mirror of
https://github.com/ipfs/awesome-ipfs.git
synced 2024-07-04 18:41:20 +00:00
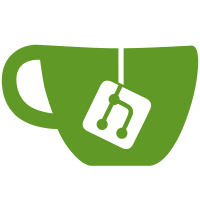
Not quite happy with the layout of these dataset items as they become to wide with the hash in the description area. Also the README.md seems to have changed order but changes were never commited. Also includes a fix to be able to run dev server on any port with the `PORT` environment variable. License: MIT Signed-off-by: Victor Bjelkholm <git@victor.earth>
73 lines
1.9 KiB
JavaScript
73 lines
1.9 KiB
JavaScript
const chokidar = require('chokidar')
|
|
const path = require('path')
|
|
const runAll = require('npm-run-all')
|
|
const dataFolder = path.join(__dirname, '../data')
|
|
const srcFolder = path.join(__dirname, '../src')
|
|
const cssPath = path.join(__dirname, '../src/css')
|
|
const jsPath = path.join(__dirname, '../src/js')
|
|
const http = require('http')
|
|
|
|
const options = {
|
|
stdout: process.stdout,
|
|
stderr: process.stderr
|
|
}
|
|
|
|
const runHugo = () => {
|
|
return runAll(['build:hugo'], options).catch(() => {})
|
|
}
|
|
|
|
const handler = (path) => {
|
|
if (path.startsWith(dataFolder)) {
|
|
runAll(['build:data'], options).then(runHugo)
|
|
} else if (path.startsWith(cssPath)) {
|
|
runAll(['build:css'], options).then(runHugo)
|
|
} else if (path.startsWith(jsPath)) {
|
|
runAll(['build:js'], options).then(runHugo)
|
|
} else {
|
|
runHugo()
|
|
}
|
|
}
|
|
|
|
async function run () {
|
|
console.log('Preparing fonts, css, js and data...')
|
|
await runAll(['build:fonts', 'build:css', 'build:js', 'build:icons', 'build:data'], {
|
|
stdout: process.stdout,
|
|
stderr: process.stderr,
|
|
parallel: true
|
|
})
|
|
|
|
await runHugo()
|
|
|
|
console.log('Starting server...')
|
|
|
|
const ecstatic = require('ecstatic')({
|
|
root: `${__dirname}/../public`,
|
|
showDir: true,
|
|
autoIndex: true
|
|
})
|
|
|
|
const port = process.env.PORT || 8080
|
|
http.createServer(ecstatic).listen(port)
|
|
|
|
const watcher = chokidar.watch([dataFolder, srcFolder], {
|
|
ignored: (string) => string.indexOf('src/content') !== -1 ||
|
|
string.indexOf('src/data') !== -1 ||
|
|
string.indexOf('src/resources') !== -1 ||
|
|
string.indexOf('src/layouts/partials/indexes') !== -1 ||
|
|
string.indexOf('src/static/fonts') !== -1 ||
|
|
string.indexOf('src/static/app.css') !== -1 ||
|
|
string.indexOf('src/static/app.js') !== -1,
|
|
persistent: true,
|
|
ignoreInitial: true,
|
|
awaitWriteFinish: true
|
|
})
|
|
|
|
watcher
|
|
.on('ready', () => console.log('Listening on :' + port))
|
|
.on('add', handler)
|
|
.on('change', handler)
|
|
.on('unlink', handler)
|
|
}
|
|
|
|
run()
|