, "th")) goto l831; goto l830;
|
|
l831:; ctx->pos= yypos830; ctx->thunkpos= yythunkpos830; if (!yymatchString(ctx, "TH")) goto l829;
|
|
}
|
|
l830:; if (!yy_Spnl(ctx)) goto l829; if (!yymatchChar(ctx, '>')) goto l829;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseTh", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l829:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseTh", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenTh(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenTh")); if (!yymatchChar(ctx, '<')) goto l832; if (!yy_Spnl(ctx)) goto l832;
|
|
{ int yypos833= ctx->pos, yythunkpos833= ctx->thunkpos; if (!yymatchString(ctx, "th")) goto l834; goto l833;
|
|
l834:; ctx->pos= yypos833; ctx->thunkpos= yythunkpos833; if (!yymatchString(ctx, "TH")) goto l832;
|
|
}
|
|
l833:; if (!yy_Spnl(ctx)) goto l832;
|
|
l835:;
|
|
{ int yypos836= ctx->pos, yythunkpos836= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l836; goto l835;
|
|
l836:; ctx->pos= yypos836; ctx->thunkpos= yythunkpos836;
|
|
} if (!yymatchChar(ctx, '>')) goto l832;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenTh", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l832:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenTh", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockTfoot(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockTfoot")); if (!yy_HtmlBlockOpenTfoot(ctx)) goto l837;
|
|
l838:;
|
|
{ int yypos839= ctx->pos, yythunkpos839= ctx->thunkpos;
|
|
{ int yypos840= ctx->pos, yythunkpos840= ctx->thunkpos; if (!yy_HtmlBlockTfoot(ctx)) goto l841; goto l840;
|
|
l841:; ctx->pos= yypos840; ctx->thunkpos= yythunkpos840;
|
|
{ int yypos842= ctx->pos, yythunkpos842= ctx->thunkpos; if (!yy_HtmlBlockCloseTfoot(ctx)) goto l842; goto l839;
|
|
l842:; ctx->pos= yypos842; ctx->thunkpos= yythunkpos842;
|
|
} if (!yymatchDot(ctx)) goto l839;
|
|
}
|
|
l840:; goto l838;
|
|
l839:; ctx->pos= yypos839; ctx->thunkpos= yythunkpos839;
|
|
} if (!yy_HtmlBlockCloseTfoot(ctx)) goto l837;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockTfoot", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l837:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockTfoot", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseTfoot(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseTfoot")); if (!yymatchChar(ctx, '<')) goto l843; if (!yy_Spnl(ctx)) goto l843; if (!yymatchChar(ctx, '/')) goto l843;
|
|
{ int yypos844= ctx->pos, yythunkpos844= ctx->thunkpos; if (!yymatchString(ctx, "tfoot")) goto l845; goto l844;
|
|
l845:; ctx->pos= yypos844; ctx->thunkpos= yythunkpos844; if (!yymatchString(ctx, "TFOOT")) goto l843;
|
|
}
|
|
l844:; if (!yy_Spnl(ctx)) goto l843; if (!yymatchChar(ctx, '>')) goto l843;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseTfoot", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l843:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseTfoot", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenTfoot(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenTfoot")); if (!yymatchChar(ctx, '<')) goto l846; if (!yy_Spnl(ctx)) goto l846;
|
|
{ int yypos847= ctx->pos, yythunkpos847= ctx->thunkpos; if (!yymatchString(ctx, "tfoot")) goto l848; goto l847;
|
|
l848:; ctx->pos= yypos847; ctx->thunkpos= yythunkpos847; if (!yymatchString(ctx, "TFOOT")) goto l846;
|
|
}
|
|
l847:; if (!yy_Spnl(ctx)) goto l846;
|
|
l849:;
|
|
{ int yypos850= ctx->pos, yythunkpos850= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l850; goto l849;
|
|
l850:; ctx->pos= yypos850; ctx->thunkpos= yythunkpos850;
|
|
} if (!yymatchChar(ctx, '>')) goto l846;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenTfoot", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l846:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenTfoot", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockTd(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockTd")); if (!yy_HtmlBlockOpenTd(ctx)) goto l851;
|
|
l852:;
|
|
{ int yypos853= ctx->pos, yythunkpos853= ctx->thunkpos;
|
|
{ int yypos854= ctx->pos, yythunkpos854= ctx->thunkpos; if (!yy_HtmlBlockTd(ctx)) goto l855; goto l854;
|
|
l855:; ctx->pos= yypos854; ctx->thunkpos= yythunkpos854;
|
|
{ int yypos856= ctx->pos, yythunkpos856= ctx->thunkpos; if (!yy_HtmlBlockCloseTd(ctx)) goto l856; goto l853;
|
|
l856:; ctx->pos= yypos856; ctx->thunkpos= yythunkpos856;
|
|
} if (!yymatchDot(ctx)) goto l853;
|
|
}
|
|
l854:; goto l852;
|
|
l853:; ctx->pos= yypos853; ctx->thunkpos= yythunkpos853;
|
|
} if (!yy_HtmlBlockCloseTd(ctx)) goto l851;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockTd", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l851:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockTd", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseTd(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseTd")); if (!yymatchChar(ctx, '<')) goto l857; if (!yy_Spnl(ctx)) goto l857; if (!yymatchChar(ctx, '/')) goto l857;
|
|
{ int yypos858= ctx->pos, yythunkpos858= ctx->thunkpos; if (!yymatchString(ctx, "td")) goto l859; goto l858;
|
|
l859:; ctx->pos= yypos858; ctx->thunkpos= yythunkpos858; if (!yymatchString(ctx, "TD")) goto l857;
|
|
}
|
|
l858:; if (!yy_Spnl(ctx)) goto l857; if (!yymatchChar(ctx, '>')) goto l857;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseTd", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l857:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseTd", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenTd(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenTd")); if (!yymatchChar(ctx, '<')) goto l860; if (!yy_Spnl(ctx)) goto l860;
|
|
{ int yypos861= ctx->pos, yythunkpos861= ctx->thunkpos; if (!yymatchString(ctx, "td")) goto l862; goto l861;
|
|
l862:; ctx->pos= yypos861; ctx->thunkpos= yythunkpos861; if (!yymatchString(ctx, "TD")) goto l860;
|
|
}
|
|
l861:; if (!yy_Spnl(ctx)) goto l860;
|
|
l863:;
|
|
{ int yypos864= ctx->pos, yythunkpos864= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l864; goto l863;
|
|
l864:; ctx->pos= yypos864; ctx->thunkpos= yythunkpos864;
|
|
} if (!yymatchChar(ctx, '>')) goto l860;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenTd", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l860:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenTd", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockTbody(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockTbody")); if (!yy_HtmlBlockOpenTbody(ctx)) goto l865;
|
|
l866:;
|
|
{ int yypos867= ctx->pos, yythunkpos867= ctx->thunkpos;
|
|
{ int yypos868= ctx->pos, yythunkpos868= ctx->thunkpos; if (!yy_HtmlBlockTbody(ctx)) goto l869; goto l868;
|
|
l869:; ctx->pos= yypos868; ctx->thunkpos= yythunkpos868;
|
|
{ int yypos870= ctx->pos, yythunkpos870= ctx->thunkpos; if (!yy_HtmlBlockCloseTbody(ctx)) goto l870; goto l867;
|
|
l870:; ctx->pos= yypos870; ctx->thunkpos= yythunkpos870;
|
|
} if (!yymatchDot(ctx)) goto l867;
|
|
}
|
|
l868:; goto l866;
|
|
l867:; ctx->pos= yypos867; ctx->thunkpos= yythunkpos867;
|
|
} if (!yy_HtmlBlockCloseTbody(ctx)) goto l865;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockTbody", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l865:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockTbody", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseTbody(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseTbody")); if (!yymatchChar(ctx, '<')) goto l871; if (!yy_Spnl(ctx)) goto l871; if (!yymatchChar(ctx, '/')) goto l871;
|
|
{ int yypos872= ctx->pos, yythunkpos872= ctx->thunkpos; if (!yymatchString(ctx, "tbody")) goto l873; goto l872;
|
|
l873:; ctx->pos= yypos872; ctx->thunkpos= yythunkpos872; if (!yymatchString(ctx, "TBODY")) goto l871;
|
|
}
|
|
l872:; if (!yy_Spnl(ctx)) goto l871; if (!yymatchChar(ctx, '>')) goto l871;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseTbody", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l871:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseTbody", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenTbody(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenTbody")); if (!yymatchChar(ctx, '<')) goto l874; if (!yy_Spnl(ctx)) goto l874;
|
|
{ int yypos875= ctx->pos, yythunkpos875= ctx->thunkpos; if (!yymatchString(ctx, "tbody")) goto l876; goto l875;
|
|
l876:; ctx->pos= yypos875; ctx->thunkpos= yythunkpos875; if (!yymatchString(ctx, "TBODY")) goto l874;
|
|
}
|
|
l875:; if (!yy_Spnl(ctx)) goto l874;
|
|
l877:;
|
|
{ int yypos878= ctx->pos, yythunkpos878= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l878; goto l877;
|
|
l878:; ctx->pos= yypos878; ctx->thunkpos= yythunkpos878;
|
|
} if (!yymatchChar(ctx, '>')) goto l874;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenTbody", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l874:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenTbody", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockLi(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockLi")); if (!yy_HtmlBlockOpenLi(ctx)) goto l879;
|
|
l880:;
|
|
{ int yypos881= ctx->pos, yythunkpos881= ctx->thunkpos;
|
|
{ int yypos882= ctx->pos, yythunkpos882= ctx->thunkpos; if (!yy_HtmlBlockLi(ctx)) goto l883; goto l882;
|
|
l883:; ctx->pos= yypos882; ctx->thunkpos= yythunkpos882;
|
|
{ int yypos884= ctx->pos, yythunkpos884= ctx->thunkpos; if (!yy_HtmlBlockCloseLi(ctx)) goto l884; goto l881;
|
|
l884:; ctx->pos= yypos884; ctx->thunkpos= yythunkpos884;
|
|
} if (!yymatchDot(ctx)) goto l881;
|
|
}
|
|
l882:; goto l880;
|
|
l881:; ctx->pos= yypos881; ctx->thunkpos= yythunkpos881;
|
|
} if (!yy_HtmlBlockCloseLi(ctx)) goto l879;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockLi", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l879:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockLi", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseLi(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseLi")); if (!yymatchChar(ctx, '<')) goto l885; if (!yy_Spnl(ctx)) goto l885; if (!yymatchChar(ctx, '/')) goto l885;
|
|
{ int yypos886= ctx->pos, yythunkpos886= ctx->thunkpos; if (!yymatchString(ctx, "li")) goto l887; goto l886;
|
|
l887:; ctx->pos= yypos886; ctx->thunkpos= yythunkpos886; if (!yymatchString(ctx, "LI")) goto l885;
|
|
}
|
|
l886:; if (!yy_Spnl(ctx)) goto l885; if (!yymatchChar(ctx, '>')) goto l885;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseLi", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l885:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseLi", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenLi(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenLi")); if (!yymatchChar(ctx, '<')) goto l888; if (!yy_Spnl(ctx)) goto l888;
|
|
{ int yypos889= ctx->pos, yythunkpos889= ctx->thunkpos; if (!yymatchString(ctx, "li")) goto l890; goto l889;
|
|
l890:; ctx->pos= yypos889; ctx->thunkpos= yythunkpos889; if (!yymatchString(ctx, "LI")) goto l888;
|
|
}
|
|
l889:; if (!yy_Spnl(ctx)) goto l888;
|
|
l891:;
|
|
{ int yypos892= ctx->pos, yythunkpos892= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l892; goto l891;
|
|
l892:; ctx->pos= yypos892; ctx->thunkpos= yythunkpos892;
|
|
} if (!yymatchChar(ctx, '>')) goto l888;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenLi", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l888:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenLi", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockFrameset(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockFrameset")); if (!yy_HtmlBlockOpenFrameset(ctx)) goto l893;
|
|
l894:;
|
|
{ int yypos895= ctx->pos, yythunkpos895= ctx->thunkpos;
|
|
{ int yypos896= ctx->pos, yythunkpos896= ctx->thunkpos; if (!yy_HtmlBlockFrameset(ctx)) goto l897; goto l896;
|
|
l897:; ctx->pos= yypos896; ctx->thunkpos= yythunkpos896;
|
|
{ int yypos898= ctx->pos, yythunkpos898= ctx->thunkpos; if (!yy_HtmlBlockCloseFrameset(ctx)) goto l898; goto l895;
|
|
l898:; ctx->pos= yypos898; ctx->thunkpos= yythunkpos898;
|
|
} if (!yymatchDot(ctx)) goto l895;
|
|
}
|
|
l896:; goto l894;
|
|
l895:; ctx->pos= yypos895; ctx->thunkpos= yythunkpos895;
|
|
} if (!yy_HtmlBlockCloseFrameset(ctx)) goto l893;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockFrameset", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l893:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockFrameset", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseFrameset(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseFrameset")); if (!yymatchChar(ctx, '<')) goto l899; if (!yy_Spnl(ctx)) goto l899; if (!yymatchChar(ctx, '/')) goto l899;
|
|
{ int yypos900= ctx->pos, yythunkpos900= ctx->thunkpos; if (!yymatchString(ctx, "frameset")) goto l901; goto l900;
|
|
l901:; ctx->pos= yypos900; ctx->thunkpos= yythunkpos900; if (!yymatchString(ctx, "FRAMESET")) goto l899;
|
|
}
|
|
l900:; if (!yy_Spnl(ctx)) goto l899; if (!yymatchChar(ctx, '>')) goto l899;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseFrameset", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l899:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseFrameset", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenFrameset(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenFrameset")); if (!yymatchChar(ctx, '<')) goto l902; if (!yy_Spnl(ctx)) goto l902;
|
|
{ int yypos903= ctx->pos, yythunkpos903= ctx->thunkpos; if (!yymatchString(ctx, "frameset")) goto l904; goto l903;
|
|
l904:; ctx->pos= yypos903; ctx->thunkpos= yythunkpos903; if (!yymatchString(ctx, "FRAMESET")) goto l902;
|
|
}
|
|
l903:; if (!yy_Spnl(ctx)) goto l902;
|
|
l905:;
|
|
{ int yypos906= ctx->pos, yythunkpos906= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l906; goto l905;
|
|
l906:; ctx->pos= yypos906; ctx->thunkpos= yythunkpos906;
|
|
} if (!yymatchChar(ctx, '>')) goto l902;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenFrameset", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l902:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenFrameset", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockDt(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockDt")); if (!yy_HtmlBlockOpenDt(ctx)) goto l907;
|
|
l908:;
|
|
{ int yypos909= ctx->pos, yythunkpos909= ctx->thunkpos;
|
|
{ int yypos910= ctx->pos, yythunkpos910= ctx->thunkpos; if (!yy_HtmlBlockDt(ctx)) goto l911; goto l910;
|
|
l911:; ctx->pos= yypos910; ctx->thunkpos= yythunkpos910;
|
|
{ int yypos912= ctx->pos, yythunkpos912= ctx->thunkpos; if (!yy_HtmlBlockCloseDt(ctx)) goto l912; goto l909;
|
|
l912:; ctx->pos= yypos912; ctx->thunkpos= yythunkpos912;
|
|
} if (!yymatchDot(ctx)) goto l909;
|
|
}
|
|
l910:; goto l908;
|
|
l909:; ctx->pos= yypos909; ctx->thunkpos= yythunkpos909;
|
|
} if (!yy_HtmlBlockCloseDt(ctx)) goto l907;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockDt", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l907:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockDt", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseDt(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseDt")); if (!yymatchChar(ctx, '<')) goto l913; if (!yy_Spnl(ctx)) goto l913; if (!yymatchChar(ctx, '/')) goto l913;
|
|
{ int yypos914= ctx->pos, yythunkpos914= ctx->thunkpos; if (!yymatchString(ctx, "dt")) goto l915; goto l914;
|
|
l915:; ctx->pos= yypos914; ctx->thunkpos= yythunkpos914; if (!yymatchString(ctx, "DT")) goto l913;
|
|
}
|
|
l914:; if (!yy_Spnl(ctx)) goto l913; if (!yymatchChar(ctx, '>')) goto l913;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseDt", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l913:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseDt", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenDt(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenDt")); if (!yymatchChar(ctx, '<')) goto l916; if (!yy_Spnl(ctx)) goto l916;
|
|
{ int yypos917= ctx->pos, yythunkpos917= ctx->thunkpos; if (!yymatchString(ctx, "dt")) goto l918; goto l917;
|
|
l918:; ctx->pos= yypos917; ctx->thunkpos= yythunkpos917; if (!yymatchString(ctx, "DT")) goto l916;
|
|
}
|
|
l917:; if (!yy_Spnl(ctx)) goto l916;
