mirror of
https://github.com/RetroShare/RetroShare.git
synced 2024-10-01 02:35:48 -04:00
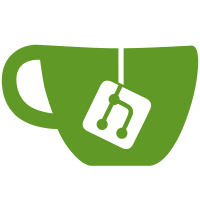
- written test for new forumreadstatus item, and other recent changes to distrib items added graphics support to blogs - can now have pics for html blogs! but removed attachment, a channels artifact added copy constructor to rstlvimage, needed for tests to work git-svn-id: http://svn.code.sf.net/p/retroshare/code/trunk@3317 b45a01b8-16f6-495d-af2f-9b41ad6348cc
125 lines
3.4 KiB
C++
125 lines
3.4 KiB
C++
#ifndef RS_FORUM_ITEMS_H
|
|
#define RS_FORUM_ITEMS_H
|
|
|
|
/*
|
|
* libretroshare/src/serialiser: rsforumitems.h
|
|
*
|
|
* RetroShare Serialiser.
|
|
*
|
|
* Copyright 2007-2008 by Robert Fernie.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Library General Public
|
|
* License Version 2 as published by the Free Software Foundation.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Library General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Library General Public
|
|
* License along with this library; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
|
|
* USA.
|
|
*
|
|
* Please report all bugs and problems to "retroshare@lunamutt.com".
|
|
*
|
|
*/
|
|
|
|
#include <map>
|
|
|
|
#include "serialiser/rsserviceids.h"
|
|
#include "serialiser/rsserial.h"
|
|
#include "serialiser/rstlvtypes.h"
|
|
#include "serialiser/rstlvkeys.h"
|
|
|
|
#include "serialiser/rsdistribitems.h"
|
|
|
|
const uint8_t RS_PKT_SUBTYPE_FORUM_GRP = 0x01;
|
|
const uint8_t RS_PKT_SUBTYPE_FORUM_MSG = 0x02;
|
|
const uint8_t RS_PKT_SUBTYPE_FORUM_READ_STATUS = 0x03;
|
|
|
|
/**************************************************************************/
|
|
|
|
class RsForumMsg: public RsDistribMsg
|
|
{
|
|
public:
|
|
RsForumMsg()
|
|
:RsDistribMsg(RS_SERVICE_TYPE_FORUM, RS_PKT_SUBTYPE_FORUM_MSG) { return; }
|
|
virtual ~RsForumMsg() { return; }
|
|
|
|
virtual void clear();
|
|
virtual std::ostream& print(std::ostream &out, uint16_t indent = 0);
|
|
|
|
/*
|
|
* RsDistribMsg has:
|
|
* grpId, parentId, threadId & timestamp.
|
|
*/
|
|
|
|
std::string srcId;
|
|
|
|
std::wstring title;
|
|
std::wstring msg;
|
|
|
|
};
|
|
|
|
/*!
|
|
* This is used to keep track of whether a message has been read
|
|
* by client
|
|
*/
|
|
class RsForumReadStatus : public RsDistribChildConfig
|
|
{
|
|
public:
|
|
RsForumReadStatus()
|
|
: RsDistribChildConfig(RS_SERVICE_TYPE_FORUM, RS_PKT_SUBTYPE_FORUM_READ_STATUS)
|
|
{ return; }
|
|
|
|
virtual ~RsForumReadStatus() {return; }
|
|
|
|
virtual void clear();
|
|
virtual std::ostream& print(std::ostream &out, uint16_t indent);
|
|
|
|
std::string forumId;
|
|
|
|
/// a map which contains the read for messages within a forum
|
|
std::map<std::string, uint32_t> msgReadStatus;
|
|
|
|
};
|
|
|
|
class RsForumSerialiser: public RsSerialType
|
|
{
|
|
public:
|
|
RsForumSerialiser()
|
|
:RsSerialType(RS_PKT_VERSION_SERVICE, RS_SERVICE_TYPE_FORUM)
|
|
{ return; }
|
|
virtual ~RsForumSerialiser()
|
|
{ return; }
|
|
|
|
virtual uint32_t size(RsItem *);
|
|
virtual bool serialise (RsItem *item, void *data, uint32_t *size);
|
|
virtual RsItem * deserialise(void *data, uint32_t *size);
|
|
|
|
private:
|
|
|
|
/* For RS_PKT_SUBTYPE_FORUM_GRP */
|
|
//virtual uint32_t sizeGrp(RsForumGrp *);
|
|
//virtual bool serialiseGrp (RsForumGrp *item, void *data, uint32_t *size);
|
|
//virtual RsForumGrp *deserialiseGrp(void *data, uint32_t *size);
|
|
|
|
/* For RS_PKT_SUBTYPE_FORUM_MSG */
|
|
virtual uint32_t sizeMsg(RsForumMsg *);
|
|
virtual bool serialiseMsg(RsForumMsg *item, void *data, uint32_t *size);
|
|
virtual RsForumMsg *deserialiseMsg(void *data, uint32_t *size);
|
|
|
|
virtual uint32_t sizeReadStatus(RsForumReadStatus* );
|
|
virtual bool serialiseReadStatus(RsForumReadStatus* item, void* data, uint32_t *size);
|
|
virtual RsForumReadStatus *deserialiseReadStatus(void* data, uint32_t *size);
|
|
|
|
};
|
|
|
|
/**************************************************************************/
|
|
|
|
#endif /* RS_FORUM_ITEMS_H */
|
|
|
|
|