mirror of
https://github.com/RetroShare/RetroShare.git
synced 2024-12-28 08:59:37 -05:00
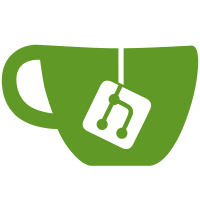
In preparation for making RS support IPv6. NB: This breaks the build of retroshare-gui, as the sockaddr_storage_xxx fns are only defined as prototypes for now. All the aux libraries like udp / stun / tcponudp / dht have still to be converted. These changes will probably break various things and need to be tested thoroughly. git-svn-id: http://svn.code.sf.net/p/retroshare/code/branches/v0.6-initdev@6735 b45a01b8-16f6-495d-af2f-9b41ad6348cc
114 lines
3.0 KiB
C++
114 lines
3.0 KiB
C++
/*
|
|
* libretroshare/src/zeroconf: p3zcnatassist.h
|
|
*
|
|
* ZeroConf interface for RetroShare.
|
|
*
|
|
* Copyright 2011-2012 by Robert Fernie.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Library General Public
|
|
* License Version 2 as published by the Free Software Foundation.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Library General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Library General Public
|
|
* License along with this library; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
|
|
* USA.
|
|
*
|
|
* Please report all bugs and problems to "retroshare@lunamutt.com".
|
|
*
|
|
*/
|
|
|
|
|
|
#ifndef MRK_P3_ZC_NAT_ASSIST_H
|
|
#define MRK_P3_ZC_NAT_ASSIST_H
|
|
|
|
#include "util/rswin.h"
|
|
|
|
#include "pqi/pqiassist.h"
|
|
#include "retroshare/rsdht.h"
|
|
|
|
#include <string>
|
|
#include <map>
|
|
#include "pqi/pqinetwork.h"
|
|
#include "pqi/pqimonitor.h"
|
|
#include "pqi/p3peermgr.h"
|
|
#include "util/rsthreads.h"
|
|
|
|
#include <dns_sd.h>
|
|
|
|
|
|
class p3NetMgr;
|
|
|
|
class p3zcNatAssist: public pqiNetAssistFirewall
|
|
{
|
|
public:
|
|
p3zcNatAssist();
|
|
virtual ~p3zcNatAssist();
|
|
|
|
/* pqiNetAssist - external interface functions */
|
|
virtual int tick();
|
|
virtual void enable(bool on);
|
|
virtual void shutdown(); /* blocking call */
|
|
virtual void restart();
|
|
|
|
virtual bool getEnabled();
|
|
virtual bool getActive();
|
|
|
|
virtual void setInternalPort(unsigned short iport_in);
|
|
virtual void setExternalPort(unsigned short eport_in);
|
|
virtual bool getInternalAddress(struct sockaddr_storage &addr);
|
|
virtual bool getExternalAddress(struct sockaddr_storage &addr);
|
|
|
|
/* TO IMPLEMENT: New Port Forward interface to support as many ports as necessary */
|
|
virtual bool requestPortForward(const PortForwardParams ¶ms) { return false; }
|
|
virtual bool statusPortForward(const uint32_t fwdId, PortForwardParams ¶ms) { return false; }
|
|
|
|
/* pqiNetAssistConnect - external interface functions */
|
|
|
|
|
|
public:
|
|
|
|
// Callbacks must be public -> so they can be accessed.
|
|
void callbackMapping(DNSServiceRef sdRef, DNSServiceFlags flags,
|
|
uint32_t interfaceIndex, DNSServiceErrorType errorCode,
|
|
uint32_t externalAddress, DNSServiceProtocol protocol,
|
|
uint16_t internalPort, uint16_t externalPort, uint32_t ttl);
|
|
|
|
private:
|
|
|
|
/* monitoring fns */
|
|
void checkServiceFDs();
|
|
void locked_checkFD(DNSServiceRef ref);
|
|
|
|
int locked_startMapping();
|
|
void locked_stopMapping();
|
|
|
|
/**************** DATA ****************/
|
|
|
|
RsMutex mZcMtx;
|
|
|
|
bool mEnabled;
|
|
bool mMapped;
|
|
|
|
uint16_t mLocalPort;
|
|
bool mLocalPortSet;
|
|
|
|
uint16_t mExternalPort;
|
|
bool mExternalPortSet;
|
|
|
|
struct sockaddr_storage mExternalAddress;
|
|
int mTTL;
|
|
|
|
DNSServiceRef mMappingRef;
|
|
uint32_t mMappingStatus;
|
|
time_t mMappingStatusTS;
|
|
};
|
|
|
|
#endif /* MRK_P3_ZC_NAT_ASSIST_H */
|
|
|