|
|
l919:;
|
|
{ int yypos920= ctx->pos, yythunkpos920= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l920; goto l919;
|
|
l920:; ctx->pos= yypos920; ctx->thunkpos= yythunkpos920;
|
|
} if (!yymatchChar(ctx, '>')) goto l916;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenDt", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l916:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenDt", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockDd(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockDd")); if (!yy_HtmlBlockOpenDd(ctx)) goto l921;
|
|
l922:;
|
|
{ int yypos923= ctx->pos, yythunkpos923= ctx->thunkpos;
|
|
{ int yypos924= ctx->pos, yythunkpos924= ctx->thunkpos; if (!yy_HtmlBlockDd(ctx)) goto l925; goto l924;
|
|
l925:; ctx->pos= yypos924; ctx->thunkpos= yythunkpos924;
|
|
{ int yypos926= ctx->pos, yythunkpos926= ctx->thunkpos; if (!yy_HtmlBlockCloseDd(ctx)) goto l926; goto l923;
|
|
l926:; ctx->pos= yypos926; ctx->thunkpos= yythunkpos926;
|
|
} if (!yymatchDot(ctx)) goto l923;
|
|
}
|
|
l924:; goto l922;
|
|
l923:; ctx->pos= yypos923; ctx->thunkpos= yythunkpos923;
|
|
} if (!yy_HtmlBlockCloseDd(ctx)) goto l921;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockDd", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l921:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockDd", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseDd(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseDd")); if (!yymatchChar(ctx, '<')) goto l927; if (!yy_Spnl(ctx)) goto l927; if (!yymatchChar(ctx, '/')) goto l927;
|
|
{ int yypos928= ctx->pos, yythunkpos928= ctx->thunkpos; if (!yymatchString(ctx, "dd")) goto l929; goto l928;
|
|
l929:; ctx->pos= yypos928; ctx->thunkpos= yythunkpos928; if (!yymatchString(ctx, "DD")) goto l927;
|
|
}
|
|
l928:; if (!yy_Spnl(ctx)) goto l927; if (!yymatchChar(ctx, '>')) goto l927;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseDd", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l927:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseDd", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenDd(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenDd")); if (!yymatchChar(ctx, '<')) goto l930; if (!yy_Spnl(ctx)) goto l930;
|
|
{ int yypos931= ctx->pos, yythunkpos931= ctx->thunkpos; if (!yymatchString(ctx, "dd")) goto l932; goto l931;
|
|
l932:; ctx->pos= yypos931; ctx->thunkpos= yythunkpos931; if (!yymatchString(ctx, "DD")) goto l930;
|
|
}
|
|
l931:; if (!yy_Spnl(ctx)) goto l930;
|
|
l933:;
|
|
{ int yypos934= ctx->pos, yythunkpos934= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l934; goto l933;
|
|
l934:; ctx->pos= yypos934; ctx->thunkpos= yythunkpos934;
|
|
} if (!yymatchChar(ctx, '>')) goto l930;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenDd", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l930:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenDd", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockUl(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockUl")); if (!yy_HtmlBlockOpenUl(ctx)) goto l935;
|
|
l936:;
|
|
{ int yypos937= ctx->pos, yythunkpos937= ctx->thunkpos;
|
|
{ int yypos938= ctx->pos, yythunkpos938= ctx->thunkpos; if (!yy_HtmlBlockUl(ctx)) goto l939; goto l938;
|
|
l939:; ctx->pos= yypos938; ctx->thunkpos= yythunkpos938;
|
|
{ int yypos940= ctx->pos, yythunkpos940= ctx->thunkpos; if (!yy_HtmlBlockCloseUl(ctx)) goto l940; goto l937;
|
|
l940:; ctx->pos= yypos940; ctx->thunkpos= yythunkpos940;
|
|
} if (!yymatchDot(ctx)) goto l937;
|
|
}
|
|
l938:; goto l936;
|
|
l937:; ctx->pos= yypos937; ctx->thunkpos= yythunkpos937;
|
|
} if (!yy_HtmlBlockCloseUl(ctx)) goto l935;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockUl", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l935:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockUl", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseUl(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseUl")); if (!yymatchChar(ctx, '<')) goto l941; if (!yy_Spnl(ctx)) goto l941; if (!yymatchChar(ctx, '/')) goto l941;
|
|
{ int yypos942= ctx->pos, yythunkpos942= ctx->thunkpos; if (!yymatchString(ctx, "ul")) goto l943; goto l942;
|
|
l943:; ctx->pos= yypos942; ctx->thunkpos= yythunkpos942; if (!yymatchString(ctx, "UL")) goto l941;
|
|
}
|
|
l942:; if (!yy_Spnl(ctx)) goto l941; if (!yymatchChar(ctx, '>')) goto l941;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseUl", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l941:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseUl", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenUl(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenUl")); if (!yymatchChar(ctx, '<')) goto l944; if (!yy_Spnl(ctx)) goto l944;
|
|
{ int yypos945= ctx->pos, yythunkpos945= ctx->thunkpos; if (!yymatchString(ctx, "ul")) goto l946; goto l945;
|
|
l946:; ctx->pos= yypos945; ctx->thunkpos= yythunkpos945; if (!yymatchString(ctx, "UL")) goto l944;
|
|
}
|
|
l945:; if (!yy_Spnl(ctx)) goto l944;
|
|
l947:;
|
|
{ int yypos948= ctx->pos, yythunkpos948= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l948; goto l947;
|
|
l948:; ctx->pos= yypos948; ctx->thunkpos= yythunkpos948;
|
|
} if (!yymatchChar(ctx, '>')) goto l944;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenUl", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l944:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenUl", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockTable(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockTable")); if (!yy_HtmlBlockOpenTable(ctx)) goto l949;
|
|
l950:;
|
|
{ int yypos951= ctx->pos, yythunkpos951= ctx->thunkpos;
|
|
{ int yypos952= ctx->pos, yythunkpos952= ctx->thunkpos; if (!yy_HtmlBlockTable(ctx)) goto l953; goto l952;
|
|
l953:; ctx->pos= yypos952; ctx->thunkpos= yythunkpos952;
|
|
{ int yypos954= ctx->pos, yythunkpos954= ctx->thunkpos; if (!yy_HtmlBlockCloseTable(ctx)) goto l954; goto l951;
|
|
l954:; ctx->pos= yypos954; ctx->thunkpos= yythunkpos954;
|
|
} if (!yymatchDot(ctx)) goto l951;
|
|
}
|
|
l952:; goto l950;
|
|
l951:; ctx->pos= yypos951; ctx->thunkpos= yythunkpos951;
|
|
} if (!yy_HtmlBlockCloseTable(ctx)) goto l949;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockTable", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l949:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockTable", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseTable(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseTable")); if (!yymatchChar(ctx, '<')) goto l955; if (!yy_Spnl(ctx)) goto l955; if (!yymatchChar(ctx, '/')) goto l955;
|
|
{ int yypos956= ctx->pos, yythunkpos956= ctx->thunkpos; if (!yymatchString(ctx, "table")) goto l957; goto l956;
|
|
l957:; ctx->pos= yypos956; ctx->thunkpos= yythunkpos956; if (!yymatchString(ctx, "TABLE")) goto l955;
|
|
}
|
|
l956:; if (!yy_Spnl(ctx)) goto l955; if (!yymatchChar(ctx, '>')) goto l955;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseTable", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l955:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseTable", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenTable(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenTable")); if (!yymatchChar(ctx, '<')) goto l958; if (!yy_Spnl(ctx)) goto l958;
|
|
{ int yypos959= ctx->pos, yythunkpos959= ctx->thunkpos; if (!yymatchString(ctx, "table")) goto l960; goto l959;
|
|
l960:; ctx->pos= yypos959; ctx->thunkpos= yythunkpos959; if (!yymatchString(ctx, "TABLE")) goto l958;
|
|
}
|
|
l959:; if (!yy_Spnl(ctx)) goto l958;
|
|
l961:;
|
|
{ int yypos962= ctx->pos, yythunkpos962= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l962; goto l961;
|
|
l962:; ctx->pos= yypos962; ctx->thunkpos= yythunkpos962;
|
|
} if (!yymatchChar(ctx, '>')) goto l958;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenTable", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l958:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenTable", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockPre(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockPre")); if (!yy_HtmlBlockOpenPre(ctx)) goto l963;
|
|
l964:;
|
|
{ int yypos965= ctx->pos, yythunkpos965= ctx->thunkpos;
|
|
{ int yypos966= ctx->pos, yythunkpos966= ctx->thunkpos; if (!yy_HtmlBlockPre(ctx)) goto l967; goto l966;
|
|
l967:; ctx->pos= yypos966; ctx->thunkpos= yythunkpos966;
|
|
{ int yypos968= ctx->pos, yythunkpos968= ctx->thunkpos; if (!yy_HtmlBlockClosePre(ctx)) goto l968; goto l965;
|
|
l968:; ctx->pos= yypos968; ctx->thunkpos= yythunkpos968;
|
|
} if (!yymatchDot(ctx)) goto l965;
|
|
}
|
|
l966:; goto l964;
|
|
l965:; ctx->pos= yypos965; ctx->thunkpos= yythunkpos965;
|
|
} if (!yy_HtmlBlockClosePre(ctx)) goto l963;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockPre", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l963:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockPre", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockClosePre(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockClosePre")); if (!yymatchChar(ctx, '<')) goto l969; if (!yy_Spnl(ctx)) goto l969; if (!yymatchChar(ctx, '/')) goto l969;
|
|
{ int yypos970= ctx->pos, yythunkpos970= ctx->thunkpos; if (!yymatchString(ctx, "pre")) goto l971; goto l970;
|
|
l971:; ctx->pos= yypos970; ctx->thunkpos= yythunkpos970; if (!yymatchString(ctx, "PRE")) goto l969;
|
|
}
|
|
l970:; if (!yy_Spnl(ctx)) goto l969; if (!yymatchChar(ctx, '>')) goto l969;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockClosePre", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l969:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockClosePre", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenPre(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenPre")); if (!yymatchChar(ctx, '<')) goto l972; if (!yy_Spnl(ctx)) goto l972;
|
|
{ int yypos973= ctx->pos, yythunkpos973= ctx->thunkpos; if (!yymatchString(ctx, "pre")) goto l974; goto l973;
|
|
l974:; ctx->pos= yypos973; ctx->thunkpos= yythunkpos973; if (!yymatchString(ctx, "PRE")) goto l972;
|
|
}
|
|
l973:; if (!yy_Spnl(ctx)) goto l972;
|
|
l975:;
|
|
{ int yypos976= ctx->pos, yythunkpos976= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l976; goto l975;
|
|
l976:; ctx->pos= yypos976; ctx->thunkpos= yythunkpos976;
|
|
} if (!yymatchChar(ctx, '>')) goto l972;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenPre", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l972:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenPre", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockP(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockP")); if (!yy_HtmlBlockOpenP(ctx)) goto l977;
|
|
l978:;
|
|
{ int yypos979= ctx->pos, yythunkpos979= ctx->thunkpos;
|
|
{ int yypos980= ctx->pos, yythunkpos980= ctx->thunkpos; if (!yy_HtmlBlockP(ctx)) goto l981; goto l980;
|
|
l981:; ctx->pos= yypos980; ctx->thunkpos= yythunkpos980;
|
|
{ int yypos982= ctx->pos, yythunkpos982= ctx->thunkpos; if (!yy_HtmlBlockCloseP(ctx)) goto l982; goto l979;
|
|
l982:; ctx->pos= yypos982; ctx->thunkpos= yythunkpos982;
|
|
} if (!yymatchDot(ctx)) goto l979;
|
|
}
|
|
l980:; goto l978;
|
|
l979:; ctx->pos= yypos979; ctx->thunkpos= yythunkpos979;
|
|
} if (!yy_HtmlBlockCloseP(ctx)) goto l977;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockP", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l977:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockP", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseP(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseP")); if (!yymatchChar(ctx, '<')) goto l983; if (!yy_Spnl(ctx)) goto l983; if (!yymatchChar(ctx, '/')) goto l983;
|
|
{ int yypos984= ctx->pos, yythunkpos984= ctx->thunkpos; if (!yymatchChar(ctx, 'p')) goto l985; goto l984;
|
|
l985:; ctx->pos= yypos984; ctx->thunkpos= yythunkpos984; if (!yymatchChar(ctx, 'P')) goto l983;
|
|
}
|
|
l984:; if (!yy_Spnl(ctx)) goto l983; if (!yymatchChar(ctx, '>')) goto l983;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseP", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l983:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseP", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenP(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenP")); if (!yymatchChar(ctx, '<')) goto l986; if (!yy_Spnl(ctx)) goto l986;
|
|
{ int yypos987= ctx->pos, yythunkpos987= ctx->thunkpos; if (!yymatchChar(ctx, 'p')) goto l988; goto l987;
|
|
l988:; ctx->pos= yypos987; ctx->thunkpos= yythunkpos987; if (!yymatchChar(ctx, 'P')) goto l986;
|
|
}
|
|
l987:; if (!yy_Spnl(ctx)) goto l986;
|
|
l989:;
|
|
{ int yypos990= ctx->pos, yythunkpos990= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l990; goto l989;
|
|
l990:; ctx->pos= yypos990; ctx->thunkpos= yythunkpos990;
|
|
} if (!yymatchChar(ctx, '>')) goto l986;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenP", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l986:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenP", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOl(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOl")); if (!yy_HtmlBlockOpenOl(ctx)) goto l991;
|
|
l992:;
|
|
{ int yypos993= ctx->pos, yythunkpos993= ctx->thunkpos;
|
|
{ int yypos994= ctx->pos, yythunkpos994= ctx->thunkpos; if (!yy_HtmlBlockOl(ctx)) goto l995; goto l994;
|
|
l995:; ctx->pos= yypos994; ctx->thunkpos= yythunkpos994;
|
|
{ int yypos996= ctx->pos, yythunkpos996= ctx->thunkpos; if (!yy_HtmlBlockCloseOl(ctx)) goto l996; goto l993;
|
|
l996:; ctx->pos= yypos996; ctx->thunkpos= yythunkpos996;
|
|
} if (!yymatchDot(ctx)) goto l993;
|
|
}
|
|
l994:; goto l992;
|
|
l993:; ctx->pos= yypos993; ctx->thunkpos= yythunkpos993;
|
|
} if (!yy_HtmlBlockCloseOl(ctx)) goto l991;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOl", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l991:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOl", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseOl(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseOl")); if (!yymatchChar(ctx, '<')) goto l997; if (!yy_Spnl(ctx)) goto l997; if (!yymatchChar(ctx, '/')) goto l997;
|
|
{ int yypos998= ctx->pos, yythunkpos998= ctx->thunkpos; if (!yymatchString(ctx, "ol")) goto l999; goto l998;
|
|
l999:; ctx->pos= yypos998; ctx->thunkpos= yythunkpos998; if (!yymatchString(ctx, "OL")) goto l997;
|
|
}
|
|
l998:; if (!yy_Spnl(ctx)) goto l997; if (!yymatchChar(ctx, '>')) goto l997;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseOl", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l997:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseOl", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenOl(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenOl")); if (!yymatchChar(ctx, '<')) goto l1000; if (!yy_Spnl(ctx)) goto l1000;
|
|
{ int yypos1001= ctx->pos, yythunkpos1001= ctx->thunkpos; if (!yymatchString(ctx, "ol")) goto l1002; goto l1001;
|
|
l1002:; ctx->pos= yypos1001; ctx->thunkpos= yythunkpos1001; if (!yymatchString(ctx, "OL")) goto l1000;
|
|
}
|
|
l1001:; if (!yy_Spnl(ctx)) goto l1000;
|
|
l1003:;
|
|
{ int yypos1004= ctx->pos, yythunkpos1004= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1004; goto l1003;
|
|
l1004:; ctx->pos= yypos1004; ctx->thunkpos= yythunkpos1004;
|
|
} if (!yymatchChar(ctx, '>')) goto l1000;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenOl", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1000:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenOl", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockNoscript(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockNoscript")); if (!yy_HtmlBlockOpenNoscript(ctx)) goto l1005;
|
|
l1006:;
|
|
{ int yypos1007= ctx->pos, yythunkpos1007= ctx->thunkpos;
|
|
{ int yypos1008= ctx->pos, yythunkpos1008= ctx->thunkpos; if (!yy_HtmlBlockNoscript(ctx)) goto l1009; goto l1008;
|
|
l1009:; ctx->pos= yypos1008; ctx->thunkpos= yythunkpos1008;
|
|
{ int yypos1010= ctx->pos, yythunkpos1010= ctx->thunkpos; if (!yy_HtmlBlockCloseNoscript(ctx)) goto l1010; goto l1007;
|
|
