mirror of
https://github.com/RetroShare/RetroShare.git
synced 2024-10-01 02:35:48 -04:00
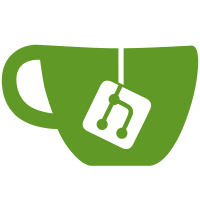
- Added error handling to xml functions - Added xslt transformation - Added retransform of existing messages - Redesigned preview dialog - Enabled embed images for forum feeds - Changed config format, switching back to an older version results in a loss of all data of the FeedReader Added new base class RSPlainTextEdit with placeholder text. New library libxslt needed git-svn-id: http://svn.code.sf.net/p/retroshare/code/trunk@6081 b45a01b8-16f6-495d-af2f-9b41ad6348cc
107 lines
3.3 KiB
C++
107 lines
3.3 KiB
C++
/****************************************************************
|
|
* RetroShare GUI is distributed under the following license:
|
|
*
|
|
* Copyright (C) 2012 by Thunder
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor,
|
|
* Boston, MA 02110-1301, USA.
|
|
****************************************************************/
|
|
|
|
#ifndef PREVIEWFEEDDIALOG_H
|
|
#define PREVIEWFEEDDIALOG_H
|
|
|
|
#include <QDialog>
|
|
#include <QItemDelegate>
|
|
|
|
#include "interface/rsFeedReader.h"
|
|
|
|
namespace Ui {
|
|
class PreviewFeedDialog;
|
|
}
|
|
|
|
class QTreeWidget;
|
|
class RsFeedReader;
|
|
class FeedReaderNotify;
|
|
class FeedInfo;
|
|
|
|
// not yet functional
|
|
//class PreviewItemDelegate : public QItemDelegate
|
|
//{
|
|
// Q_OBJECT
|
|
|
|
//public:
|
|
// PreviewItemDelegate(QTreeWidget *parent);
|
|
|
|
//private slots:
|
|
// void sectionResized(int logicalIndex, int oldSize, int newSize);
|
|
|
|
//protected:
|
|
// virtual void drawDisplay(QPainter *painter, const QStyleOptionViewItem &option, const QRect &rect, const QString &text) const;
|
|
// virtual void drawFocus(QPainter *painter, const QStyleOptionViewItem &option, const QRect &rect) const;
|
|
// virtual QSize sizeHint(const QStyleOptionViewItem &option, const QModelIndex &index) const;
|
|
//};
|
|
|
|
class PreviewFeedDialog : public QDialog
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
PreviewFeedDialog(RsFeedReader *feedReader, FeedReaderNotify *notify, const FeedInfo &feedInfo, QWidget *parent = 0);
|
|
~PreviewFeedDialog();
|
|
|
|
RsFeedTransformationType getData(std::list<std::string> &xpathsToUse, std::list<std::string> &xpathsToRemove, std::string &xslt);
|
|
|
|
protected:
|
|
bool eventFilter(QObject *obj, QEvent *ev);
|
|
|
|
private slots:
|
|
void previousMsg();
|
|
void nextMsg();
|
|
void showStructureFrame();
|
|
void xpathListCustomPopupMenu(QPoint point);
|
|
void xpathCloseEditor(QWidget *editor, QAbstractItemDelegate::EndEditHint hint);
|
|
void addXPath();
|
|
void editXPath();
|
|
void removeXPath();
|
|
void transformationTypeChanged();
|
|
|
|
/* FeedReaderNotify */
|
|
void feedChanged(const QString &feedId, int type);
|
|
void msgChanged(const QString &feedId, const QString &msgId, int type);
|
|
|
|
private:
|
|
void processSettings(bool load);
|
|
int getMsgPos();
|
|
void setFeedInfo(const QString &info);
|
|
void setTransformationInfo(const QString &info);
|
|
void fillFeedInfo(const FeedInfo &feedInfo);
|
|
void updateMsgCount();
|
|
void updateMsg();
|
|
void fillStructureTree(bool transform);
|
|
void processTransformation();
|
|
|
|
RsFeedReader *mFeedReader;
|
|
FeedReaderNotify *mNotify;
|
|
std::string mFeedId;
|
|
std::string mMsgId;
|
|
std::list<std::string> mMsgIds;
|
|
std::string mDescription;
|
|
std::string mDescriptionTransformed;
|
|
|
|
Ui::PreviewFeedDialog *ui;
|
|
};
|
|
|
|
#endif // PREVIEWFEEDDIALOG_H
|