mirror of
https://github.com/RetroShare/RetroShare.git
synced 2024-12-28 08:59:37 -05:00
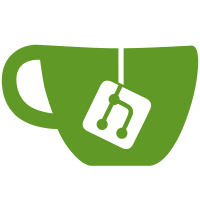
Deprecate id field in JSON API as it may cause problems in Qml Offer gxs_id field in JSON API as an id alternative LibresapiLocalClient support callbacks now an instance may be shared for different tasks Expose an instance of LibresapiLocalClient to Qml, type exposure is kept for retrocompatibility but deprecated Qml app now has a tab that permit to exchange some message with selected distant peer
58 lines
1.7 KiB
C++
58 lines
1.7 KiB
C++
/*
|
|
* libresapi local socket client
|
|
* Copyright (C) 2016 Gioacchino Mazzurco <gio@eigenlab.org>
|
|
* Copyright (C) 2016 Manu Pineda <manu@cooperativa.cat>
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU Affero General Public License as
|
|
* published by the Free Software Foundation, either version 3 of the
|
|
* License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU Affero General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Affero General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef LIBRESAPILOCALCLIENT_H
|
|
#define LIBRESAPILOCALCLIENT_H
|
|
|
|
#include <QLocalSocket>
|
|
#include <QQueue>
|
|
#include <QJSValue>
|
|
|
|
class LibresapiLocalClient : public QObject
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
LibresapiLocalClient() : mLocalSocket(this) {}
|
|
|
|
Q_INVOKABLE int request( const QString& path, const QString& jsonData = "",
|
|
QJSValue callback = QJSValue::NullValue);
|
|
Q_INVOKABLE void openConnection(QString socketPath);
|
|
|
|
private:
|
|
QLocalSocket mLocalSocket;
|
|
QQueue<QJSValue> callbackQueue;
|
|
|
|
private slots:
|
|
void socketError(QLocalSocket::LocalSocketError error);
|
|
void read();
|
|
|
|
signals:
|
|
/// @deprecated @see LibresapiLocalClient::responseReceived instead
|
|
void goodResponseReceived(const QString & msg);
|
|
|
|
/**
|
|
* @brief responseReceived emitted when a response is received
|
|
* @param msg
|
|
*/
|
|
void responseReceived(const QString & msg);
|
|
};
|
|
|
|
#endif // LIBRESAPILOCALCLIENT_H
|