l1010:; ctx->pos= yypos1010; ctx->thunkpos= yythunkpos1010;
|
|
} if (!yymatchDot(ctx)) goto l1007;
|
|
}
|
|
l1008:; goto l1006;
|
|
l1007:; ctx->pos= yypos1007; ctx->thunkpos= yythunkpos1007;
|
|
} if (!yy_HtmlBlockCloseNoscript(ctx)) goto l1005;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockNoscript", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1005:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockNoscript", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseNoscript(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseNoscript")); if (!yymatchChar(ctx, '<')) goto l1011; if (!yy_Spnl(ctx)) goto l1011; if (!yymatchChar(ctx, '/')) goto l1011;
|
|
{ int yypos1012= ctx->pos, yythunkpos1012= ctx->thunkpos; if (!yymatchString(ctx, "noscript")) goto l1013; goto l1012;
|
|
l1013:; ctx->pos= yypos1012; ctx->thunkpos= yythunkpos1012; if (!yymatchString(ctx, "NOSCRIPT")) goto l1011;
|
|
}
|
|
l1012:; if (!yy_Spnl(ctx)) goto l1011; if (!yymatchChar(ctx, '>')) goto l1011;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseNoscript", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1011:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseNoscript", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenNoscript(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenNoscript")); if (!yymatchChar(ctx, '<')) goto l1014; if (!yy_Spnl(ctx)) goto l1014;
|
|
{ int yypos1015= ctx->pos, yythunkpos1015= ctx->thunkpos; if (!yymatchString(ctx, "noscript")) goto l1016; goto l1015;
|
|
l1016:; ctx->pos= yypos1015; ctx->thunkpos= yythunkpos1015; if (!yymatchString(ctx, "NOSCRIPT")) goto l1014;
|
|
}
|
|
l1015:; if (!yy_Spnl(ctx)) goto l1014;
|
|
l1017:;
|
|
{ int yypos1018= ctx->pos, yythunkpos1018= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1018; goto l1017;
|
|
l1018:; ctx->pos= yypos1018; ctx->thunkpos= yythunkpos1018;
|
|
} if (!yymatchChar(ctx, '>')) goto l1014;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenNoscript", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1014:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenNoscript", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockNoframes(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockNoframes")); if (!yy_HtmlBlockOpenNoframes(ctx)) goto l1019;
|
|
l1020:;
|
|
{ int yypos1021= ctx->pos, yythunkpos1021= ctx->thunkpos;
|
|
{ int yypos1022= ctx->pos, yythunkpos1022= ctx->thunkpos; if (!yy_HtmlBlockNoframes(ctx)) goto l1023; goto l1022;
|
|
l1023:; ctx->pos= yypos1022; ctx->thunkpos= yythunkpos1022;
|
|
{ int yypos1024= ctx->pos, yythunkpos1024= ctx->thunkpos; if (!yy_HtmlBlockCloseNoframes(ctx)) goto l1024; goto l1021;
|
|
l1024:; ctx->pos= yypos1024; ctx->thunkpos= yythunkpos1024;
|
|
} if (!yymatchDot(ctx)) goto l1021;
|
|
}
|
|
l1022:; goto l1020;
|
|
l1021:; ctx->pos= yypos1021; ctx->thunkpos= yythunkpos1021;
|
|
} if (!yy_HtmlBlockCloseNoframes(ctx)) goto l1019;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockNoframes", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1019:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockNoframes", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseNoframes(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseNoframes")); if (!yymatchChar(ctx, '<')) goto l1025; if (!yy_Spnl(ctx)) goto l1025; if (!yymatchChar(ctx, '/')) goto l1025;
|
|
{ int yypos1026= ctx->pos, yythunkpos1026= ctx->thunkpos; if (!yymatchString(ctx, "noframes")) goto l1027; goto l1026;
|
|
l1027:; ctx->pos= yypos1026; ctx->thunkpos= yythunkpos1026; if (!yymatchString(ctx, "NOFRAMES")) goto l1025;
|
|
}
|
|
l1026:; if (!yy_Spnl(ctx)) goto l1025; if (!yymatchChar(ctx, '>')) goto l1025;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseNoframes", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1025:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseNoframes", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenNoframes(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenNoframes")); if (!yymatchChar(ctx, '<')) goto l1028; if (!yy_Spnl(ctx)) goto l1028;
|
|
{ int yypos1029= ctx->pos, yythunkpos1029= ctx->thunkpos; if (!yymatchString(ctx, "noframes")) goto l1030; goto l1029;
|
|
l1030:; ctx->pos= yypos1029; ctx->thunkpos= yythunkpos1029; if (!yymatchString(ctx, "NOFRAMES")) goto l1028;
|
|
}
|
|
l1029:; if (!yy_Spnl(ctx)) goto l1028;
|
|
l1031:;
|
|
{ int yypos1032= ctx->pos, yythunkpos1032= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1032; goto l1031;
|
|
l1032:; ctx->pos= yypos1032; ctx->thunkpos= yythunkpos1032;
|
|
} if (!yymatchChar(ctx, '>')) goto l1028;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenNoframes", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1028:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenNoframes", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockMenu(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockMenu")); if (!yy_HtmlBlockOpenMenu(ctx)) goto l1033;
|
|
l1034:;
|
|
{ int yypos1035= ctx->pos, yythunkpos1035= ctx->thunkpos;
|
|
{ int yypos1036= ctx->pos, yythunkpos1036= ctx->thunkpos; if (!yy_HtmlBlockMenu(ctx)) goto l1037; goto l1036;
|
|
l1037:; ctx->pos= yypos1036; ctx->thunkpos= yythunkpos1036;
|
|
{ int yypos1038= ctx->pos, yythunkpos1038= ctx->thunkpos; if (!yy_HtmlBlockCloseMenu(ctx)) goto l1038; goto l1035;
|
|
l1038:; ctx->pos= yypos1038; ctx->thunkpos= yythunkpos1038;
|
|
} if (!yymatchDot(ctx)) goto l1035;
|
|
}
|
|
l1036:; goto l1034;
|
|
l1035:; ctx->pos= yypos1035; ctx->thunkpos= yythunkpos1035;
|
|
} if (!yy_HtmlBlockCloseMenu(ctx)) goto l1033;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockMenu", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1033:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockMenu", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseMenu(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseMenu")); if (!yymatchChar(ctx, '<')) goto l1039; if (!yy_Spnl(ctx)) goto l1039; if (!yymatchChar(ctx, '/')) goto l1039;
|
|
{ int yypos1040= ctx->pos, yythunkpos1040= ctx->thunkpos; if (!yymatchString(ctx, "menu")) goto l1041; goto l1040;
|
|
l1041:; ctx->pos= yypos1040; ctx->thunkpos= yythunkpos1040; if (!yymatchString(ctx, "MENU")) goto l1039;
|
|
}
|
|
l1040:; if (!yy_Spnl(ctx)) goto l1039; if (!yymatchChar(ctx, '>')) goto l1039;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseMenu", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1039:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseMenu", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenMenu(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenMenu")); if (!yymatchChar(ctx, '<')) goto l1042; if (!yy_Spnl(ctx)) goto l1042;
|
|
{ int yypos1043= ctx->pos, yythunkpos1043= ctx->thunkpos; if (!yymatchString(ctx, "menu")) goto l1044; goto l1043;
|
|
l1044:; ctx->pos= yypos1043; ctx->thunkpos= yythunkpos1043; if (!yymatchString(ctx, "MENU")) goto l1042;
|
|
}
|
|
l1043:; if (!yy_Spnl(ctx)) goto l1042;
|
|
l1045:;
|
|
{ int yypos1046= ctx->pos, yythunkpos1046= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1046; goto l1045;
|
|
l1046:; ctx->pos= yypos1046; ctx->thunkpos= yythunkpos1046;
|
|
} if (!yymatchChar(ctx, '>')) goto l1042;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenMenu", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1042:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenMenu", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockH6(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockH6")); if (!yy_HtmlBlockOpenH6(ctx)) goto l1047;
|
|
l1048:;
|
|
{ int yypos1049= ctx->pos, yythunkpos1049= ctx->thunkpos;
|
|
{ int yypos1050= ctx->pos, yythunkpos1050= ctx->thunkpos; if (!yy_HtmlBlockH6(ctx)) goto l1051; goto l1050;
|
|
l1051:; ctx->pos= yypos1050; ctx->thunkpos= yythunkpos1050;
|
|
{ int yypos1052= ctx->pos, yythunkpos1052= ctx->thunkpos; if (!yy_HtmlBlockCloseH6(ctx)) goto l1052; goto l1049;
|
|
l1052:; ctx->pos= yypos1052; ctx->thunkpos= yythunkpos1052;
|
|
} if (!yymatchDot(ctx)) goto l1049;
|
|
}
|
|
l1050:; goto l1048;
|
|
l1049:; ctx->pos= yypos1049; ctx->thunkpos= yythunkpos1049;
|
|
} if (!yy_HtmlBlockCloseH6(ctx)) goto l1047;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockH6", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1047:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockH6", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseH6(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseH6")); if (!yymatchChar(ctx, '<')) goto l1053; if (!yy_Spnl(ctx)) goto l1053; if (!yymatchChar(ctx, '/')) goto l1053;
|
|
{ int yypos1054= ctx->pos, yythunkpos1054= ctx->thunkpos; if (!yymatchString(ctx, "h6")) goto l1055; goto l1054;
|
|
l1055:; ctx->pos= yypos1054; ctx->thunkpos= yythunkpos1054; if (!yymatchString(ctx, "H6")) goto l1053;
|
|
}
|
|
l1054:; if (!yy_Spnl(ctx)) goto l1053; if (!yymatchChar(ctx, '>')) goto l1053;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseH6", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1053:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseH6", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenH6(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenH6")); if (!yymatchChar(ctx, '<')) goto l1056; if (!yy_Spnl(ctx)) goto l1056;
|
|
{ int yypos1057= ctx->pos, yythunkpos1057= ctx->thunkpos; if (!yymatchString(ctx, "h6")) goto l1058; goto l1057;
|
|
l1058:; ctx->pos= yypos1057; ctx->thunkpos= yythunkpos1057; if (!yymatchString(ctx, "H6")) goto l1056;
|
|
}
|
|
l1057:; if (!yy_Spnl(ctx)) goto l1056;
|
|
l1059:;
|
|
{ int yypos1060= ctx->pos, yythunkpos1060= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1060; goto l1059;
|
|
l1060:; ctx->pos= yypos1060; ctx->thunkpos= yythunkpos1060;
|
|
} if (!yymatchChar(ctx, '>')) goto l1056;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenH6", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1056:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenH6", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockH5(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockH5")); if (!yy_HtmlBlockOpenH5(ctx)) goto l1061;
|
|
l1062:;
|
|
{ int yypos1063= ctx->pos, yythunkpos1063= ctx->thunkpos;
|
|
{ int yypos1064= ctx->pos, yythunkpos1064= ctx->thunkpos; if (!yy_HtmlBlockH5(ctx)) goto l1065; goto l1064;
|
|
l1065:; ctx->pos= yypos1064; ctx->thunkpos= yythunkpos1064;
|
|
{ int yypos1066= ctx->pos, yythunkpos1066= ctx->thunkpos; if (!yy_HtmlBlockCloseH5(ctx)) goto l1066; goto l1063;
|
|
l1066:; ctx->pos= yypos1066; ctx->thunkpos= yythunkpos1066;
|
|
} if (!yymatchDot(ctx)) goto l1063;
|
|
}
|
|
l1064:; goto l1062;
|
|
l1063:; ctx->pos= yypos1063; ctx->thunkpos= yythunkpos1063;
|
|
} if (!yy_HtmlBlockCloseH5(ctx)) goto l1061;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockH5", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1061:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockH5", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseH5(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseH5")); if (!yymatchChar(ctx, '<')) goto l1067; if (!yy_Spnl(ctx)) goto l1067; if (!yymatchChar(ctx, '/')) goto l1067;
|
|
{ int yypos1068= ctx->pos, yythunkpos1068= ctx->thunkpos; if (!yymatchString(ctx, "h5")) goto l1069; goto l1068;
|
|
l1069:; ctx->pos= yypos1068; ctx->thunkpos= yythunkpos1068; if (!yymatchString(ctx, "H5")) goto l1067;
|
|
}
|
|
l1068:; if (!yy_Spnl(ctx)) goto l1067; if (!yymatchChar(ctx, '>')) goto l1067;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseH5", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1067:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseH5", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenH5(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenH5")); if (!yymatchChar(ctx, '<')) goto l1070; if (!yy_Spnl(ctx)) goto l1070;
|
|
{ int yypos1071= ctx->pos, yythunkpos1071= ctx->thunkpos; if (!yymatchString(ctx, "h5")) goto l1072; goto l1071;
|
|
l1072:; ctx->pos= yypos1071; ctx->thunkpos= yythunkpos1071; if (!yymatchString(ctx, "H5")) goto l1070;
|
|
}
|
|
l1071:; if (!yy_Spnl(ctx)) goto l1070;
|
|
l1073:;
|
|
{ int yypos1074= ctx->pos, yythunkpos1074= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1074; goto l1073;
|
|
l1074:; ctx->pos= yypos1074; ctx->thunkpos= yythunkpos1074;
|
|
} if (!yymatchChar(ctx, '>')) goto l1070;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenH5", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1070:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenH5", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockH4(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockH4")); if (!yy_HtmlBlockOpenH4(ctx)) goto l1075;
|
|
l1076:;
|
|
{ int yypos1077= ctx->pos, yythunkpos1077= ctx->thunkpos;
|
|
{ int yypos1078= ctx->pos, yythunkpos1078= ctx->thunkpos; if (!yy_HtmlBlockH4(ctx)) goto l1079; goto l1078;
|
|
l1079:; ctx->pos= yypos1078; ctx->thunkpos= yythunkpos1078;
|
|
{ int yypos1080= ctx->pos, yythunkpos1080= ctx->thunkpos; if (!yy_HtmlBlockCloseH4(ctx)) goto l1080; goto l1077;
|
|
l1080:; ctx->pos= yypos1080; ctx->thunkpos= yythunkpos1080;
|
|
} if (!yymatchDot(ctx)) goto l1077;
|
|
}
|
|
l1078:; goto l1076;
|
|
l1077:; ctx->pos= yypos1077; ctx->thunkpos= yythunkpos1077;
|
|
} if (!yy_HtmlBlockCloseH4(ctx)) goto l1075;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockH4", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1075:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockH4", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseH4(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseH4")); if (!yymatchChar(ctx, '<')) goto l1081; if (!yy_Spnl(ctx)) goto l1081; if (!yymatchChar(ctx, '/')) goto l1081;
|
|
{ int yypos1082= ctx->pos, yythunkpos1082= ctx->thunkpos; if (!yymatchString(ctx, "h4")) goto l1083; goto l1082;
|
|
l1083:; ctx->pos= yypos1082; ctx->thunkpos= yythunkpos1082; if (!yymatchString(ctx, "H4")) goto l1081;
|
|
}
|
|
l1082:; if (!yy_Spnl(ctx)) goto l1081; if (!yymatchChar(ctx, '>')) goto l1081;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseH4", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1081:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseH4", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenH4(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenH4")); if (!yymatchChar(ctx, '<')) goto l1084; if (!yy_Spnl(ctx)) goto l1084;
|
|
{ int yypos1085= ctx->pos, yythunkpos1085= ctx->thunkpos; if (!yymatchString(ctx, "h4")) goto l1086; goto l1085;
|
|
l1086:; ctx->pos= yypos1085; ctx->thunkpos= yythunkpos1085; if (!yymatchString(ctx, "H4")) goto l1084;
|
|
}
|
|
l1085:; if (!yy_Spnl(ctx)) goto l1084;
|
|
l1087:;
|
|
{ int yypos1088= ctx->pos, yythunkpos1088= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1088; goto l1087;
|
|
l1088:; ctx->pos= yypos1088; ctx->thunkpos= yythunkpos1088;
|
|
} if (!yymatchChar(ctx, '>')) goto l1084;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenH4", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1084:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenH4", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockH3(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockH3")); if (!yy_HtmlBlockOpenH3(ctx)) goto l1089;
|
|
l1090:;
|
|
{ int yypos1091= ctx->pos, yythunkpos1091= ctx->thunkpos;
|
|
{ int yypos1092= ctx->pos, yythunkpos1092= ctx->thunkpos; if (!yy_HtmlBlockH3(ctx)) goto l1093; goto l1092;
|
|
l1093:; ctx->pos= yypos1092; ctx->thunkpos= yythunkpos1092;
|
|
{ int yypos1094= ctx->pos, yythunkpos1094= ctx->thunkpos; if (!yy_HtmlBlockCloseH3(ctx)) goto l1094; goto l1091;
|
|
l1094:; ctx->pos= yypos1094; ctx->thunkpos= yythunkpos1094;
|
|
} if (!yymatchDot(ctx)) goto l1091;
|
|
}
|
|
l1092:; goto l1090;
|
|
l1091:; ctx->pos= yypos1091; ctx->thunkpos= yythunkpos1091;
|
|
} if (!yy_HtmlBlockCloseH3(ctx)) goto l1089;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockH3", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1089:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockH3", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseH3(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseH3")); if (!yymatchChar(ctx, '<')) goto l1095; if (!yy_Spnl(ctx)) goto l1095; if (!yymatchChar(ctx, '/')) goto l1095;
|
|
{ int yypos1096= ctx->pos, yythunkpos1096= ctx->thunkpos; if (!yymatchString(ctx, "h3")) goto l1097; goto l1096;
|
|
l1097:; ctx->pos= yypos1096; ctx->thunkpos= yythunkpos1096; if (!yymatchString(ctx, "H3")) goto l1095;
|
|
}
|
|
l1096:; if (!yy_Spnl(ctx)) goto l1095; if (!yymatchChar(ctx, '>')) goto l1095;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseH3", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1095:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseH3", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenH3(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenH3")); if (!yymatchChar(ctx, '<')) goto l1098; if (!yy_Spnl(ctx)) goto l1098;
|
|
{ int yypos1099= ctx->pos, yythunkpos1099= ctx->thunkpos; if (!yymatchString(ctx, "h3")) goto l1100; goto l1099;
|
|
l1100:; ctx->pos= yypos1099; ctx->thunkpos= yythunkpos1099; if (!yymatchString(ctx, "H3")) goto l1098;
|
|
}
|
|
l1099:; if (!yy_Spnl(ctx)) goto l1098;
|
|
l1101:;
|
|
{ int yypos1102= ctx->pos, yythunkpos1102= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1102; goto l1101;
|
|
l1102:; ctx->pos= yypos1102; ctx->thunkpos= yythunkpos1102;
|
|
} if (!yymatchChar(ctx, '>')) goto l1098;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenH3", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1098:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenH3", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockH2(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockH2")); if (!yy_HtmlBlockOpenH2(ctx)) goto l1103;
|
|
l1104:;
|
|
{ int yypos1105= ctx->pos, yythunkpos1105= ctx->thunkpos;
|
|
{ int yypos1106= ctx->pos, yythunkpos1106= ctx->thunkpos; if (!yy_HtmlBlockH2(ctx)) goto l1107; goto l1106;
|
|
l1107:; ctx->pos= yypos1106; ctx->thunkpos= yythunkpos1106;
|
|
{ int yypos1108= ctx->pos, yythunkpos1108= ctx->thunkpos; if (!yy_HtmlBlockCloseH2(ctx)) goto l1108; goto l1105;
|
|
l1108:; ctx->pos= yypos1108; ctx->thunkpos= yythunkpos1108;
|
|
} if (!yymatchDot(ctx)) goto l1105;
|
|
}
|
|
l1106:; goto l1104;
|
|
l1105:; ctx->pos= yypos1105; ctx->thunkpos= yythunkpos1105;
|
|
} if (!yy_HtmlBlockCloseH2(ctx)) goto l1103;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockH2", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1103:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockH2", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseH2(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseH2")); if (!yymatchChar(ctx, '<')) goto l1109; if (!yy_Spnl(ctx)) goto l1109; if (!yymatchChar(ctx, '/')) goto l1109;
|
|
{ int yypos1110= ctx->pos, yythunkpos1110= ctx->thunkpos; if (!yymatchString(ctx, "h2")) goto l1111; goto l1110;
|
|
l1111:; ctx->pos= yypos1110; ctx->thunkpos= yythunkpos1110; if (!yymatchString(ctx, "H2")) goto l1109;
|
|
}
|
|
l1110:; if (!yy_Spnl(ctx)) goto l1109; if (!yymatchChar(ctx, '>')) goto l1109;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseH2", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1109:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseH2", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenH2(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenH2")); if (!yymatchChar(ctx, '<')) goto l1112; if (!yy_Spnl(ctx)) goto l1112;
|
|
{ int yypos1113= ctx->pos, yythunkpos1113= ctx->thunkpos; if (!yymatchString(ctx, "h2")) goto l1114; goto l1113;
|
|
l1114:; ctx->pos= yypos1113; ctx->thunkpos= yythunkpos1113; if (!yymatchString(ctx, "H2")) goto l1112;
|
|
}
|
|
l1113:; if (!yy_Spnl(ctx)) goto l1112;
|
|
l1115:;
|
|
{ int yypos1116= ctx->pos, yythunkpos1116= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1116; goto l1115;
|
|
l1116:; ctx->pos= yypos1116; ctx->thunkpos= yythunkpos1116;
|
|
} if (!yymatchChar(ctx, '>')) goto l1112;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenH2", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1112:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenH2", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockH1(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockH1")); if (!yy_HtmlBlockOpenH1(ctx)) goto l1117;
|
|
l1118:;
|
|
{ int yypos1119= ctx->pos, yythunkpos1119= ctx->thunkpos;
|
|
{ int yypos1120= ctx->pos, yythunkpos1120= ctx->thunkpos; if (!yy_HtmlBlockH1(ctx)) goto l1121; goto l1120;
|
|
l1121:; ctx->pos= yypos1120; ctx->thunkpos= yythunkpos1120;
|
|
{ int yypos1122= ctx->pos, yythunkpos1122= ctx->thunkpos; if (!yy_HtmlBlockCloseH1(ctx)) goto l1122; goto l1119;
|
|
l1122:; ctx->pos= yypos1122; ctx->thunkpos= yythunkpos1122;
|
|
} if (!yymatchDot(ctx)) goto l1119;
|
|
}
|
|
l1120:; goto l1118;
|
|
l1119:; ctx->pos= yypos1119; ctx->thunkpos= yythunkpos1119;
|
|
} if (!yy_HtmlBlockCloseH1(ctx)) goto l1117;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockH1", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1117:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockH1", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseH1(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseH1")); if (!yymatchChar(ctx, '<')) goto l1123; if (!yy_Spnl(ctx)) goto l1123; if (!yymatchChar(ctx, '/')) goto l1123;
|
|
{ int yypos1124= ctx->pos, yythunkpos1124= ctx->thunkpos; if (!yymatchString(ctx, "h1")) goto l1125; goto l1124;
|
|
l1125:; ctx->pos= yypos1124; ctx->thunkpos= yythunkpos1124; if (!yymatchString(ctx, "H1")) goto l1123;
|
|
}
|
|
l1124:; if (!yy_Spnl(ctx)) goto l1123; if (!yymatchChar(ctx, '>')) goto l1123;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseH1", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1123:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseH1", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenH1(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenH1")); if (!yymatchChar(ctx, '<')) goto l1126; if (!yy_Spnl(ctx)) goto l1126;
|
|
{ int yypos1127= ctx->pos, yythunkpos1127= ctx->thunkpos; if (!yymatchString(ctx, "h1")) goto l1128; goto l1127;
|
|
l1128:; ctx->pos= yypos1127; ctx->thunkpos= yythunkpos1127; if (!yymatchString(ctx, "H1")) goto l1126;
|
|
}
|
|
l1127:; if (!yy_Spnl(ctx)) goto l1126;
|
|
l1129:;
|
|
{ int yypos1130= ctx->pos, yythunkpos1130= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1130; goto l1129;
|
|
l1130:; ctx->pos= yypos1130; ctx->thunkpos= yythunkpos1130;
|
|
} if (!yymatchChar(ctx, '>')) goto l1126;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenH1", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1126:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenH1", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockForm(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockForm")); if (!yy_HtmlBlockOpenForm(ctx)) goto l1131;
|
|
l1132:;
|
|
{ int yypos1133= ctx->pos, yythunkpos1133= ctx->thunkpos;
|
|
{ int yypos1134= ctx->pos, yythunkpos1134= ctx->thunkpos; if (!yy_HtmlBlockForm(ctx)) goto l1135; goto l1134;
|
|
l1135:; ctx->pos= yypos1134; ctx->thunkpos= yythunkpos1134;
|
|
{ int yypos1136= ctx->pos, yythunkpos1136= ctx->thunkpos; if (!yy_HtmlBlockCloseForm(ctx)) goto l1136; goto l1133;
|
|
l1136:; ctx->pos= yypos1136; ctx->thunkpos= yythunkpos1136;
|
|
} if (!yymatchDot(ctx)) goto l1133;
|
|
}
|
|
l1134:; goto l1132;
|
|
l1133:; ctx->pos= yypos1133; ctx->thunkpos= yythunkpos1133;
|
|
} if (!yy_HtmlBlockCloseForm(ctx)) goto l1131;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockForm", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1131:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockForm", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseForm(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseForm")); if (!yymatchChar(ctx, '<')) goto l1137; if (!yy_Spnl(ctx)) goto l1137; if (!yymatchChar(ctx, '/')) goto l1137;
|
|
{ int yypos1138= ctx->pos, yythunkpos1138= ctx->thunkpos; if (!yymatchString(ctx, "form")) goto l1139; goto l1138;
|
|
l1139:; ctx->pos= yypos1138; ctx->thunkpos= yythunkpos1138; if (!yymatchString(ctx, "FORM")) goto l1137;
|
|
}
|
|
l1138:; if (!yy_Spnl(ctx)) goto l1137; if (!yymatchChar(ctx, '>')) goto l1137;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseForm", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1137:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseForm", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenForm(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenForm")); if (!yymatchChar(ctx, '<')) goto l1140; if (!yy_Spnl(ctx)) goto l1140;
|
|
{ int yypos1141= ctx->pos, yythunkpos1141= ctx->thunkpos; if (!yymatchString(ctx, "form")) goto l1142; goto l1141;
|
|
l1142:; ctx->pos= yypos1141; ctx->thunkpos= yythunkpos1141; if (!yymatchString(ctx, "FORM")) goto l1140;
|
|
}
|
|
l1141:; if (!yy_Spnl(ctx)) goto l1140;
|
|
l1143:;
|
|
{ int yypos1144= ctx->pos, yythunkpos1144= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1144; goto l1143;
|
|
l1144:; ctx->pos= yypos1144; ctx->thunkpos= yythunkpos1144;
|
|
} if (!yymatchChar(ctx, '>')) goto l1140;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenForm", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1140:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenForm", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockFieldset(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockFieldset")); if (!yy_HtmlBlockOpenFieldset(ctx)) goto l1145;
|
|
l1146:;
|
|
{ int yypos1147= ctx->pos, yythunkpos1147= ctx->thunkpos;
|
|
{ int yypos1148= ctx->pos, yythunkpos1148= ctx->thunkpos; if (!yy_HtmlBlockFieldset(ctx)) goto l1149; goto l1148;
|
|
l1149:; ctx->pos= yypos1148; ctx->thunkpos= yythunkpos1148;
|
|
{ int yypos1150= ctx->pos, yythunkpos1150= ctx->thunkpos; if (!yy_HtmlBlockCloseFieldset(ctx)) goto l1150; goto l1147;
|
|
l1150:; ctx->pos= yypos1150; ctx->thunkpos= yythunkpos1150;
|
|
} if (!yymatchDot(ctx)) goto l1147;
|
|
}
|
|
l1148:; goto l1146;
|
|
l1147:; ctx->pos= yypos1147; ctx->thunkpos= yythunkpos1147;
|
|
} if (!yy_HtmlBlockCloseFieldset(ctx)) goto l1145;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockFieldset", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1145:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockFieldset", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseFieldset(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseFieldset")); if (!yymatchChar(ctx, '<')) goto l1151; if (!yy_Spnl(ctx)) goto l1151; if (!yymatchChar(ctx, '/')) goto l1151;
|
|
{ int yypos1152= ctx->pos, yythunkpos1152= ctx->thunkpos; if (!yymatchString(ctx, "fieldset")) goto l1153; goto l1152;
|
|
l1153:; ctx->pos= yypos1152; ctx->thunkpos= yythunkpos1152; if (!yymatchString(ctx, "FIELDSET")) goto l1151;
|
|
}
|
|
l1152:; if (!yy_Spnl(ctx)) goto l1151; if (!yymatchChar(ctx, '>')) goto l1151;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseFieldset", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1151:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseFieldset", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenFieldset(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenFieldset")); if (!yymatchChar(ctx, '<')) goto l1154; if (!yy_Spnl(ctx)) goto l1154;
|
|
{ int yypos1155= ctx->pos, yythunkpos1155= ctx->thunkpos; if (!yymatchString(ctx, "fieldset")) goto l1156; goto l1155;
|
|
l1156:; ctx->pos= yypos1155; ctx->thunkpos= yythunkpos1155; if (!yymatchString(ctx, "FIELDSET")) goto l1154;
|
|
}
|
|
l1155:; if (!yy_Spnl(ctx)) goto l1154;
|
|
l1157:;
|
|
{ int yypos1158= ctx->pos, yythunkpos1158= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1158; goto l1157;
|
|
l1158:; ctx->pos= yypos1158; ctx->thunkpos= yythunkpos1158;
|
|
} if (!yymatchChar(ctx, '>')) goto l1154;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenFieldset", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1154:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenFieldset", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockDl(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockDl")); if (!yy_HtmlBlockOpenDl(ctx)) goto l1159;
|
|
l1160:;
|
|
{ int yypos1161= ctx->pos, yythunkpos1161= ctx->thunkpos;
|
|
{ int yypos1162= ctx->pos, yythunkpos1162= ctx->thunkpos; if (!yy_HtmlBlockDl(ctx)) goto l1163; goto l1162;
|
|
l1163:; ctx->pos= yypos1162; ctx->thunkpos= yythunkpos1162;
|
|
{ int yypos1164= ctx->pos, yythunkpos1164= ctx->thunkpos; if (!yy_HtmlBlockCloseDl(ctx)) goto l1164; goto l1161;
|
|
l1164:; ctx->pos= yypos1164; ctx->thunkpos= yythunkpos1164;
|
|
} if (!yymatchDot(ctx)) goto l1161;
|
|
}
|
|
l1162:; goto l1160;
|
|
l1161:; ctx->pos= yypos1161; ctx->thunkpos= yythunkpos1161;
|
|
} if (!yy_HtmlBlockCloseDl(ctx)) goto l1159;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockDl", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1159:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockDl", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseDl(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseDl")); if (!yymatchChar(ctx, '<')) goto l1165; if (!yy_Spnl(ctx)) goto l1165; if (!yymatchChar(ctx, '/')) goto l1165;
|
|
{ int yypos1166= ctx->pos, yythunkpos1166= ctx->thunkpos; if (!yymatchString(ctx, "dl")) goto l1167; goto l1166;
|
|
l1167:; ctx->pos= yypos1166; ctx->thunkpos= yythunkpos1166; if (!yymatchString(ctx, "DL")) goto l1165;
|
|
}
|
|
l1166:; if (!yy_Spnl(ctx)) goto l1165; if (!yymatchChar(ctx, '>')) goto l1165;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseDl", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1165:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseDl", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenDl(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenDl")); if (!yymatchChar(ctx, '<')) goto l1168; if (!yy_Spnl(ctx)) goto l1168;
|
|
{ int yypos1169= ctx->pos, yythunkpos1169= ctx->thunkpos; if (!yymatchString(ctx, "dl")) goto l1170; goto l1169;
|
|
l1170:; ctx->pos= yypos1169; ctx->thunkpos= yythunkpos1169; if (!yymatchString(ctx, "DL")) goto l1168;
|
|
}
|
|
l1169:; if (!yy_Spnl(ctx)) goto l1168;
|
|
l1171:;
|
|
{ int yypos1172= ctx->pos, yythunkpos1172= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1172; goto l1171;
|
|
l1172:; ctx->pos= yypos1172; ctx->thunkpos= yythunkpos1172;
|
|
} if (!yymatchChar(ctx, '>')) goto l1168;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenDl", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1168:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenDl", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockDiv(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockDiv")); if (!yy_HtmlBlockOpenDiv(ctx)) goto l1173;
|
|
l1174:;
|
|
{ int yypos1175= ctx->pos, yythunkpos1175= ctx->thunkpos;
|
|
{ int yypos1176= ctx->pos, yythunkpos1176= ctx->thunkpos; if (!yy_HtmlBlockDiv(ctx)) goto l1177; goto l1176;
|
|
l1177:; ctx->pos= yypos1176; ctx->thunkpos= yythunkpos1176;
|
|
{ int yypos1178= ctx->pos, yythunkpos1178= ctx->thunkpos; if (!yy_HtmlBlockCloseDiv(ctx)) goto l1178; goto l1175;
|
|
l1178:; ctx->pos= yypos1178; ctx->thunkpos= yythunkpos1178;
|
|
} if (!yymatchDot(ctx)) goto l1175;
|
|
}
|
|
l1176:; goto l1174;
|
|
l1175:; ctx->pos= yypos1175; ctx->thunkpos= yythunkpos1175;
|
|
} if (!yy_HtmlBlockCloseDiv(ctx)) goto l1173;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockDiv", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1173:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockDiv", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseDiv(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseDiv")); if (!yymatchChar(ctx, '<')) goto l1179; if (!yy_Spnl(ctx)) goto l1179; if (!yymatchChar(ctx, '/')) goto l1179;
|
|
{ int yypos1180= ctx->pos, yythunkpos1180= ctx->thunkpos; if (!yymatchString(ctx, "div")) goto l1181; goto l1180;
|
|
l1181:; ctx->pos= yypos1180; ctx->thunkpos= yythunkpos1180; if (!yymatchString(ctx, "DIV")) goto l1179;
|
|
}
|
|
l1180:; if (!yy_Spnl(ctx)) goto l1179; if (!yymatchChar(ctx, '>')) goto l1179;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseDiv", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1179:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseDiv", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenDiv(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenDiv")); if (!yymatchChar(ctx, '<')) goto l1182; if (!yy_Spnl(ctx)) goto l1182;
|
|
{ int yypos1183= ctx->pos, yythunkpos1183= ctx->thunkpos; if (!yymatchString(ctx, "div")) goto l1184; goto l1183;
|
|
l1184:; ctx->pos= yypos1183; ctx->thunkpos= yythunkpos1183; if (!yymatchString(ctx, "DIV")) goto l1182;
|
|
}
|
|
l1183:; if (!yy_Spnl(ctx)) goto l1182;
|
|
l1185:;
|
|
{ int yypos1186= ctx->pos, yythunkpos1186= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1186; goto l1185;
|
|
l1186:; ctx->pos= yypos1186; ctx->thunkpos= yythunkpos1186;
|
|
} if (!yymatchChar(ctx, '>')) goto l1182;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenDiv", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1182:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenDiv", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockDir(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockDir")); if (!yy_HtmlBlockOpenDir(ctx)) goto l1187;
|
|
l1188:;
|
|
{ int yypos1189= ctx->pos, yythunkpos1189= ctx->thunkpos;
|
|
{ int yypos1190= ctx->pos, yythunkpos1190= ctx->thunkpos; if (!yy_HtmlBlockDir(ctx)) goto l1191; goto l1190;
|
|
l1191:; ctx->pos= yypos1190; ctx->thunkpos= yythunkpos1190;
|
|
{ int yypos1192= ctx->pos, yythunkpos1192= ctx->thunkpos; if (!yy_HtmlBlockCloseDir(ctx)) goto l1192; goto l1189;
|
|
l1192:; ctx->pos= yypos1192; ctx->thunkpos= yythunkpos1192;
|
|
} if (!yymatchDot(ctx)) goto l1189;
|
|
}
|
|
l1190:; goto l1188;
|
|
l1189:; ctx->pos= yypos1189; ctx->thunkpos= yythunkpos1189;
|
|
} if (!yy_HtmlBlockCloseDir(ctx)) goto l1187;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockDir", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1187:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockDir", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseDir(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseDir")); if (!yymatchChar(ctx, '<')) goto l1193; if (!yy_Spnl(ctx)) goto l1193; if (!yymatchChar(ctx, '/')) goto l1193;
|
|
{ int yypos1194= ctx->pos, yythunkpos1194= ctx->thunkpos; if (!yymatchString(ctx, "dir")) goto l1195; goto l1194;
|
|
l1195:; ctx->pos= yypos1194; ctx->thunkpos= yythunkpos1194; if (!yymatchString(ctx, "DIR")) goto l1193;
|
|
}
|
|
l1194:; if (!yy_Spnl(ctx)) goto l1193; if (!yymatchChar(ctx, '>')) goto l1193;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseDir", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1193:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseDir", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenDir(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenDir")); if (!yymatchChar(ctx, '<')) goto l1196; if (!yy_Spnl(ctx)) goto l1196;
|
|
{ int yypos1197= ctx->pos, yythunkpos1197= ctx->thunkpos; if (!yymatchString(ctx, "dir")) goto l1198; goto l1197;
|
|
l1198:; ctx->pos= yypos1197; ctx->thunkpos= yythunkpos1197; if (!yymatchString(ctx, "DIR")) goto l1196;
|
|
}
|
|
l1197:; if (!yy_Spnl(ctx)) goto l1196;
|
|
l1199:;
|
|
{ int yypos1200= ctx->pos, yythunkpos1200= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1200; goto l1199;
|
|
l1200:; ctx->pos= yypos1200; ctx->thunkpos= yythunkpos1200;
|
|
} if (!yymatchChar(ctx, '>')) goto l1196;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenDir", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1196:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenDir", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCenter(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCenter")); if (!yy_HtmlBlockOpenCenter(ctx)) goto l1201;
|
|
l1202:;
|
|
{ int yypos1203= ctx->pos, yythunkpos1203= ctx->thunkpos;
|
|
{ int yypos1204= ctx->pos, yythunkpos1204= ctx->thunkpos; if (!yy_HtmlBlockCenter(ctx)) goto l1205; goto l1204;
|
|
l1205:; ctx->pos= yypos1204; ctx->thunkpos= yythunkpos1204;
|
|
{ int yypos1206= ctx->pos, yythunkpos1206= ctx->thunkpos; if (!yy_HtmlBlockCloseCenter(ctx)) goto l1206; goto l1203;
|
|
l1206:; ctx->pos= yypos1206; ctx->thunkpos= yythunkpos1206;
|
|
} if (!yymatchDot(ctx)) goto l1203;
|
|
}
|
|
l1204:; goto l1202;
|
|
l1203:; ctx->pos= yypos1203; ctx->thunkpos= yythunkpos1203;
|
|
} if (!yy_HtmlBlockCloseCenter(ctx)) goto l1201;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCenter", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1201:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCenter", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseCenter(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseCenter")); if (!yymatchChar(ctx, '<')) goto l1207; if (!yy_Spnl(ctx)) goto l1207; if (!yymatchChar(ctx, '/')) goto l1207;
|
|
{ int yypos1208= ctx->pos, yythunkpos1208= ctx->thunkpos; if (!yymatchString(ctx, "center")) goto l1209; goto l1208;
|
|
l1209:; ctx->pos= yypos1208; ctx->thunkpos= yythunkpos1208; if (!yymatchString(ctx, "CENTER")) goto l1207;
|
|
}
|
|
l1208:; if (!yy_Spnl(ctx)) goto l1207; if (!yymatchChar(ctx, '>')) goto l1207;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseCenter", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1207:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseCenter", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenCenter(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenCenter")); if (!yymatchChar(ctx, '<')) goto l1210; if (!yy_Spnl(ctx)) goto l1210;
|
|
{ int yypos1211= ctx->pos, yythunkpos1211= ctx->thunkpos; if (!yymatchString(ctx, "center")) goto l1212; goto l1211;
|
|
l1212:; ctx->pos= yypos1211; ctx->thunkpos= yythunkpos1211; if (!yymatchString(ctx, "CENTER")) goto l1210;
|
|
}
|
|
l1211:; if (!yy_Spnl(ctx)) goto l1210;
|
|
l1213:;
|
|
{ int yypos1214= ctx->pos, yythunkpos1214= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1214; goto l1213;
|
|
l1214:; ctx->pos= yypos1214; ctx->thunkpos= yythunkpos1214;
|
|
} if (!yymatchChar(ctx, '>')) goto l1210;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenCenter", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1210:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenCenter", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockBlockquote(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockBlockquote")); if (!yy_HtmlBlockOpenBlockquote(ctx)) goto l1215;
|
|
l1216:;
|
|
{ int yypos1217= ctx->pos, yythunkpos1217= ctx->thunkpos;
|
|
{ int yypos1218= ctx->pos, yythunkpos1218= ctx->thunkpos; if (!yy_HtmlBlockBlockquote(ctx)) goto l1219; goto l1218;
|
|
l1219:; ctx->pos= yypos1218; ctx->thunkpos= yythunkpos1218;
|
|
{ int yypos1220= ctx->pos, yythunkpos1220= ctx->thunkpos; if (!yy_HtmlBlockCloseBlockquote(ctx)) goto l1220; goto l1217;
|
|
l1220:; ctx->pos= yypos1220; ctx->thunkpos= yythunkpos1220;
|
|
} if (!yymatchDot(ctx)) goto l1217;
|
|
}
|
|
l1218:; goto l1216;
|
|
l1217:; ctx->pos= yypos1217; ctx->thunkpos= yythunkpos1217;
|
|
} if (!yy_HtmlBlockCloseBlockquote(ctx)) goto l1215;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockBlockquote", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1215:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockBlockquote", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseBlockquote(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseBlockquote")); if (!yymatchChar(ctx, '<')) goto l1221; if (!yy_Spnl(ctx)) goto l1221; if (!yymatchChar(ctx, '/')) goto l1221;
|
|
{ int yypos1222= ctx->pos, yythunkpos1222= ctx->thunkpos; if (!yymatchString(ctx, "blockquote")) goto l1223; goto l1222;
|
|
l1223:; ctx->pos= yypos1222; ctx->thunkpos= yythunkpos1222; if (!yymatchString(ctx, "BLOCKQUOTE")) goto l1221;
|
|
}
|
|
l1222:; if (!yy_Spnl(ctx)) goto l1221; if (!yymatchChar(ctx, '>')) goto l1221;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseBlockquote", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1221:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseBlockquote", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenBlockquote(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenBlockquote")); if (!yymatchChar(ctx, '<')) goto l1224; if (!yy_Spnl(ctx)) goto l1224;
|
|
{ int yypos1225= ctx->pos, yythunkpos1225= ctx->thunkpos; if (!yymatchString(ctx, "blockquote")) goto l1226; goto l1225;
|
|
l1226:; ctx->pos= yypos1225; ctx->thunkpos= yythunkpos1225; if (!yymatchString(ctx, "BLOCKQUOTE")) goto l1224;
|
|
}
|
|
l1225:; if (!yy_Spnl(ctx)) goto l1224;
|
|
l1227:;
|
|
{ int yypos1228= ctx->pos, yythunkpos1228= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1228; goto l1227;
|
|
l1228:; ctx->pos= yypos1228; ctx->thunkpos= yythunkpos1228;
|
|
} if (!yymatchChar(ctx, '>')) goto l1224;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenBlockquote", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1224:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenBlockquote", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockAddress(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockAddress")); if (!yy_HtmlBlockOpenAddress(ctx)) goto l1229;
|
|
l1230:;
|
|
{ int yypos1231= ctx->pos, yythunkpos1231= ctx->thunkpos;
|
|
{ int yypos1232= ctx->pos, yythunkpos1232= ctx->thunkpos; if (!yy_HtmlBlockAddress(ctx)) goto l1233; goto l1232;
|
|
l1233:; ctx->pos= yypos1232; ctx->thunkpos= yythunkpos1232;
|
|
{ int yypos1234= ctx->pos, yythunkpos1234= ctx->thunkpos; if (!yy_HtmlBlockCloseAddress(ctx)) goto l1234; goto l1231;
|
|
l1234:; ctx->pos= yypos1234; ctx->thunkpos= yythunkpos1234;
|
|
} if (!yymatchDot(ctx)) goto l1231;
|
|
}
|
|
l1232:; goto l1230;
|
|
l1231:; ctx->pos= yypos1231; ctx->thunkpos= yythunkpos1231;
|
|
} if (!yy_HtmlBlockCloseAddress(ctx)) goto l1229;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockAddress", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1229:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockAddress", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockCloseAddress(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockCloseAddress")); if (!yymatchChar(ctx, '<')) goto l1235; if (!yy_Spnl(ctx)) goto l1235; if (!yymatchChar(ctx, '/')) goto l1235;
|
|
{ int yypos1236= ctx->pos, yythunkpos1236= ctx->thunkpos; if (!yymatchString(ctx, "address")) goto l1237; goto l1236;
|
|
l1237:; ctx->pos= yypos1236; ctx->thunkpos= yythunkpos1236; if (!yymatchString(ctx, "ADDRESS")) goto l1235;
|
|
}
|
|
l1236:; if (!yy_Spnl(ctx)) goto l1235; if (!yymatchChar(ctx, '>')) goto l1235;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockCloseAddress", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1235:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockCloseAddress", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlAttribute(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlAttribute"));
|
|
{ int yypos1241= ctx->pos, yythunkpos1241= ctx->thunkpos; if (!yy_AlphanumericAscii(ctx)) goto l1242; goto l1241;
|
|
l1242:; ctx->pos= yypos1241; ctx->thunkpos= yythunkpos1241; if (!yymatchChar(ctx, '-')) goto l1238;
|
|
}
|
|
l1241:;
|
|
l1239:;
|
|
{ int yypos1240= ctx->pos, yythunkpos1240= ctx->thunkpos;
|
|
{ int yypos1243= ctx->pos, yythunkpos1243= ctx->thunkpos; if (!yy_AlphanumericAscii(ctx)) goto l1244; goto l1243;
|
|
l1244:; ctx->pos= yypos1243; ctx->thunkpos= yythunkpos1243; if (!yymatchChar(ctx, '-')) goto l1240;
|
|
}
|
|
l1243:; goto l1239;
|
|
l1240:; ctx->pos= yypos1240; ctx->thunkpos= yythunkpos1240;
|
|
} if (!yy_Spnl(ctx)) goto l1238;
|
|
{ int yypos1245= ctx->pos, yythunkpos1245= ctx->thunkpos; if (!yymatchChar(ctx, '=')) goto l1245; if (!yy_Spnl(ctx)) goto l1245;
|
|
{ int yypos1247= ctx->pos, yythunkpos1247= ctx->thunkpos; if (!yy_Quoted(ctx)) goto l1248; goto l1247;
|
|
l1248:; ctx->pos= yypos1247; ctx->thunkpos= yythunkpos1247;
|
|
{ int yypos1251= ctx->pos, yythunkpos1251= ctx->thunkpos; if (!yymatchChar(ctx, '>')) goto l1251; goto l1245;
|
|
l1251:; ctx->pos= yypos1251; ctx->thunkpos= yythunkpos1251;
|
|
} if (!yy_Nonspacechar(ctx)) goto l1245;
|
|
l1249:;
|
|
{ int yypos1250= ctx->pos, yythunkpos1250= ctx->thunkpos;
|
|
{ int yypos1252= ctx->pos, yythunkpos1252= ctx->thunkpos; if (!yymatchChar(ctx, '>')) goto l1252; goto l1250;
|
|
l1252:; ctx->pos= yypos1252; ctx->thunkpos= yythunkpos1252;
|
|
} if (!yy_Nonspacechar(ctx)) goto l1250; goto l1249;
|
|
l1250:; ctx->pos= yypos1250; ctx->thunkpos= yythunkpos1250;
|
|
}
|
|
}
|
|
l1247:; goto l1246;
|
|
l1245:; ctx->pos= yypos1245; ctx->thunkpos= yythunkpos1245;
|
|
}
|
|
l1246:; if (!yy_Spnl(ctx)) goto l1238;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlAttribute", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1238:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlAttribute", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Spnl(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Spnl")); if (!yy_Sp(ctx)) goto l1253;
|
|
{ int yypos1254= ctx->pos, yythunkpos1254= ctx->thunkpos; if (!yy_Newline(ctx)) goto l1254; if (!yy_Sp(ctx)) goto l1254; goto l1255;
|
|
l1254:; ctx->pos= yypos1254; ctx->thunkpos= yythunkpos1254;
|
|
}
|
|
l1255:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Spnl", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1253:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Spnl", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlockOpenAddress(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlockOpenAddress")); if (!yymatchChar(ctx, '<')) goto l1256; if (!yy_Spnl(ctx)) goto l1256;
|
|
{ int yypos1257= ctx->pos, yythunkpos1257= ctx->thunkpos; if (!yymatchString(ctx, "address")) goto l1258; goto l1257;
|
|
l1258:; ctx->pos= yypos1257; ctx->thunkpos= yythunkpos1257; if (!yymatchString(ctx, "ADDRESS")) goto l1256;
|
|
}
|
|
l1257:; if (!yy_Spnl(ctx)) goto l1256;
|
|
l1259:;
|
|
{ int yypos1260= ctx->pos, yythunkpos1260= ctx->thunkpos; if (!yy_HtmlAttribute(ctx)) goto l1260; goto l1259;
|
|
l1260:; ctx->pos= yypos1260; ctx->thunkpos= yythunkpos1260;
|
|
} if (!yymatchChar(ctx, '>')) goto l1256;
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlockOpenAddress", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1256:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlockOpenAddress", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_OptionallyIndentedLine(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "OptionallyIndentedLine"));
|
|
{ int yypos1262= ctx->pos, yythunkpos1262= ctx->thunkpos; if (!yy_Indent(ctx)) goto l1262; goto l1263;
|
|
l1262:; ctx->pos= yypos1262; ctx->thunkpos= yythunkpos1262;
|
|
}
|
|
l1263:; if (!yy_Line(ctx)) goto l1261;
|
|
yyprintf((stderr, " ok %s @ %s\n", "OptionallyIndentedLine", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1261:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "OptionallyIndentedLine", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Indent(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Indent"));
|
|
{ int yypos1265= ctx->pos, yythunkpos1265= ctx->thunkpos; if (!yymatchChar(ctx, '\t')) goto l1266; goto l1265;
|
|
l1266:; ctx->pos= yypos1265; ctx->thunkpos= yythunkpos1265; if (!yymatchString(ctx, " ")) goto l1264;
|
|
}
|
|
l1265:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Indent", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1264:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Indent", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_ListBlockLine(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "ListBlockLine"));
|
|
{ int yypos1268= ctx->pos, yythunkpos1268= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1268; goto l1267;
|
|
l1268:; ctx->pos= yypos1268; ctx->thunkpos= yythunkpos1268;
|
|
}
|
|
{ int yypos1269= ctx->pos, yythunkpos1269= ctx->thunkpos;
|
|
{ int yypos1270= ctx->pos, yythunkpos1270= ctx->thunkpos; if (!yy_Indent(ctx)) goto l1270; goto l1271;
|
|
l1270:; ctx->pos= yypos1270; ctx->thunkpos= yythunkpos1270;
|
|
}
|
|
l1271:;
|
|
{ int yypos1272= ctx->pos, yythunkpos1272= ctx->thunkpos; if (!yy_Bullet(ctx)) goto l1273; goto l1272;
|
|
l1273:; ctx->pos= yypos1272; ctx->thunkpos= yythunkpos1272; if (!yy_Enumerator(ctx)) goto l1269;
|
|
}
|
|
l1272:; goto l1267;
|
|
l1269:; ctx->pos= yypos1269; ctx->thunkpos= yythunkpos1269;
|
|
}
|
|
{ int yypos1274= ctx->pos, yythunkpos1274= ctx->thunkpos; if (!yy_HorizontalRule(ctx)) goto l1274; goto l1267;
|
|
l1274:; ctx->pos= yypos1274; ctx->thunkpos= yythunkpos1274;
|
|
} if (!yy_OptionallyIndentedLine(ctx)) goto l1267;
|
|
yyprintf((stderr, " ok %s @ %s\n", "ListBlockLine", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1267:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "ListBlockLine", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_ListContinuationBlock(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "ListContinuationBlock")); if (!yy_StartList(ctx)) goto l1275; yyDo(ctx, yySet, -1, 0); yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l1275;
|
|
l1276:;
|
|
{ int yypos1277= ctx->pos, yythunkpos1277= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1277; goto l1276;
|
|
l1277:; ctx->pos= yypos1277; ctx->thunkpos= yythunkpos1277;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l1275; yyDo(ctx, yy_1_ListContinuationBlock, ctx->begin, ctx->end); if (!yy_Indent(ctx)) goto l1275; if (!yy_ListBlock(ctx)) goto l1275; yyDo(ctx, yy_2_ListContinuationBlock, ctx->begin, ctx->end);
|
|
l1278:;
|
|
{ int yypos1279= ctx->pos, yythunkpos1279= ctx->thunkpos; if (!yy_Indent(ctx)) goto l1279; if (!yy_ListBlock(ctx)) goto l1279; yyDo(ctx, yy_2_ListContinuationBlock, ctx->begin, ctx->end); goto l1278;
|
|
l1279:; ctx->pos= yypos1279; ctx->thunkpos= yythunkpos1279;
|
|
} yyDo(ctx, yy_3_ListContinuationBlock, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "ListContinuationBlock", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l1275:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "ListContinuationBlock", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_ListBlock(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "ListBlock")); if (!yy_StartList(ctx)) goto l1280; yyDo(ctx, yySet, -1, 0);
|
|
{ int yypos1281= ctx->pos, yythunkpos1281= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1281; goto l1280;
|
|
l1281:; ctx->pos= yypos1281; ctx->thunkpos= yythunkpos1281;
|
|
} if (!yy_Line(ctx)) goto l1280; yyDo(ctx, yy_1_ListBlock, ctx->begin, ctx->end);
|
|
l1282:;
|
|
{ int yypos1283= ctx->pos, yythunkpos1283= ctx->thunkpos; if (!yy_ListBlockLine(ctx)) goto l1283; yyDo(ctx, yy_2_ListBlock, ctx->begin, ctx->end); goto l1282;
|
|
l1283:; ctx->pos= yypos1283; ctx->thunkpos= yythunkpos1283;
|
|
} yyDo(ctx, yy_3_ListBlock, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "ListBlock", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l1280:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "ListBlock", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_ListItem(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "ListItem"));
|
|
{ int yypos1285= ctx->pos, yythunkpos1285= ctx->thunkpos; if (!yy_Bullet(ctx)) goto l1286; goto l1285;
|
|
l1286:; ctx->pos= yypos1285; ctx->thunkpos= yythunkpos1285; if (!yy_Enumerator(ctx)) goto l1284;
|
|
}
|
|
l1285:; if (!yy_StartList(ctx)) goto l1284; yyDo(ctx, yySet, -1, 0); if (!yy_ListBlock(ctx)) goto l1284; yyDo(ctx, yy_1_ListItem, ctx->begin, ctx->end);
|
|
l1287:;
|
|
{ int yypos1288= ctx->pos, yythunkpos1288= ctx->thunkpos; if (!yy_ListContinuationBlock(ctx)) goto l1288; yyDo(ctx, yy_2_ListItem, ctx->begin, ctx->end); goto l1287;
|
|
l1288:; ctx->pos= yypos1288; ctx->thunkpos= yythunkpos1288;
|
|
} yyDo(ctx, yy_3_ListItem, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "ListItem", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l1284:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "ListItem", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Enumerator(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Enumerator")); if (!yy_NonindentSpace(ctx)) goto l1289; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\000\000\377\003\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l1289;
|
|
l1290:;
|
|
{ int yypos1291= ctx->pos, yythunkpos1291= ctx->thunkpos; if (!yymatchClass(ctx, (unsigned char *)"\000\000\000\000\000\000\377\003\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000")) goto l1291; goto l1290;
|
|
l1291:; ctx->pos= yypos1291; ctx->thunkpos= yythunkpos1291;
|
|
} if (!yymatchChar(ctx, '.')) goto l1289; if (!yy_Spacechar(ctx)) goto l1289;
|
|
l1292:;
|
|
{ int yypos1293= ctx->pos, yythunkpos1293= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l1293; goto l1292;
|
|
l1293:; ctx->pos= yypos1293; ctx->thunkpos= yythunkpos1293;
|
|
}
|
|
yyprintf((stderr, " ok %s @ %s\n", "Enumerator", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1289:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Enumerator", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_ListItemTight(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "ListItemTight"));
|
|
{ int yypos1295= ctx->pos, yythunkpos1295= ctx->thunkpos; if (!yy_Bullet(ctx)) goto l1296; goto l1295;
|
|
l1296:; ctx->pos= yypos1295; ctx->thunkpos= yythunkpos1295; if (!yy_Enumerator(ctx)) goto l1294;
|
|
}
|
|
l1295:; if (!yy_StartList(ctx)) goto l1294; yyDo(ctx, yySet, -1, 0); if (!yy_ListBlock(ctx)) goto l1294; yyDo(ctx, yy_1_ListItemTight, ctx->begin, ctx->end);
|
|
l1297:;
|
|
{ int yypos1298= ctx->pos, yythunkpos1298= ctx->thunkpos;
|
|
{ int yypos1299= ctx->pos, yythunkpos1299= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1299; goto l1298;
|
|
l1299:; ctx->pos= yypos1299; ctx->thunkpos= yythunkpos1299;
|
|
} if (!yy_ListContinuationBlock(ctx)) goto l1298; yyDo(ctx, yy_2_ListItemTight, ctx->begin, ctx->end); goto l1297;
|
|
l1298:; ctx->pos= yypos1298; ctx->thunkpos= yythunkpos1298;
|
|
}
|
|
{ int yypos1300= ctx->pos, yythunkpos1300= ctx->thunkpos; if (!yy_ListContinuationBlock(ctx)) goto l1300; goto l1294;
|
|
l1300:; ctx->pos= yypos1300; ctx->thunkpos= yythunkpos1300;
|
|
} yyDo(ctx, yy_3_ListItemTight, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "ListItemTight", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l1294:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "ListItemTight", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_ListLoose(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 2, 0);
|
|
yyprintf((stderr, "%s\n", "ListLoose")); if (!yy_StartList(ctx)) goto l1301; yyDo(ctx, yySet, -2, 0); if (!yy_ListItem(ctx)) goto l1301; yyDo(ctx, yySet, -1, 0);
|
|
l1304:;
|
|
{ int yypos1305= ctx->pos, yythunkpos1305= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1305; goto l1304;
|
|
l1305:; ctx->pos= yypos1305; ctx->thunkpos= yythunkpos1305;
|
|
} yyDo(ctx, yy_1_ListLoose, ctx->begin, ctx->end);
|
|
l1302:;
|
|
{ int yypos1303= ctx->pos, yythunkpos1303= ctx->thunkpos; if (!yy_ListItem(ctx)) goto l1303; yyDo(ctx, yySet, -1, 0);
|
|
l1306:;
|
|
{ int yypos1307= ctx->pos, yythunkpos1307= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1307; goto l1306;
|
|
l1307:; ctx->pos= yypos1307; ctx->thunkpos= yythunkpos1307;
|
|
} yyDo(ctx, yy_1_ListLoose, ctx->begin, ctx->end); goto l1302;
|
|
l1303:; ctx->pos= yypos1303; ctx->thunkpos= yythunkpos1303;
|
|
} yyDo(ctx, yy_2_ListLoose, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "ListLoose", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 2, 0);
|
|
return 1;
|
|
l1301:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "ListLoose", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_ListTight(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "ListTight")); if (!yy_StartList(ctx)) goto l1308; yyDo(ctx, yySet, -1, 0); if (!yy_ListItemTight(ctx)) goto l1308; yyDo(ctx, yy_1_ListTight, ctx->begin, ctx->end);
|
|
l1309:;
|
|
{ int yypos1310= ctx->pos, yythunkpos1310= ctx->thunkpos; if (!yy_ListItemTight(ctx)) goto l1310; yyDo(ctx, yy_1_ListTight, ctx->begin, ctx->end); goto l1309;
|
|
l1310:; ctx->pos= yypos1310; ctx->thunkpos= yythunkpos1310;
|
|
}
|
|
l1311:;
|
|
{ int yypos1312= ctx->pos, yythunkpos1312= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1312; goto l1311;
|
|
l1312:; ctx->pos= yypos1312; ctx->thunkpos= yythunkpos1312;
|
|
}
|
|
{ int yypos1313= ctx->pos, yythunkpos1313= ctx->thunkpos;
|
|
{ int yypos1314= ctx->pos, yythunkpos1314= ctx->thunkpos; if (!yy_Bullet(ctx)) goto l1315; goto l1314;
|
|
l1315:; ctx->pos= yypos1314; ctx->thunkpos= yythunkpos1314; if (!yy_Enumerator(ctx)) goto l1313;
|
|
}
|
|
l1314:; goto l1308;
|
|
l1313:; ctx->pos= yypos1313; ctx->thunkpos= yythunkpos1313;
|
|
} yyDo(ctx, yy_2_ListTight, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "ListTight", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l1308:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "ListTight", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Spacechar(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Spacechar"));
|
|
{ int yypos1317= ctx->pos, yythunkpos1317= ctx->thunkpos; if (!yymatchChar(ctx, ' ')) goto l1318; goto l1317;
|
|
l1318:; ctx->pos= yypos1317; ctx->thunkpos= yythunkpos1317; if (!yymatchChar(ctx, '\t')) goto l1316;
|
|
}
|
|
l1317:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Spacechar", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1316:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Spacechar", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Bullet(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Bullet"));
|
|
{ int yypos1320= ctx->pos, yythunkpos1320= ctx->thunkpos; if (!yy_HorizontalRule(ctx)) goto l1320; goto l1319;
|
|
l1320:; ctx->pos= yypos1320; ctx->thunkpos= yythunkpos1320;
|
|
} if (!yy_NonindentSpace(ctx)) goto l1319;
|
|
{ int yypos1321= ctx->pos, yythunkpos1321= ctx->thunkpos; if (!yymatchChar(ctx, '+')) goto l1322; goto l1321;
|
|
l1322:; ctx->pos= yypos1321; ctx->thunkpos= yythunkpos1321; if (!yymatchChar(ctx, '*')) goto l1323; goto l1321;
|
|
l1323:; ctx->pos= yypos1321; ctx->thunkpos= yythunkpos1321; if (!yymatchChar(ctx, '-')) goto l1319;
|
|
}
|
|
l1321:; if (!yy_Spacechar(ctx)) goto l1319;
|
|
l1324:;
|
|
{ int yypos1325= ctx->pos, yythunkpos1325= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l1325; goto l1324;
|
|
l1325:; ctx->pos= yypos1325; ctx->thunkpos= yythunkpos1325;
|
|
}
|
|
yyprintf((stderr, " ok %s @ %s\n", "Bullet", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1319:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Bullet", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_VerbatimChunk(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "VerbatimChunk")); if (!yy_StartList(ctx)) goto l1326; yyDo(ctx, yySet, -1, 0);
|
|
l1327:;
|
|
{ int yypos1328= ctx->pos, yythunkpos1328= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1328; yyDo(ctx, yy_1_VerbatimChunk, ctx->begin, ctx->end); goto l1327;
|
|
l1328:; ctx->pos= yypos1328; ctx->thunkpos= yythunkpos1328;
|
|
} if (!yy_NonblankIndentedLine(ctx)) goto l1326; yyDo(ctx, yy_2_VerbatimChunk, ctx->begin, ctx->end);
|
|
l1329:;
|
|
{ int yypos1330= ctx->pos, yythunkpos1330= ctx->thunkpos; if (!yy_NonblankIndentedLine(ctx)) goto l1330; yyDo(ctx, yy_2_VerbatimChunk, ctx->begin, ctx->end); goto l1329;
|
|
l1330:; ctx->pos= yypos1330; ctx->thunkpos= yythunkpos1330;
|
|
} yyDo(ctx, yy_3_VerbatimChunk, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "VerbatimChunk", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l1326:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "VerbatimChunk", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_IndentedLine(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "IndentedLine")); if (!yy_Indent(ctx)) goto l1331; if (!yy_Line(ctx)) goto l1331;
|
|
yyprintf((stderr, " ok %s @ %s\n", "IndentedLine", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1331:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "IndentedLine", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_NonblankIndentedLine(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "NonblankIndentedLine"));
|
|
{ int yypos1333= ctx->pos, yythunkpos1333= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1333; goto l1332;
|
|
l1333:; ctx->pos= yypos1333; ctx->thunkpos= yythunkpos1333;
|
|
} if (!yy_IndentedLine(ctx)) goto l1332;
|
|
yyprintf((stderr, " ok %s @ %s\n", "NonblankIndentedLine", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1332:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "NonblankIndentedLine", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Line(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Line")); if (!yy_RawLine(ctx)) goto l1334; yyDo(ctx, yy_1_Line, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Line", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1334:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Line", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_BlockQuoteRaw(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "BlockQuoteRaw")); if (!yy_StartList(ctx)) goto l1335; yyDo(ctx, yySet, -1, 0); if (!yymatchChar(ctx, '>')) goto l1335;
|
|
{ int yypos1338= ctx->pos, yythunkpos1338= ctx->thunkpos; if (!yymatchChar(ctx, ' ')) goto l1338; goto l1339;
|
|
l1338:; ctx->pos= yypos1338; ctx->thunkpos= yythunkpos1338;
|
|
}
|
|
l1339:; if (!yy_Line(ctx)) goto l1335; yyDo(ctx, yy_1_BlockQuoteRaw, ctx->begin, ctx->end);
|
|
l1340:;
|
|
{ int yypos1341= ctx->pos, yythunkpos1341= ctx->thunkpos;
|
|
{ int yypos1342= ctx->pos, yythunkpos1342= ctx->thunkpos; if (!yymatchChar(ctx, '>')) goto l1342; goto l1341;
|
|
l1342:; ctx->pos= yypos1342; ctx->thunkpos= yythunkpos1342;
|
|
}
|
|
{ int yypos1343= ctx->pos, yythunkpos1343= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1343; goto l1341;
|
|
l1343:; ctx->pos= yypos1343; ctx->thunkpos= yythunkpos1343;
|
|
} if (!yy_Line(ctx)) goto l1341; yyDo(ctx, yy_2_BlockQuoteRaw, ctx->begin, ctx->end); goto l1340;
|
|
l1341:; ctx->pos= yypos1341; ctx->thunkpos= yythunkpos1341;
|
|
}
|
|
l1344:;
|
|
{ int yypos1345= ctx->pos, yythunkpos1345= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1345; yyDo(ctx, yy_3_BlockQuoteRaw, ctx->begin, ctx->end); goto l1344;
|
|
l1345:; ctx->pos= yypos1345; ctx->thunkpos= yythunkpos1345;
|
|
}
|
|
l1336:;
|
|
{ int yypos1337= ctx->pos, yythunkpos1337= ctx->thunkpos; if (!yymatchChar(ctx, '>')) goto l1337;
|
|
{ int yypos1346= ctx->pos, yythunkpos1346= ctx->thunkpos; if (!yymatchChar(ctx, ' ')) goto l1346; goto l1347;
|
|
l1346:; ctx->pos= yypos1346; ctx->thunkpos= yythunkpos1346;
|
|
}
|
|
l1347:; if (!yy_Line(ctx)) goto l1337; yyDo(ctx, yy_1_BlockQuoteRaw, ctx->begin, ctx->end);
|
|
l1348:;
|
|
{ int yypos1349= ctx->pos, yythunkpos1349= ctx->thunkpos;
|
|
{ int yypos1350= ctx->pos, yythunkpos1350= ctx->thunkpos; if (!yymatchChar(ctx, '>')) goto l1350; goto l1349;
|
|
l1350:; ctx->pos= yypos1350; ctx->thunkpos= yythunkpos1350;
|
|
}
|
|
{ int yypos1351= ctx->pos, yythunkpos1351= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1351; goto l1349;
|
|
l1351:; ctx->pos= yypos1351; ctx->thunkpos= yythunkpos1351;
|
|
} if (!yy_Line(ctx)) goto l1349; yyDo(ctx, yy_2_BlockQuoteRaw, ctx->begin, ctx->end); goto l1348;
|
|
l1349:; ctx->pos= yypos1349; ctx->thunkpos= yythunkpos1349;
|
|
}
|
|
l1352:;
|
|
{ int yypos1353= ctx->pos, yythunkpos1353= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1353; yyDo(ctx, yy_3_BlockQuoteRaw, ctx->begin, ctx->end); goto l1352;
|
|
l1353:; ctx->pos= yypos1353; ctx->thunkpos= yythunkpos1353;
|
|
} goto l1336;
|
|
l1337:; ctx->pos= yypos1337; ctx->thunkpos= yythunkpos1337;
|
|
} yyDo(ctx, yy_4_BlockQuoteRaw, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "BlockQuoteRaw", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l1335:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "BlockQuoteRaw", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Endline(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Endline"));
|
|
{ int yypos1355= ctx->pos, yythunkpos1355= ctx->thunkpos; if (!yy_LineBreak(ctx)) goto l1356; goto l1355;
|
|
l1356:; ctx->pos= yypos1355; ctx->thunkpos= yythunkpos1355; if (!yy_TerminalEndline(ctx)) goto l1357; goto l1355;
|
|
l1357:; ctx->pos= yypos1355; ctx->thunkpos= yythunkpos1355; if (!yy_NormalEndline(ctx)) goto l1354;
|
|
}
|
|
l1355:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Endline", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1354:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Endline", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_RawLine(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "RawLine"));
|
|
{ int yypos1359= ctx->pos, yythunkpos1359= ctx->thunkpos; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l1360;
|
|
l1361:;
|
|
{ int yypos1362= ctx->pos, yythunkpos1362= ctx->thunkpos;
|
|
{ int yypos1363= ctx->pos, yythunkpos1363= ctx->thunkpos; if (!yymatchChar(ctx, '\r')) goto l1363; goto l1362;
|
|
l1363:; ctx->pos= yypos1363; ctx->thunkpos= yythunkpos1363;
|
|
}
|
|
{ int yypos1364= ctx->pos, yythunkpos1364= ctx->thunkpos; if (!yymatchChar(ctx, '\n')) goto l1364; goto l1362;
|
|
l1364:; ctx->pos= yypos1364; ctx->thunkpos= yythunkpos1364;
|
|
} if (!yymatchDot(ctx)) goto l1362; goto l1361;
|
|
l1362:; ctx->pos= yypos1362; ctx->thunkpos= yythunkpos1362;
|
|
} if (!yy_Newline(ctx)) goto l1360; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l1360; goto l1359;
|
|
l1360:; ctx->pos= yypos1359; ctx->thunkpos= yythunkpos1359; yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l1358; if (!yymatchDot(ctx)) goto l1358;
|
|
l1365:;
|
|
{ int yypos1366= ctx->pos, yythunkpos1366= ctx->thunkpos; if (!yymatchDot(ctx)) goto l1366; goto l1365;
|
|
l1366:; ctx->pos= yypos1366; ctx->thunkpos= yythunkpos1366;
|
|
} yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l1358; if (!yy_Eof(ctx)) goto l1358;
|
|
}
|
|
l1359:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "RawLine", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1358:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "RawLine", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_SetextBottom2(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "SetextBottom2")); if (!yymatchChar(ctx, '-')) goto l1367;
|
|
l1368:;
|
|
{ int yypos1369= ctx->pos, yythunkpos1369= ctx->thunkpos; if (!yymatchChar(ctx, '-')) goto l1369; goto l1368;
|
|
l1369:; ctx->pos= yypos1369; ctx->thunkpos= yythunkpos1369;
|
|
} if (!yy_Newline(ctx)) goto l1367;
|
|
yyprintf((stderr, " ok %s @ %s\n", "SetextBottom2", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1367:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "SetextBottom2", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_SetextBottom1(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "SetextBottom1")); if (!yymatchChar(ctx, '=')) goto l1370;
|
|
l1371:;
|
|
{ int yypos1372= ctx->pos, yythunkpos1372= ctx->thunkpos; if (!yymatchChar(ctx, '=')) goto l1372; goto l1371;
|
|
l1372:; ctx->pos= yypos1372; ctx->thunkpos= yythunkpos1372;
|
|
} if (!yy_Newline(ctx)) goto l1370;
|
|
yyprintf((stderr, " ok %s @ %s\n", "SetextBottom1", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1370:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "SetextBottom1", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_SetextHeading2(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "SetextHeading2"));
|
|
{ int yypos1374= ctx->pos, yythunkpos1374= ctx->thunkpos; if (!yy_RawLine(ctx)) goto l1373; if (!yy_SetextBottom2(ctx)) goto l1373; ctx->pos= yypos1374; ctx->thunkpos= yythunkpos1374;
|
|
} if (!yy_StartList(ctx)) goto l1373; yyDo(ctx, yySet, -1, 0);
|
|
{ int yypos1377= ctx->pos, yythunkpos1377= ctx->thunkpos; if (!yy_Endline(ctx)) goto l1377; goto l1373;
|
|
l1377:; ctx->pos= yypos1377; ctx->thunkpos= yythunkpos1377;
|
|
} if (!yy_Inline(ctx)) goto l1373; yyDo(ctx, yy_1_SetextHeading2, ctx->begin, ctx->end);
|
|
l1375:;
|
|
{ int yypos1376= ctx->pos, yythunkpos1376= ctx->thunkpos;
|
|
{ int yypos1378= ctx->pos, yythunkpos1378= ctx->thunkpos; if (!yy_Endline(ctx)) goto l1378; goto l1376;
|
|
l1378:; ctx->pos= yypos1378; ctx->thunkpos= yythunkpos1378;
|
|
} if (!yy_Inline(ctx)) goto l1376; yyDo(ctx, yy_1_SetextHeading2, ctx->begin, ctx->end); goto l1375;
|
|
l1376:; ctx->pos= yypos1376; ctx->thunkpos= yythunkpos1376;
|
|
}
|
|
{ int yypos1379= ctx->pos, yythunkpos1379= ctx->thunkpos; if (!yy_Sp(ctx)) goto l1379; goto l1380;
|
|
l1379:; ctx->pos= yypos1379; ctx->thunkpos= yythunkpos1379;
|
|
}
|
|
l1380:; if (!yy_Newline(ctx)) goto l1373; if (!yy_SetextBottom2(ctx)) goto l1373; yyDo(ctx, yy_2_SetextHeading2, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "SetextHeading2", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l1373:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "SetextHeading2", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_SetextHeading1(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "SetextHeading1"));
|
|
{ int yypos1382= ctx->pos, yythunkpos1382= ctx->thunkpos; if (!yy_RawLine(ctx)) goto l1381; if (!yy_SetextBottom1(ctx)) goto l1381; ctx->pos= yypos1382; ctx->thunkpos= yythunkpos1382;
|
|
} if (!yy_StartList(ctx)) goto l1381; yyDo(ctx, yySet, -1, 0);
|
|
{ int yypos1385= ctx->pos, yythunkpos1385= ctx->thunkpos; if (!yy_Endline(ctx)) goto l1385; goto l1381;
|
|
l1385:; ctx->pos= yypos1385; ctx->thunkpos= yythunkpos1385;
|
|
} if (!yy_Inline(ctx)) goto l1381; yyDo(ctx, yy_1_SetextHeading1, ctx->begin, ctx->end);
|
|
l1383:;
|
|
{ int yypos1384= ctx->pos, yythunkpos1384= ctx->thunkpos;
|
|
{ int yypos1386= ctx->pos, yythunkpos1386= ctx->thunkpos; if (!yy_Endline(ctx)) goto l1386; goto l1384;
|
|
l1386:; ctx->pos= yypos1386; ctx->thunkpos= yythunkpos1386;
|
|
} if (!yy_Inline(ctx)) goto l1384; yyDo(ctx, yy_1_SetextHeading1, ctx->begin, ctx->end); goto l1383;
|
|
l1384:; ctx->pos= yypos1384; ctx->thunkpos= yythunkpos1384;
|
|
}
|
|
{ int yypos1387= ctx->pos, yythunkpos1387= ctx->thunkpos; if (!yy_Sp(ctx)) goto l1387; goto l1388;
|
|
l1387:; ctx->pos= yypos1387; ctx->thunkpos= yythunkpos1387;
|
|
}
|
|
l1388:; if (!yy_Newline(ctx)) goto l1381; if (!yy_SetextBottom1(ctx)) goto l1381; yyDo(ctx, yy_2_SetextHeading1, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "SetextHeading1", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l1381:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "SetextHeading1", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_SetextHeading(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "SetextHeading"));
|
|
{ int yypos1390= ctx->pos, yythunkpos1390= ctx->thunkpos; if (!yy_SetextHeading1(ctx)) goto l1391; goto l1390;
|
|
l1391:; ctx->pos= yypos1390; ctx->thunkpos= yythunkpos1390; if (!yy_SetextHeading2(ctx)) goto l1389;
|
|
}
|
|
l1390:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "SetextHeading", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1389:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "SetextHeading", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_AtxHeading(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 2, 0);
|
|
yyprintf((stderr, "%s\n", "AtxHeading")); if (!yy_AtxStart(ctx)) goto l1392; yyDo(ctx, yySet, -2, 0);
|
|
{ int yypos1393= ctx->pos, yythunkpos1393= ctx->thunkpos; if (!yy_Sp(ctx)) goto l1393; goto l1394;
|
|
l1393:; ctx->pos= yypos1393; ctx->thunkpos= yythunkpos1393;
|
|
}
|
|
l1394:; if (!yy_StartList(ctx)) goto l1392; yyDo(ctx, yySet, -1, 0); if (!yy_AtxInline(ctx)) goto l1392; yyDo(ctx, yy_1_AtxHeading, ctx->begin, ctx->end);
|
|
l1395:;
|
|
{ int yypos1396= ctx->pos, yythunkpos1396= ctx->thunkpos; if (!yy_AtxInline(ctx)) goto l1396; yyDo(ctx, yy_1_AtxHeading, ctx->begin, ctx->end); goto l1395;
|
|
l1396:; ctx->pos= yypos1396; ctx->thunkpos= yythunkpos1396;
|
|
}
|
|
{ int yypos1397= ctx->pos, yythunkpos1397= ctx->thunkpos;
|
|
{ int yypos1399= ctx->pos, yythunkpos1399= ctx->thunkpos; if (!yy_Sp(ctx)) goto l1399; goto l1400;
|
|
l1399:; ctx->pos= yypos1399; ctx->thunkpos= yythunkpos1399;
|
|
}
|
|
l1400:;
|
|
l1401:;
|
|
{ int yypos1402= ctx->pos, yythunkpos1402= ctx->thunkpos; if (!yymatchChar(ctx, '#')) goto l1402; goto l1401;
|
|
l1402:; ctx->pos= yypos1402; ctx->thunkpos= yythunkpos1402;
|
|
} if (!yy_Sp(ctx)) goto l1397; goto l1398;
|
|
l1397:; ctx->pos= yypos1397; ctx->thunkpos= yythunkpos1397;
|
|
}
|
|
l1398:; if (!yy_Newline(ctx)) goto l1392; yyDo(ctx, yy_2_AtxHeading, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "AtxHeading", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 2, 0);
|
|
return 1;
|
|
l1392:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "AtxHeading", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_AtxStart(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "AtxStart")); yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l1403;
|
|
{ int yypos1404= ctx->pos, yythunkpos1404= ctx->thunkpos; if (!yymatchString(ctx, "######")) goto l1405; goto l1404;
|
|
l1405:; ctx->pos= yypos1404; ctx->thunkpos= yythunkpos1404; if (!yymatchString(ctx, "#####")) goto l1406; goto l1404;
|
|
l1406:; ctx->pos= yypos1404; ctx->thunkpos= yythunkpos1404; if (!yymatchString(ctx, "####")) goto l1407; goto l1404;
|
|
l1407:; ctx->pos= yypos1404; ctx->thunkpos= yythunkpos1404; if (!yymatchString(ctx, "###")) goto l1408; goto l1404;
|
|
l1408:; ctx->pos= yypos1404; ctx->thunkpos= yythunkpos1404; if (!yymatchString(ctx, "##")) goto l1409; goto l1404;
|
|
l1409:; ctx->pos= yypos1404; ctx->thunkpos= yythunkpos1404; if (!yymatchChar(ctx, '#')) goto l1403;
|
|
}
|
|
l1404:; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l1403; yyDo(ctx, yy_1_AtxStart, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "AtxStart", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1403:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "AtxStart", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Inline(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Inline"));
|
|
{ int yypos1411= ctx->pos, yythunkpos1411= ctx->thunkpos; if (!yy_Str(ctx)) goto l1412; goto l1411;
|
|
l1412:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_Endline(ctx)) goto l1413; goto l1411;
|
|
l1413:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_UlOrStarLine(ctx)) goto l1414; goto l1411;
|
|
l1414:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_Space(ctx)) goto l1415; goto l1411;
|
|
l1415:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_Strong(ctx)) goto l1416; goto l1411;
|
|
l1416:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_Emph(ctx)) goto l1417; goto l1411;
|
|
l1417:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_Image(ctx)) goto l1418; goto l1411;
|
|
l1418:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_Link(ctx)) goto l1419; goto l1411;
|
|
l1419:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_NoteReference(ctx)) goto l1420; goto l1411;
|
|
l1420:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_InlineNote(ctx)) goto l1421; goto l1411;
|
|
l1421:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_Code(ctx)) goto l1422; goto l1411;
|
|
l1422:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_RawHtml(ctx)) goto l1423; goto l1411;
|
|
l1423:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_Entity(ctx)) goto l1424; goto l1411;
|
|
l1424:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_EscapedChar(ctx)) goto l1425; goto l1411;
|
|
l1425:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_Smart(ctx)) goto l1426; goto l1411;
|
|
l1426:; ctx->pos= yypos1411; ctx->thunkpos= yythunkpos1411; if (!yy_Symbol(ctx)) goto l1410;
|
|
}
|
|
l1411:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Inline", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1410:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Inline", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Sp(yycontext *ctx)
|
|
{
|
|
yyprintf((stderr, "%s\n", "Sp"));
|
|
l1428:;
|
|
{ int yypos1429= ctx->pos, yythunkpos1429= ctx->thunkpos; if (!yy_Spacechar(ctx)) goto l1429; goto l1428;
|
|
l1429:; ctx->pos= yypos1429; ctx->thunkpos= yythunkpos1429;
|
|
}
|
|
yyprintf((stderr, " ok %s @ %s\n", "Sp", ctx->buf+ctx->pos));
|
|
return 1;
|
|
}
|
|
YY_RULE(int) yy_Newline(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Newline"));
|
|
{ int yypos1431= ctx->pos, yythunkpos1431= ctx->thunkpos; if (!yymatchChar(ctx, '\n')) goto l1432; goto l1431;
|
|
l1432:; ctx->pos= yypos1431; ctx->thunkpos= yythunkpos1431; if (!yymatchChar(ctx, '\r')) goto l1430;
|
|
{ int yypos1433= ctx->pos, yythunkpos1433= ctx->thunkpos; if (!yymatchChar(ctx, '\n')) goto l1433; goto l1434;
|
|
l1433:; ctx->pos= yypos1433; ctx->thunkpos= yythunkpos1433;
|
|
}
|
|
l1434:;
|
|
}
|
|
l1431:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Newline", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1430:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Newline", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_AtxInline(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "AtxInline"));
|
|
{ int yypos1436= ctx->pos, yythunkpos1436= ctx->thunkpos; if (!yy_Newline(ctx)) goto l1436; goto l1435;
|
|
l1436:; ctx->pos= yypos1436; ctx->thunkpos= yythunkpos1436;
|
|
}
|
|
{ int yypos1437= ctx->pos, yythunkpos1437= ctx->thunkpos;
|
|
{ int yypos1438= ctx->pos, yythunkpos1438= ctx->thunkpos; if (!yy_Sp(ctx)) goto l1438; goto l1439;
|
|
l1438:; ctx->pos= yypos1438; ctx->thunkpos= yythunkpos1438;
|
|
}
|
|
l1439:;
|
|
l1440:;
|
|
{ int yypos1441= ctx->pos, yythunkpos1441= ctx->thunkpos; if (!yymatchChar(ctx, '#')) goto l1441; goto l1440;
|
|
l1441:; ctx->pos= yypos1441; ctx->thunkpos= yythunkpos1441;
|
|
} if (!yy_Sp(ctx)) goto l1437; if (!yy_Newline(ctx)) goto l1437; goto l1435;
|
|
l1437:; ctx->pos= yypos1437; ctx->thunkpos= yythunkpos1437;
|
|
} if (!yy_Inline(ctx)) goto l1435;
|
|
yyprintf((stderr, " ok %s @ %s\n", "AtxInline", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1435:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "AtxInline", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Inlines(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 2, 0);
|
|
yyprintf((stderr, "%s\n", "Inlines")); if (!yy_StartList(ctx)) goto l1442; yyDo(ctx, yySet, -2, 0);
|
|
{ int yypos1445= ctx->pos, yythunkpos1445= ctx->thunkpos;
|
|
{ int yypos1447= ctx->pos, yythunkpos1447= ctx->thunkpos; if (!yy_Endline(ctx)) goto l1447; goto l1446;
|
|
l1447:; ctx->pos= yypos1447; ctx->thunkpos= yythunkpos1447;
|
|
} if (!yy_Inline(ctx)) goto l1446; yyDo(ctx, yy_1_Inlines, ctx->begin, ctx->end); goto l1445;
|
|
l1446:; ctx->pos= yypos1445; ctx->thunkpos= yythunkpos1445; if (!yy_Endline(ctx)) goto l1442; yyDo(ctx, yySet, -1, 0);
|
|
{ int yypos1448= ctx->pos, yythunkpos1448= ctx->thunkpos; if (!yy_Inline(ctx)) goto l1442; ctx->pos= yypos1448; ctx->thunkpos= yythunkpos1448;
|
|
} yyDo(ctx, yy_2_Inlines, ctx->begin, ctx->end);
|
|
}
|
|
l1445:;
|
|
l1443:;
|
|
{ int yypos1444= ctx->pos, yythunkpos1444= ctx->thunkpos;
|
|
{ int yypos1449= ctx->pos, yythunkpos1449= ctx->thunkpos;
|
|
{ int yypos1451= ctx->pos, yythunkpos1451= ctx->thunkpos; if (!yy_Endline(ctx)) goto l1451; goto l1450;
|
|
l1451:; ctx->pos= yypos1451; ctx->thunkpos= yythunkpos1451;
|
|
} if (!yy_Inline(ctx)) goto l1450; yyDo(ctx, yy_1_Inlines, ctx->begin, ctx->end); goto l1449;
|
|
l1450:; ctx->pos= yypos1449; ctx->thunkpos= yythunkpos1449; if (!yy_Endline(ctx)) goto l1444; yyDo(ctx, yySet, -1, 0);
|
|
{ int yypos1452= ctx->pos, yythunkpos1452= ctx->thunkpos; if (!yy_Inline(ctx)) goto l1444; ctx->pos= yypos1452; ctx->thunkpos= yythunkpos1452;
|
|
} yyDo(ctx, yy_2_Inlines, ctx->begin, ctx->end);
|
|
}
|
|
l1449:; goto l1443;
|
|
l1444:; ctx->pos= yypos1444; ctx->thunkpos= yythunkpos1444;
|
|
}
|
|
{ int yypos1453= ctx->pos, yythunkpos1453= ctx->thunkpos; if (!yy_Endline(ctx)) goto l1453; goto l1454;
|
|
l1453:; ctx->pos= yypos1453; ctx->thunkpos= yythunkpos1453;
|
|
}
|
|
l1454:; yyDo(ctx, yy_3_Inlines, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Inlines", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 2, 0);
|
|
return 1;
|
|
l1442:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Inlines", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_NonindentSpace(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "NonindentSpace"));
|
|
{ int yypos1456= ctx->pos, yythunkpos1456= ctx->thunkpos; if (!yymatchString(ctx, " ")) goto l1457; goto l1456;
|
|
l1457:; ctx->pos= yypos1456; ctx->thunkpos= yythunkpos1456; if (!yymatchString(ctx, " ")) goto l1458; goto l1456;
|
|
l1458:; ctx->pos= yypos1456; ctx->thunkpos= yythunkpos1456; if (!yymatchChar(ctx, ' ')) goto l1459; goto l1456;
|
|
l1459:; ctx->pos= yypos1456; ctx->thunkpos= yythunkpos1456; if (!yymatchString(ctx, "")) goto l1455;
|
|
}
|
|
l1456:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "NonindentSpace", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1455:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "NonindentSpace", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Plain(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "Plain")); if (!yy_Inlines(ctx)) goto l1460; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_Plain, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Plain", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l1460:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Plain", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Para(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "Para")); if (!yy_NonindentSpace(ctx)) goto l1461; if (!yy_Inlines(ctx)) goto l1461; yyDo(ctx, yySet, -1, 0); if (!yy_BlankLine(ctx)) goto l1461;
|
|
l1462:;
|
|
{ int yypos1463= ctx->pos, yythunkpos1463= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1463; goto l1462;
|
|
l1463:; ctx->pos= yypos1463; ctx->thunkpos= yythunkpos1463;
|
|
} yyDo(ctx, yy_1_Para, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Para", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l1461:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Para", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_StyleBlock(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "StyleBlock")); yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l1464; if (!yy_InStyleTags(ctx)) goto l1464; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l1464;
|
|
l1465:;
|
|
{ int yypos1466= ctx->pos, yythunkpos1466= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1466; goto l1465;
|
|
l1466:; ctx->pos= yypos1466; ctx->thunkpos= yythunkpos1466;
|
|
} yyDo(ctx, yy_1_StyleBlock, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "StyleBlock", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1464:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "StyleBlock", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HtmlBlock(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HtmlBlock")); yyText(ctx, ctx->begin, ctx->end); if (!(YY_BEGIN)) goto l1467;
|
|
{ int yypos1468= ctx->pos, yythunkpos1468= ctx->thunkpos; if (!yy_HtmlBlockInTags(ctx)) goto l1469; goto l1468;
|
|
l1469:; ctx->pos= yypos1468; ctx->thunkpos= yythunkpos1468; if (!yy_HtmlComment(ctx)) goto l1470; goto l1468;
|
|
l1470:; ctx->pos= yypos1468; ctx->thunkpos= yythunkpos1468; if (!yy_HtmlBlockSelfClosing(ctx)) goto l1467;
|
|
}
|
|
l1468:; yyText(ctx, ctx->begin, ctx->end); if (!(YY_END)) goto l1467; if (!yy_BlankLine(ctx)) goto l1467;
|
|
l1471:;
|
|
{ int yypos1472= ctx->pos, yythunkpos1472= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1472; goto l1471;
|
|
l1472:; ctx->pos= yypos1472; ctx->thunkpos= yythunkpos1472;
|
|
} yyDo(ctx, yy_1_HtmlBlock, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "HtmlBlock", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1467:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HtmlBlock", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_BulletList(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "BulletList"));
|
|
{ int yypos1474= ctx->pos, yythunkpos1474= ctx->thunkpos; if (!yy_Bullet(ctx)) goto l1473; ctx->pos= yypos1474; ctx->thunkpos= yythunkpos1474;
|
|
}
|
|
{ int yypos1475= ctx->pos, yythunkpos1475= ctx->thunkpos; if (!yy_ListTight(ctx)) goto l1476; goto l1475;
|
|
l1476:; ctx->pos= yypos1475; ctx->thunkpos= yythunkpos1475; if (!yy_ListLoose(ctx)) goto l1473;
|
|
}
|
|
l1475:; yyDo(ctx, yy_1_BulletList, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "BulletList", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1473:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "BulletList", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_OrderedList(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "OrderedList"));
|
|
{ int yypos1478= ctx->pos, yythunkpos1478= ctx->thunkpos; if (!yy_Enumerator(ctx)) goto l1477; ctx->pos= yypos1478; ctx->thunkpos= yythunkpos1478;
|
|
}
|
|
{ int yypos1479= ctx->pos, yythunkpos1479= ctx->thunkpos; if (!yy_ListTight(ctx)) goto l1480; goto l1479;
|
|
l1480:; ctx->pos= yypos1479; ctx->thunkpos= yythunkpos1479; if (!yy_ListLoose(ctx)) goto l1477;
|
|
}
|
|
l1479:; yyDo(ctx, yy_1_OrderedList, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "OrderedList", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1477:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "OrderedList", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Heading(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Heading"));
|
|
{ int yypos1482= ctx->pos, yythunkpos1482= ctx->thunkpos; if (!yy_SetextHeading(ctx)) goto l1483; goto l1482;
|
|
l1483:; ctx->pos= yypos1482; ctx->thunkpos= yythunkpos1482; if (!yy_AtxHeading(ctx)) goto l1481;
|
|
}
|
|
l1482:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Heading", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1481:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Heading", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_HorizontalRule(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "HorizontalRule")); if (!yy_NonindentSpace(ctx)) goto l1484;
|
|
{ int yypos1485= ctx->pos, yythunkpos1485= ctx->thunkpos; if (!yymatchChar(ctx, '*')) goto l1486; if (!yy_Sp(ctx)) goto l1486; if (!yymatchChar(ctx, '*')) goto l1486; if (!yy_Sp(ctx)) goto l1486; if (!yymatchChar(ctx, '*')) goto l1486;
|
|
l1487:;
|
|
{ int yypos1488= ctx->pos, yythunkpos1488= ctx->thunkpos; if (!yy_Sp(ctx)) goto l1488; if (!yymatchChar(ctx, '*')) goto l1488; goto l1487;
|
|
l1488:; ctx->pos= yypos1488; ctx->thunkpos= yythunkpos1488;
|
|
} goto l1485;
|
|
l1486:; ctx->pos= yypos1485; ctx->thunkpos= yythunkpos1485; if (!yymatchChar(ctx, '-')) goto l1489; if (!yy_Sp(ctx)) goto l1489; if (!yymatchChar(ctx, '-')) goto l1489; if (!yy_Sp(ctx)) goto l1489; if (!yymatchChar(ctx, '-')) goto l1489;
|
|
l1490:;
|
|
{ int yypos1491= ctx->pos, yythunkpos1491= ctx->thunkpos; if (!yy_Sp(ctx)) goto l1491; if (!yymatchChar(ctx, '-')) goto l1491; goto l1490;
|
|
l1491:; ctx->pos= yypos1491; ctx->thunkpos= yythunkpos1491;
|
|
} goto l1485;
|
|
l1489:; ctx->pos= yypos1485; ctx->thunkpos= yythunkpos1485; if (!yymatchChar(ctx, '_')) goto l1484; if (!yy_Sp(ctx)) goto l1484; if (!yymatchChar(ctx, '_')) goto l1484; if (!yy_Sp(ctx)) goto l1484; if (!yymatchChar(ctx, '_')) goto l1484;
|
|
l1492:;
|
|
{ int yypos1493= ctx->pos, yythunkpos1493= ctx->thunkpos; if (!yy_Sp(ctx)) goto l1493; if (!yymatchChar(ctx, '_')) goto l1493; goto l1492;
|
|
l1493:; ctx->pos= yypos1493; ctx->thunkpos= yythunkpos1493;
|
|
}
|
|
}
|
|
l1485:; if (!yy_Sp(ctx)) goto l1484; if (!yy_Newline(ctx)) goto l1484; if (!yy_BlankLine(ctx)) goto l1484;
|
|
l1494:;
|
|
{ int yypos1495= ctx->pos, yythunkpos1495= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1495; goto l1494;
|
|
l1495:; ctx->pos= yypos1495; ctx->thunkpos= yythunkpos1495;
|
|
} yyDo(ctx, yy_1_HorizontalRule, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "HorizontalRule", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1484:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "HorizontalRule", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Reference(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 3, 0);
|
|
yyprintf((stderr, "%s\n", "Reference")); if (!yy_NonindentSpace(ctx)) goto l1496;
|
|
{ int yypos1497= ctx->pos, yythunkpos1497= ctx->thunkpos; if (!yymatchString(ctx, "[]")) goto l1497; goto l1496;
|
|
l1497:; ctx->pos= yypos1497; ctx->thunkpos= yythunkpos1497;
|
|
} if (!yy_Label(ctx)) goto l1496; yyDo(ctx, yySet, -3, 0); if (!yymatchChar(ctx, ':')) goto l1496; if (!yy_Spnl(ctx)) goto l1496; if (!yy_RefSrc(ctx)) goto l1496; yyDo(ctx, yySet, -2, 0); if (!yy_RefTitle(ctx)) goto l1496; yyDo(ctx, yySet, -1, 0); if (!yy_BlankLine(ctx)) goto l1496;
|
|
l1498:;
|
|
{ int yypos1499= ctx->pos, yythunkpos1499= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1499; goto l1498;
|
|
l1499:; ctx->pos= yypos1499; ctx->thunkpos= yythunkpos1499;
|
|
} yyDo(ctx, yy_1_Reference, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Reference", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 3, 0);
|
|
return 1;
|
|
l1496:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Reference", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Note(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 2, 0);
|
|
yyprintf((stderr, "%s\n", "Note")); yyText(ctx, ctx->begin, ctx->end); if (!( extension(EXT_NOTES) )) goto l1500; if (!yy_NonindentSpace(ctx)) goto l1500; if (!yy_RawNoteReference(ctx)) goto l1500; yyDo(ctx, yySet, -2, 0); if (!yymatchChar(ctx, ':')) goto l1500; if (!yy_Sp(ctx)) goto l1500; if (!yy_StartList(ctx)) goto l1500; yyDo(ctx, yySet, -1, 0); if (!yy_RawNoteBlock(ctx)) goto l1500; yyDo(ctx, yy_1_Note, ctx->begin, ctx->end);
|
|
l1501:;
|
|
{ int yypos1502= ctx->pos, yythunkpos1502= ctx->thunkpos;
|
|
{ int yypos1503= ctx->pos, yythunkpos1503= ctx->thunkpos; if (!yy_Indent(ctx)) goto l1502; ctx->pos= yypos1503; ctx->thunkpos= yythunkpos1503;
|
|
} if (!yy_RawNoteBlock(ctx)) goto l1502; yyDo(ctx, yy_2_Note, ctx->begin, ctx->end); goto l1501;
|
|
l1502:; ctx->pos= yypos1502; ctx->thunkpos= yythunkpos1502;
|
|
} yyDo(ctx, yy_3_Note, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Note", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 2, 0);
|
|
return 1;
|
|
l1500:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Note", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Verbatim(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "Verbatim")); if (!yy_StartList(ctx)) goto l1504; yyDo(ctx, yySet, -1, 0); if (!yy_VerbatimChunk(ctx)) goto l1504; yyDo(ctx, yy_1_Verbatim, ctx->begin, ctx->end);
|
|
l1505:;
|
|
{ int yypos1506= ctx->pos, yythunkpos1506= ctx->thunkpos; if (!yy_VerbatimChunk(ctx)) goto l1506; yyDo(ctx, yy_1_Verbatim, ctx->begin, ctx->end); goto l1505;
|
|
l1506:; ctx->pos= yypos1506; ctx->thunkpos= yythunkpos1506;
|
|
} yyDo(ctx, yy_2_Verbatim, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Verbatim", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l1504:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Verbatim", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_BlockQuote(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "BlockQuote")); if (!yy_BlockQuoteRaw(ctx)) goto l1507; yyDo(ctx, yySet, -1, 0); yyDo(ctx, yy_1_BlockQuote, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "BlockQuote", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l1507:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "BlockQuote", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_BlankLine(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "BlankLine")); if (!yy_Sp(ctx)) goto l1508; if (!yy_Newline(ctx)) goto l1508;
|
|
yyprintf((stderr, " ok %s @ %s\n", "BlankLine", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1508:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "BlankLine", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Block(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "Block"));
|
|
l1510:;
|
|
{ int yypos1511= ctx->pos, yythunkpos1511= ctx->thunkpos; if (!yy_BlankLine(ctx)) goto l1511; goto l1510;
|
|
l1511:; ctx->pos= yypos1511; ctx->thunkpos= yythunkpos1511;
|
|
}
|
|
{ int yypos1512= ctx->pos, yythunkpos1512= ctx->thunkpos; if (!yy_BlockQuote(ctx)) goto l1513; goto l1512;
|
|
l1513:; ctx->pos= yypos1512; ctx->thunkpos= yythunkpos1512; if (!yy_Verbatim(ctx)) goto l1514; goto l1512;
|
|
l1514:; ctx->pos= yypos1512; ctx->thunkpos= yythunkpos1512; if (!yy_Note(ctx)) goto l1515; goto l1512;
|
|
l1515:; ctx->pos= yypos1512; ctx->thunkpos= yythunkpos1512; if (!yy_Reference(ctx)) goto l1516; goto l1512;
|
|
l1516:; ctx->pos= yypos1512; ctx->thunkpos= yythunkpos1512; if (!yy_HorizontalRule(ctx)) goto l1517; goto l1512;
|
|
l1517:; ctx->pos= yypos1512; ctx->thunkpos= yythunkpos1512; if (!yy_Heading(ctx)) goto l1518; goto l1512;
|
|
l1518:; ctx->pos= yypos1512; ctx->thunkpos= yythunkpos1512; if (!yy_OrderedList(ctx)) goto l1519; goto l1512;
|
|
l1519:; ctx->pos= yypos1512; ctx->thunkpos= yythunkpos1512; if (!yy_BulletList(ctx)) goto l1520; goto l1512;
|
|
l1520:; ctx->pos= yypos1512; ctx->thunkpos= yythunkpos1512; if (!yy_HtmlBlock(ctx)) goto l1521; goto l1512;
|
|
l1521:; ctx->pos= yypos1512; ctx->thunkpos= yythunkpos1512; if (!yy_StyleBlock(ctx)) goto l1522; goto l1512;
|
|
l1522:; ctx->pos= yypos1512; ctx->thunkpos= yythunkpos1512; if (!yy_Para(ctx)) goto l1523; goto l1512;
|
|
l1523:; ctx->pos= yypos1512; ctx->thunkpos= yythunkpos1512; if (!yy_Plain(ctx)) goto l1509;
|
|
}
|
|
l1512:;
|
|
yyprintf((stderr, " ok %s @ %s\n", "Block", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1509:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Block", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_StartList(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "StartList"));
|
|
{ int yypos1525= ctx->pos, yythunkpos1525= ctx->thunkpos; if (!yymatchDot(ctx)) goto l1524; ctx->pos= yypos1525; ctx->thunkpos= yythunkpos1525;
|
|
} yyDo(ctx, yy_1_StartList, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "StartList", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1524:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "StartList", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_BOM(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos;
|
|
yyprintf((stderr, "%s\n", "BOM")); if (!yymatchString(ctx, "\357\273\277")) goto l1526;
|
|
yyprintf((stderr, " ok %s @ %s\n", "BOM", ctx->buf+ctx->pos));
|
|
return 1;
|
|
l1526:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "BOM", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
YY_RULE(int) yy_Doc(yycontext *ctx)
|
|
{ int yypos0= ctx->pos, yythunkpos0= ctx->thunkpos; yyDo(ctx, yyPush, 1, 0);
|
|
yyprintf((stderr, "%s\n", "Doc"));
|
|
{ int yypos1528= ctx->pos, yythunkpos1528= ctx->thunkpos; if (!yy_BOM(ctx)) goto l1528; goto l1529;
|
|
l1528:; ctx->pos= yypos1528; ctx->thunkpos= yythunkpos1528;
|
|
}
|
|
l1529:; if (!yy_StartList(ctx)) goto l1527; yyDo(ctx, yySet, -1, 0);
|
|
l1530:;
|
|
{ int yypos1531= ctx->pos, yythunkpos1531= ctx->thunkpos; if (!yy_Block(ctx)) goto l1531; yyDo(ctx, yy_1_Doc, ctx->begin, ctx->end); goto l1530;
|
|
l1531:; ctx->pos= yypos1531; ctx->thunkpos= yythunkpos1531;
|
|
} yyDo(ctx, yy_2_Doc, ctx->begin, ctx->end);
|
|
yyprintf((stderr, " ok %s @ %s\n", "Doc", ctx->buf+ctx->pos)); yyDo(ctx, yyPop, 1, 0);
|
|
return 1;
|
|
l1527:; ctx->pos= yypos0; ctx->thunkpos= yythunkpos0;
|
|
yyprintf((stderr, " fail %s @ %s\n", "Doc", ctx->buf+ctx->pos));
|
|
return 0;
|
|
}
|
|
|
|
#ifndef YY_PART
|
|
|
|
typedef int (*yyrule)(yycontext *ctx);
|
|
|
|
YY_PARSE(int) YYPARSEFROM(YY_CTX_PARAM_ yyrule yystart)
|
|
{
|
|
int yyok;
|
|
if (!yyctx->buflen)
|
|
{
|
|
yyctx->buflen= 1024;
|
|
yyctx->buf= (char *)malloc(yyctx->buflen);
|
|
yyctx->textlen= 1024;
|
|
yyctx->text= (char *)malloc(yyctx->textlen);
|
|
yyctx->thunkslen= 32;
|
|
yyctx->thunks= (yythunk *)malloc(sizeof(yythunk) * yyctx->thunkslen);
|
|
yyctx->valslen= 32;
|
|
yyctx->vals= (YYSTYPE *)malloc(sizeof(YYSTYPE) * yyctx->valslen);
|
|
yyctx->begin= yyctx->end= yyctx->pos= yyctx->limit= yyctx->thunkpos= 0;
|
|
}
|
|
yyctx->begin= yyctx->end= yyctx->pos;
|
|
yyctx->thunkpos= 0;
|
|
yyctx->val= yyctx->vals;
|
|
yyok= yystart(yyctx);
|
|
if (yyok) yyDone(yyctx);
|
|
yyCommit(yyctx);
|
|
return yyok;
|
|
}
|
|
|
|
YY_PARSE(int) YYPARSE(YY_CTX_PARAM)
|
|
{
|
|
return YYPARSEFROM(YY_CTX_ARG_ yy_Doc);
|
|
}
|
|
|
|
#endif
|
|
|
|
|
|
|
|
|